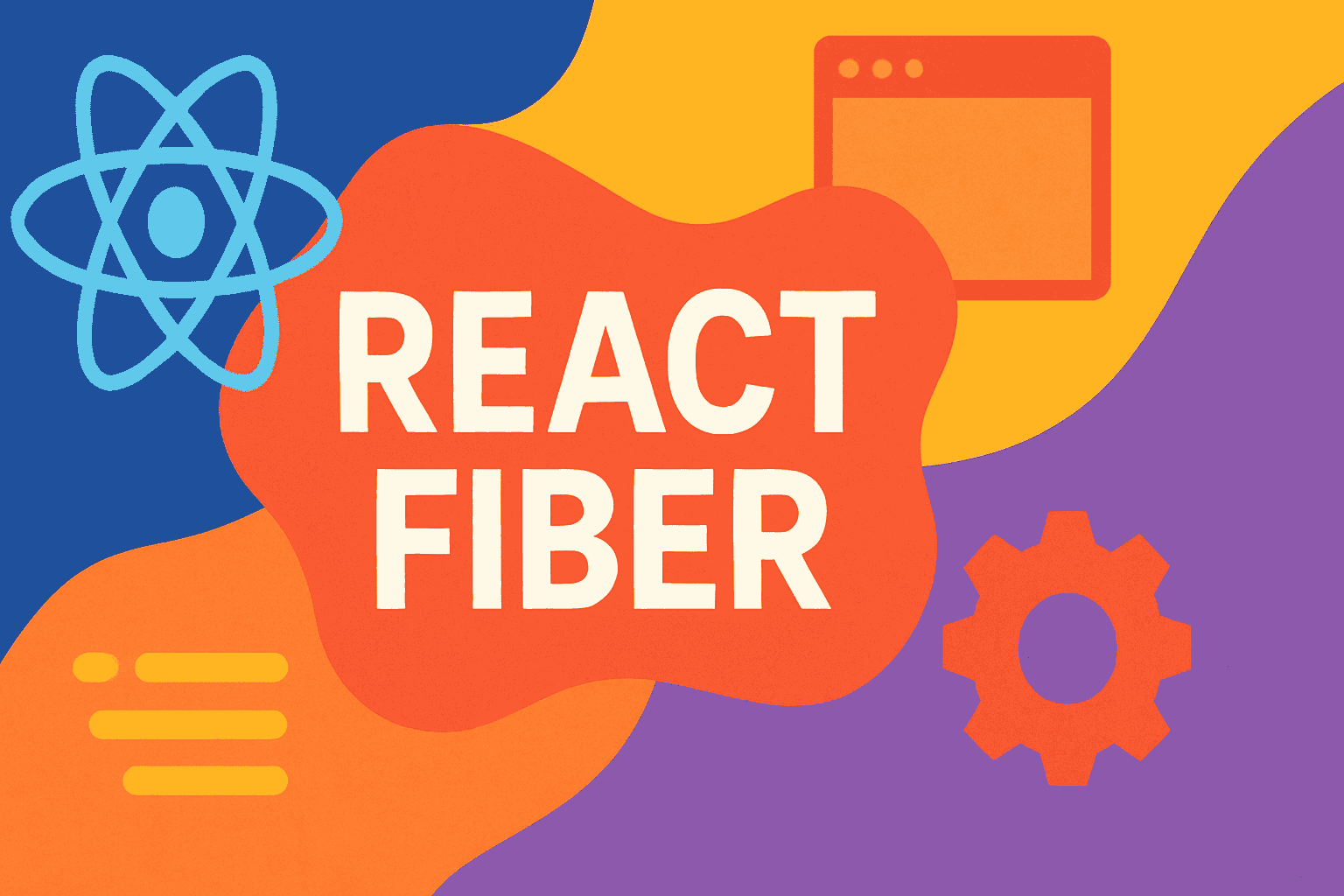
What is React Fiber?
React Fiber is the latest iteration of React’s core algorithm. It redefines how React handles rendering and updates. As developers, we know that performance and responsiveness are crucial for user experience. React Fiber addresses these needs by optimizing the rendering process.
