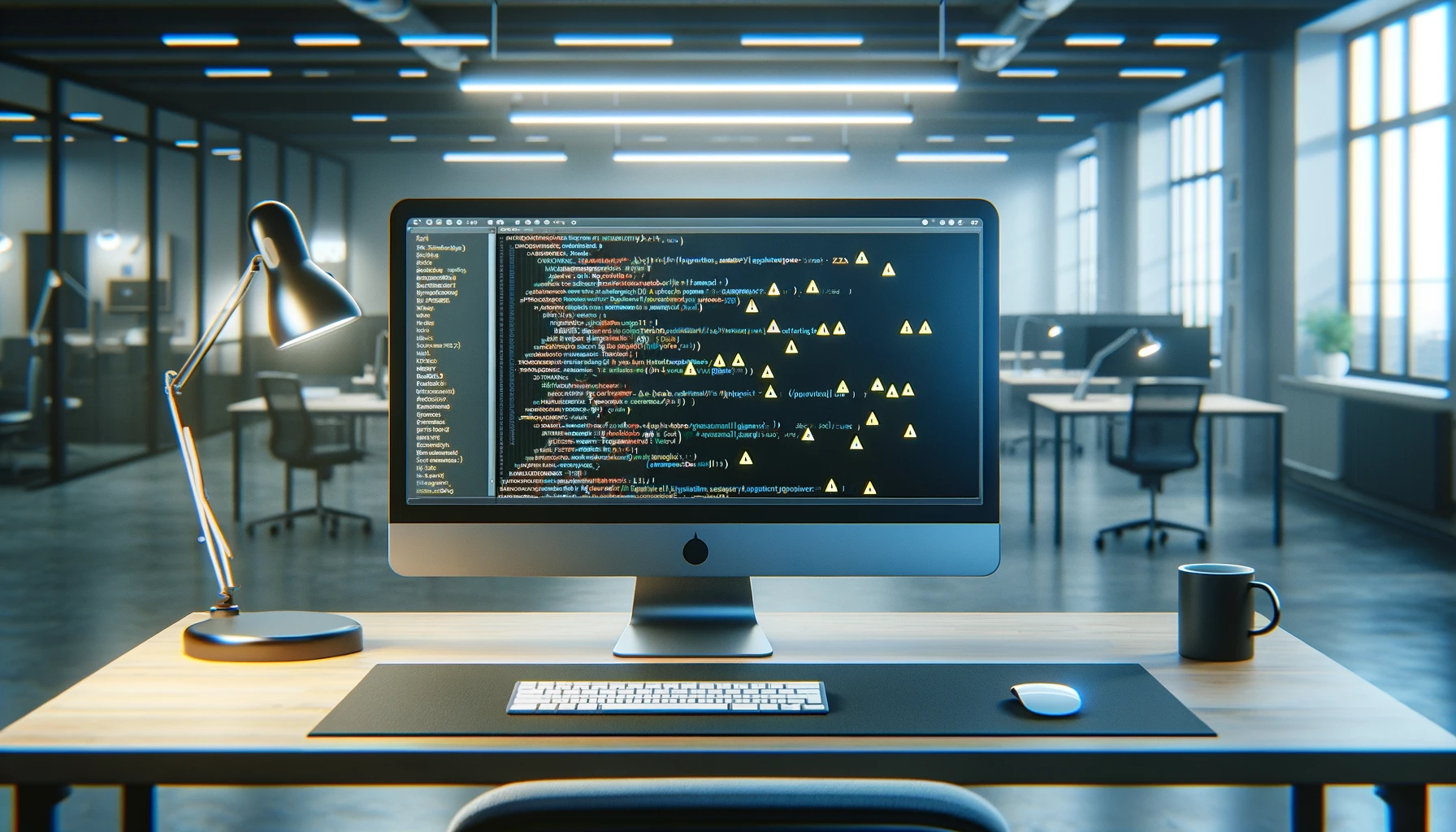
To treat warnings as errors in an Angular project, ensuring that warnings are not ignored and are addressed promptly, you can modify the Angular build configurations. Specifically, this involves changes in the TypeScript and Angular CLI configurations. Here’s how you can do it:
For TypeScript Warnings
To treat TypeScript warnings as errors, you can modify the tsconfig.json file in your project. TypeScript doesn’t directly support treating warnings as errors, but you can enforce stricter type checks and other options that will result in errors instead of warnings. Add or modify the following options in your tsconfig.json:
{ "compilerOptions": { "strict": true, // Enable all strict type-checking options. "noImplicitReturns": true, // Report error when not all code paths in function return a value. "noFallthroughCasesInSwitch": true, // Report errors for fallthrough cases in switch statement. // Add any other options you find necessary for your project } }
For Angular CLI Warnings
Angular CLI uses Webpack and Terser for its build process. As of my last update, Angular CLI does not provide a direct option to treat warnings as errors. However, you can customize the Angular build process by using custom Webpack configuration via third-party tools like @angular-builders/custom-webpack
.
Install Custom Webpack Builder:
First, you need to install the custom Webpack package.
npm install @angular-builders/custom-webpack --save-dev
Modify angular.json to Use the Custom Builder
In your angular.json, replace the default builder for your project with the custom Webpack builder. For example, change the builder from @angular-devkit/build-angular:browser
to @angular-builders/custom-webpack:browser
for the build target.
Create a Custom Webpack Configuration File:
Create a custom Webpack configuration file in your project (e.g., custom-webpack.config.js). In this file, you can use the Webpack’s stats option to treat warnings as errors.
module.exports = { stats: { warnings: true, warningsFilter: () => false, // Treat all warnings as errors by not filtering them out }, };
Reference Your Custom Webpack Config in angular.json
In your angular.json, under the configurations for the custom Webpack builder, add a reference to your custom Webpack configuration file.
"options": { "customWebpackConfig": { "path": "./custom-webpack.config.js" } }
Please note, these methods enforce stricter rules and configurations to minimize warnings or treat them as errors. However, due to the nature of JavaScript and TypeScript, not all warnings can be converted to errors without potentially introducing build process customizations that might be hard to maintain. Always test your configurations thoroughly to ensure they work as expected in your development and production builds.
Previous
How to use custom name except for _count in prisma?
Next