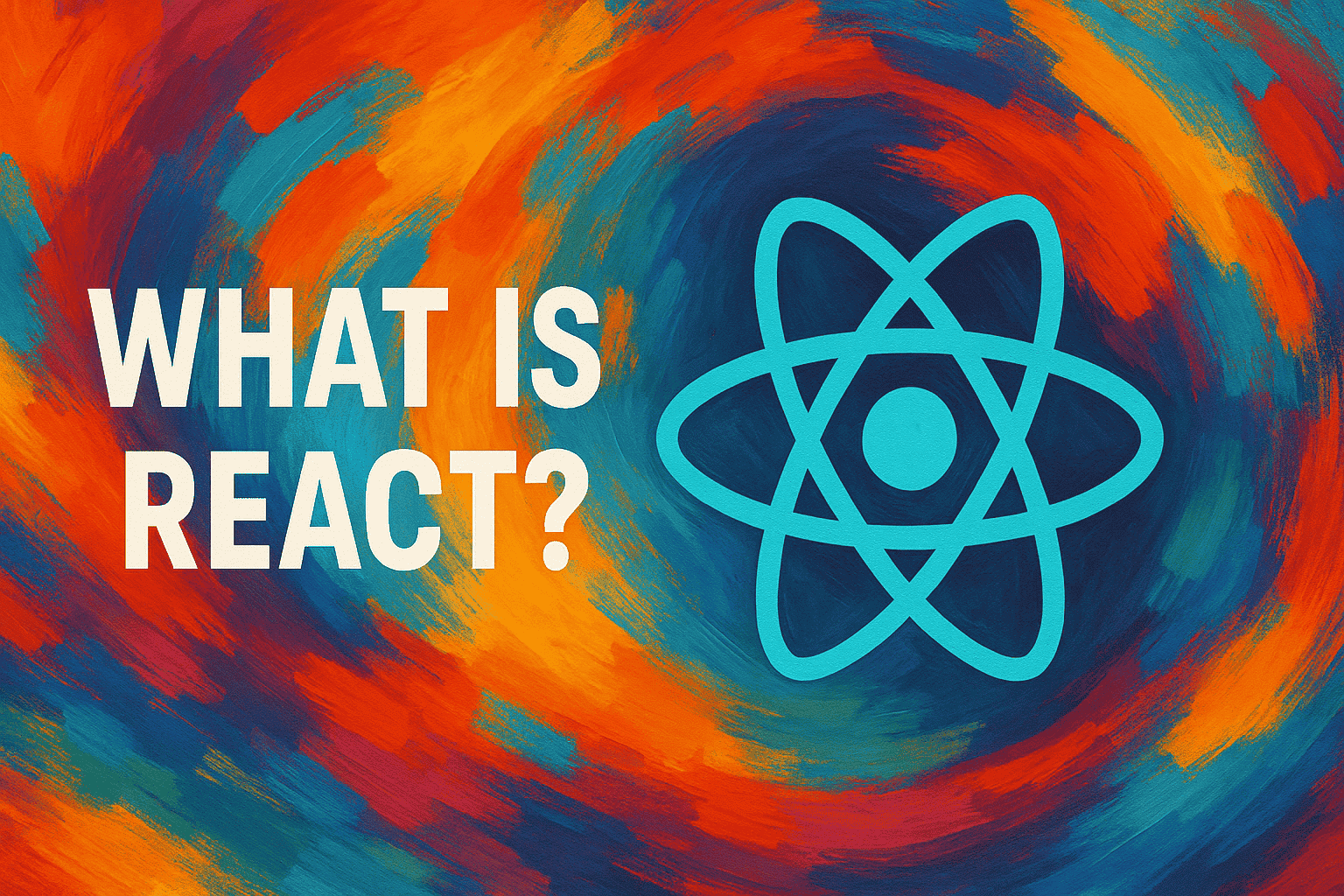
What is React?
React is a powerful JavaScript library for building user interfaces. It simplifies the process of creating interactive and dynamic web applications. Developed by Facebook, React focuses on the view layer of an application, making it easier to build scalable and efficient UIs.
At its core, React allows developers to create reusable UI components. These components can manage their own state and are responsible for rendering a part of the user interface. By breaking down the UI into smaller pieces, React promotes code reusability and maintainability.
One of the key features of React is the virtual DOM. Instead of updating the real DOM directly, React creates a virtual representation. This approach enhances performance by minimizing expensive DOM operations. When the state of a component changes, React efficiently updates the virtual DOM and reconciles it with the real DOM.