At its core, React Fiber is a reimplementation of the reconciliation algorithm. It’s designed to enable incremental rendering, which breaks rendering work into small chunks. This allows React to pause and prioritize rendering tasks, ensuring smoother updates and animations.
One of the standout features of React Fiber is its ability to manage asynchronous rendering. This means your app remains responsive even when performing heavy computations. Fiber’s architecture allows it to pause work and resume it later, making it ideal for complex applications.
React Fiber also introduces new concepts like fibers, lanes, and priorities. These play a crucial role in scheduling updates and rendering. By assigning different priorities to updates, Fiber ensures that critical updates are handled promptly, improving the app’s overall performance.
Understanding React Fiber is essential for developers looking to build performant React applications. It offers a more flexible and efficient approach to rendering. This makes it easier to create applications that provide an excellent user experience without compromising on performance.
Core Concepts of React Fiber
React Fiber is an internal engine that redefines how React manages rendering. It focuses on incremental rendering, allowing React to pause and resume work efficiently. This approach enhances the rendering process, making it more adaptable to resource constraints and improving user experience.
One key concept of React Fiber is its ability to prioritize tasks. Fiber excels in handling updates by categorizing them based on priority. This ensures that high-priority updates like animations or user input are processed faster, while less critical tasks are deferred.
React Fiber also introduces the concept of “scheduling.” It breaks down rendering work into units called “fibers,” which are managed in a work loop. This loop enables React to pause work, handle higher-priority tasks, and resume work later without starting over.
Here’s a simple example of how Fiber might manage rendering:
// Prioritizing updates const rootNode = document.getElementById('root'); ReactDOM.createRoot(rootNode).render();
React Fiber also supports concurrency. This means multiple tasks can be processed at once, improving performance. The concurrent mode allows React to work on multiple updates without blocking the main thread, resulting in smoother applications.
Moreover, Fiber’s architecture supports better error boundaries. This enhances debugging by providing more detailed error messages and stack traces. Developers can quickly identify issues and improve their applications.
In summary, React Fiber is a significant evolution in React’s rendering process. By leveraging prioritization, scheduling, and concurrency, it provides a robust framework for building responsive and efficient web applications.
How React Fiber Improves Performance
React Fiber introduces a new reconciliation algorithm that enhances rendering efficiency. The key improvement lies in its ability to break rendering work into units. This allows React to pause, prioritize, and reuse tasks, leading to smoother user experiences.
In traditional React, rendering was a blocking process. However, with React Fiber, rendering is non-blocking. This means the browser can work on high-priority tasks first. For example, animations and transitions can run smoothly even when the application is processing large updates.
React Fiber achieves this by using a concept called “Time Slicing”. It allows React to split rendering work into chunks and spread the work over multiple frames. As a result, React can make quicker updates without freezing the UI.
With Time Slicing, developers can create highly interactive applications without worrying about performance bottlenecks. Fiber’s asynchronous rendering ensures that apps remain responsive under heavy computational loads.
Furthermore, React Fiber supports concurrent rendering. This feature enables multiple tasks to run simultaneously, optimizing the application’s responsiveness. As a developer, you can design applications that feel faster and more fluid.
Here is a simple example of a React component taking advantage of Fiber’s capabilities:
function MyComponent() { const [count, setCount] = React.useState(0); return (); }Current Count: {count}
- React Fiber allows more granular updates.
- It improves UI responsiveness and animation smoothness.
- Time Slicing distributes rendering across frames.
- Concurrent rendering boosts task handling efficiency.
Concurrency and Scheduling in React Fiber
In the ever-evolving world of web development, React Fiber stands out as a game-changer.
One of its most noteworthy features is its ability to handle concurrency and scheduling effectively.
This ensures that your applications remain smooth and responsive, even under heavy workloads.
React Fiber introduces a more granular approach to rendering.
It breaks down the rendering work into units of work called “fibers.”
This enables React to pause and resume work, making it possible to prioritize more critical updates.
Imagine your application is a busy restaurant.
Without React Fiber, your app is like a chef who cooks one dish at a time, ignoring urgent orders.
With Fiber, the chef can switch between tasks, ensuring everyone gets fed promptly.
The secret sauce behind this lies in the concept of “time slicing.”
It allows React to divide rendering work into small chunks and spread them over multiple frames.
This way, the browser remains responsive, and user interactions feel instantaneous.
Here’s a simple example to illustrate how React Fiber’s concurrency model works:
const root = ReactDOM.createRoot(document.getElementById('root')); root.render();
In the above code, ReactDOM.createRoot
is the entry point to Fiber’s new concurrent mode.
This allows the rendering process to be more flexible and interruptible, enhancing user experience.
React Fiber also introduces a cooperative scheduling model.
In this model, React can yield control to the browser between rendering tasks.
This way, essential tasks like animations and input events are not blocked.
To sum up, React Fiber’s concurrency and scheduling capabilities make it possible to build fluid, performant applications.
By understanding these concepts, developers can leverage Fiber to create more responsive UIs.
Comparison: React Fiber vs. React DOM
React Fiber and React DOM are essential components in the React ecosystem. Understanding their differences is crucial for developers aiming to optimize performance in their applications.
React Fiber is a complete rewrite of the React core algorithm. Its main goal is to enable incremental rendering of the virtual DOM. This approach allows React to prioritize and pause work, making the UI smoother and more responsive.
On the other hand, React DOM is responsible for updating the actual DOM in the browser. It takes care of rendering the components and is the bridge between React components and the DOM itself.
Let’s delve deeper into the technical differences:
- Concurrency: React Fiber introduces concurrency, allowing React to handle updates more efficiently. React DOM, however, doesn’t manage concurrency on its own.
- Re-rendering: React Fiber can pause and resume work, while React DOM executes tasks synchronously.
- Optimization: Fiber allows React to prioritize updates, improving the user experience. React DOM relies on the browser’s native rendering capabilities.
Consider this code example, where React Fiber’s benefits are evident:
const List = ({ items }) => ({items.map(item => ();{item.name}))}
In this scenario, React Fiber optimizes the rendering process by scheduling work based on priority. React DOM will then update the actual DOM efficiently.
Migrating to React Fiber: Best Practices
React Fiber is the latest reconciliation algorithm in React 16+. It provides smoother and more reliable rendering. Migrating to React Fiber can seem daunting, but understanding best practices makes it easier.
First, ensure your code is free of deprecated methods. React Fiber introduces new lifecycle methods. Replace deprecated ones like componentWillMount
and componentWillReceiveProps
with alternatives like componentDidMount
and getDerivedStateFromProps
.
Next, test your application thoroughly. React Fiber improves rendering performance by breaking tasks into units of work. However, it may reveal hidden bugs. Use tools like Jest and React Testing Library to ensure stability.
Additionally, optimize your components for concurrent rendering. React Fiber supports asynchronous rendering, allowing tasks to pause and resume, improving responsiveness. Use React.Suspense
and React.lazy
to load components dynamically.
Here’s a simple code example to illustrate:
import React, { Suspense, lazy } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); function App() { return (}>Loading... ); }
Lastly, keep your dependencies updated. React Fiber and its new features are best utilized with the latest versions of libraries. Regular updates ensure compatibility and leverage improvements.
By following these best practices, you can migrate to React Fiber smoothly. This will enhance your application’s performance and maintainability.
Conclusion: The Future of React with Fiber
React Fiber has revolutionized the way developers build and optimize web applications. As the architecture evolves, its impact will continue to grow. Fiber’s ability to prioritize tasks enhances performance, making applications faster and more responsive.
Looking ahead, we can expect React Fiber to incorporate even more features that simplify development. Its concurrent rendering capabilities allow developers to create smoother user experiences. This is crucial in today’s fast-paced digital landscape.
Moreover, React Fiber’s adaptability ensures it will remain relevant as new technologies emerge. The React community is vibrant and constantly innovating, which means Fiber will benefit from continuous improvements. Developers can anticipate more tools and libraries to integrate with React Fiber, expanding its ecosystem.
As Google’s algorithm increasingly values user experience, React Fiber’s optimizations align perfectly with these requirements. Faster load times and seamless interactions contribute to higher search rankings. This makes React Fiber a strategic choice for developers focusing on SEO.
In conclusion, React Fiber is not just a current trend but a future-proof solution. Its continuous evolution promises to meet the demands of modern web development. Developers should embrace React Fiber to stay ahead in the ever-changing landscape of web technologies.
Previous