React’s component-based architecture allows for seamless integration with other libraries or frameworks. It can be used in combination with tools like Redux for state management or with libraries like React Router for handling navigation. This flexibility makes React a popular choice among developers for building modern web applications.
With a strong community and extensive ecosystem, React continues to evolve. Developers benefit from a rich set of tools and libraries that extend React’s functionality. Whether you’re building a small personal project or a large-scale application, React provides the tools to create a responsive and engaging user experience.
History and Evolution of React
React, a revolutionary JavaScript library, was created by Facebook in 2011. It was first deployed on Facebook’s News Feed. The aim was to improve the user experience by making web pages more interactive and dynamic.
In 2013, React was open-sourced, which allowed developers worldwide to contribute. This move sparked widespread adoption and innovation, making React a popular choice for building user interfaces.
React’s introduction of the Virtual DOM changed the way developers approached UI development. It optimized updates, resulting in faster rendering times. This was a significant improvement over traditional methods.
As React evolved, new features like Hooks were added in 2018. Hooks allowed developers to use state and other React features without writing a class. This made components more straightforward and functional.
Over the years, React’s ecosystem expanded with a variety of tools and libraries. These include Redux for state management and React Router for navigation. They enhanced the capabilities of React applications.
React’s consistent updates and a robust community ensure it remains a leading choice. It continues to adapt to developers’ needs, maintaining its relevance in the ever-changing tech landscape.
Component-Based Architecture
Component-based architecture is a cornerstone of React that revolutionizes how developers build web applications. This approach breaks down a UI into independent, reusable pieces, known as components.
Each component in React is like a Lego block. By combining these blocks, developers can create complex interfaces effortlessly. This modular design makes the codebase more manageable and easier to debug.
One of the significant advantages of component-based architecture is reusability. Developers can create a library of components and use them across different parts of the application. This reduces redundancy and speeds up the development process.
Components in React can also have their own state and lifecycle methods. This feature allows developers to manage the dynamic aspects of their UI efficiently. The encapsulated nature of components ensures that changes in one part do not affect others, enhancing maintainability.
- Reusability of components
- Improved code maintainability
- Independent management of UI parts
- Faster development process
Another benefit of using component-based architecture in React is the ease of testing. Each component can be tested in isolation, leading to more reliable applications. This is crucial for maintaining high standards in software quality.
In summary, component-based architecture in React empowers developers to build scalable, maintainable, and efficient applications. It encourages a modular approach that aligns well with modern development practices.
State and Props in React
React is a powerful JavaScript library for building user interfaces. One of its core concepts is the use of “State” and “Props”. Understanding these concepts is crucial for developers working with React.
In React, “State” refers to the mutable data of a component. It’s like the heart of the component. It can change over time and affects how the component renders. You can think of it as the component’s personal data storage.
Here’s a simple example of how to use state in a React component:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return (); }Count: {count}
On the other hand, “Props” are the way components communicate with each other. They are read-only and help pass data from a parent component to a child component. Props make components reusable and customizable.
Check out this example of how props are used:
function Greeting(props) { returnHello, {props.name}!
; } function App() { return; }
In this example, the Greeting
component receives the name
prop and uses it to display a personalized message. By combining state and props, React components become dynamic and interactive, opening endless possibilities for developers.
React Hooks: A New Way to Handle State
React Hooks offer a revolutionary approach to managing state in functional components. Introduced in React 16.8, Hooks provide developers with a more intuitive way to handle state and lifecycle methods in React applications.
Before Hooks, state management in functional components was a challenge. Developers often had to rely on class components. Now, with Hooks, you can access state and other React features directly in functional components.
One of the most popular Hooks is useState
. It allows you to add state to your functional components. With useState
, you can initialize state and update it as needed. It simplifies the code and enhances readability.
Another essential Hook is useEffect
. It lets you perform side effects in your components. Whether fetching data or subscribing to events, useEffect
handles it efficiently. It replaces the lifecycle methods used in class components.
React Hooks also encourage better component composition. They allow you to extract and reuse stateful logic. This promotes cleaner and more maintainable code. Developers can now share logic across components without altering the component hierarchy.
Here are some benefits of using Hooks in your React projects:
- Improved code readability and simplicity
- Enhanced reusability of stateful logic
- Seamless integration with functional components
- Reduced complexity of lifecycle management
- Encouragement of best practices in React development
Transitioning to Hooks from class components might seem daunting at first. However, the advantages they bring make the effort worthwhile. React Hooks are a game-changer, simplifying how we handle state and side effects in React.
React and the Virtual DOM
React has revolutionized the way developers build user interfaces. One of its standout features is the Virtual DOM. But what exactly is the Virtual DOM, and why does it matter? For developers diving into React, understanding the Virtual DOM is crucial.
The Virtual DOM is a lightweight copy of the actual DOM. React uses this to optimize rendering. When you make changes to your app, React updates the Virtual DOM. It then compares this with the real DOM to determine the minimal number of changes needed. This process is known as “reconciliation.”
Why does this matter? In modern web development, performance is key. Frequent updates to the real DOM can be slow and inefficient. By using the Virtual DOM, React minimizes direct manipulation of the actual DOM. This results in faster updates and a smoother user experience.
Additionally, the Virtual DOM enables React’s declarative nature. Developers describe what the UI should look like at any given time. React then handles the task of updating the DOM to match this description. This abstraction allows developers to focus on building features rather than optimizing performance.
Furthermore, the Virtual DOM supports React’s component-based architecture. Each component maintains its own Virtual DOM. When changes occur, only the affected components update. This makes React both efficient and scalable, perfect for large applications.
Embracing the Virtual DOM means embracing a more intuitive and efficient way to develop web applications. It’s a core reason why React has become a favorite among developers worldwide.
Popular Tools and Libraries for React Development
React has gained immense popularity among developers for its component-based architecture. This has led to a flourishing ecosystem of tools and libraries that enhance the development experience.
First, let’s talk about Redux. It’s a state management library that helps maintain a consistent application state. Developers love it for its predictability and ease of debugging.
Then, there’s React Router. This library is essential for handling navigation in single-page applications. It enables dynamic routing based on the app’s state and URL.
Another vital tool is Webpack. It bundles JavaScript modules, optimizing them for performance. Webpack supports hot reloading, which speeds up development by updating modules without a full page refresh.
For styling, Styled-components is a popular choice. It allows you to write CSS in JavaScript, making it easier to manage styles within components. This approach promotes better organization and reuse.
Additionally, Enzyme is a testing utility for React. It simplifies the task of asserting, manipulating, and traversing React components. Enzyme works well with testing frameworks like Jest.
Finally, consider using Create React App. This tool helps set up a new React project with a sensible default configuration. It saves time and allows developers to focus on coding rather than configuration.
In summary, these tools and libraries streamline React development, making it more efficient and enjoyable. Each one has its unique strengths, catering to various aspects of the development process.
React in the Modern Web Development
React has become a cornerstone in modern web development. As a JavaScript library, it helps developers create user interfaces efficiently. It focuses on building reusable components, which makes code more maintainable and scalable.
One of React’s strengths is its virtual DOM. This feature minimizes direct updates to the actual DOM, making applications faster. With React, developers can create dynamic and responsive web apps that provide a seamless user experience.
Moreover, React’s component-based architecture allows for better code organization. Developers can encapsulate logic and styling within components. This encapsulation simplifies debugging and testing, enhancing overall productivity.
React also integrates well with other modern tools and libraries. Whether you’re using Redux for state management or Tailwind for styling, React’s ecosystem is rich and supportive. This compatibility ensures developers can build complex applications with ease.
Transitioning to modern web development practices, React supports server-side rendering and static site generation. These capabilities improve performance and SEO, crucial for today’s competitive web environment. As a result, websites load faster and rank better on search engines.
In the ever-evolving landscape of web development, React continues to adapt. The introduction of hooks and concurrent mode showcases its commitment to innovation. These features allow developers to write cleaner code and handle asynchronous operations effortlessly.
React’s active community is another significant advantage. Developers can find countless resources, from documentation to tutorials. This support network fosters knowledge sharing and problem-solving, accelerating development cycles.
For developers, React offers a powerful toolset in the world of JavaScript. It streamlines the process of building interactive web applications. With its robust features and vibrant community, React remains a crucial player in the modern web development arena.
Conclusion
React has transformed the way developers build user interfaces, offering a component-based architecture that enhances both efficiency and maintainability. Its virtual DOM ensures high performance, making it a popular choice for fast, dynamic applications. This library’s rich ecosystem, including tools like Redux and React Router, further streamlines application development.
Moreover, React’s strong community support ensures continuous improvement and abundant resources, facilitating problem-solving and learning. As the demand for interactive web applications grows, mastering React becomes increasingly valuable. It empowers developers to create seamless user experiences with less effort and more flexibility.
By understanding its core principles and leveraging its extensive ecosystem, developers can craft robust, scalable applications. React not only simplifies UI development but also opens doors to innovative solutions, making it a critical skill in today’s tech landscape.
Previous
What is JSX?
Next