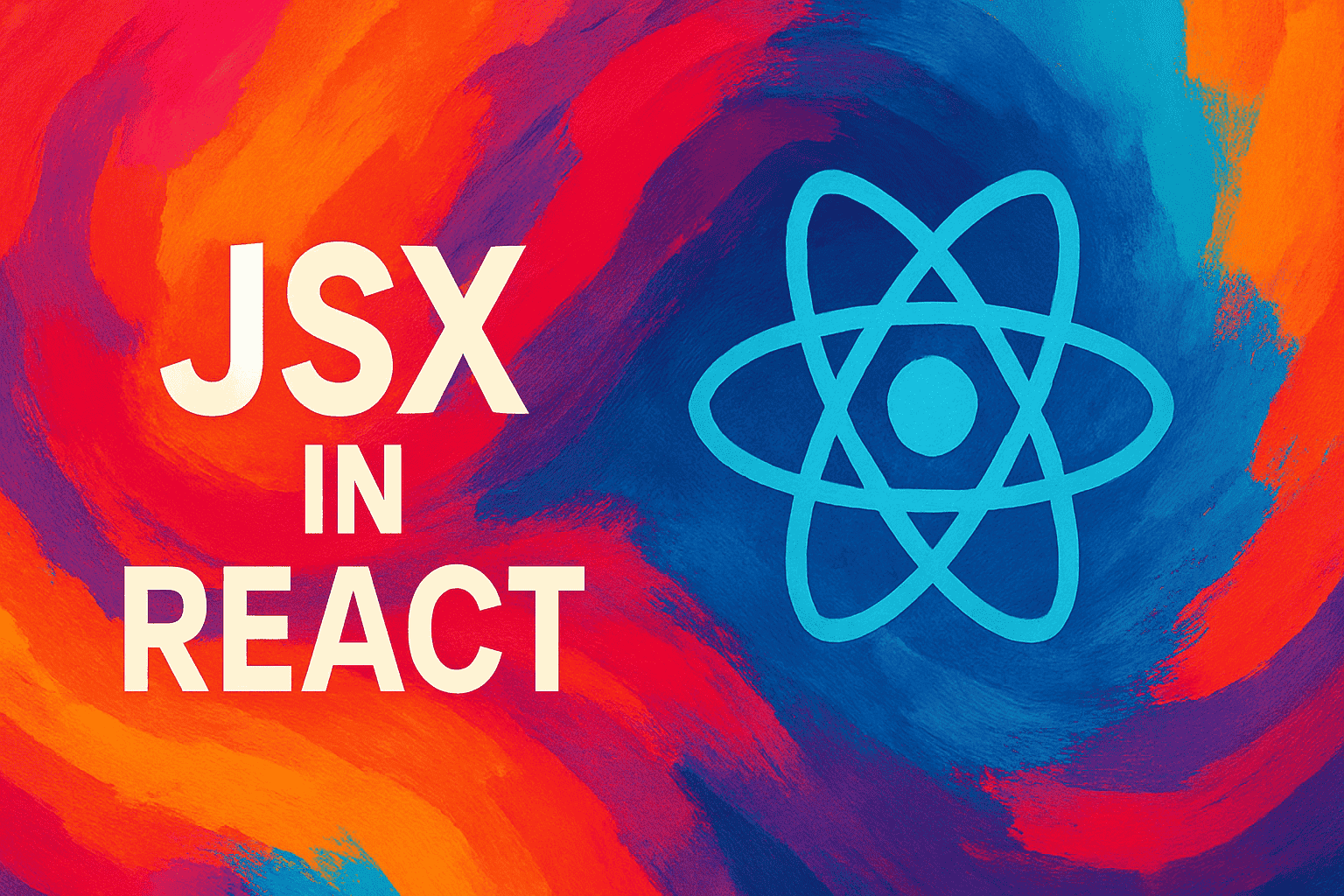
Fast Answers
What is JSX?
JSX, or JavaScript XML, is a syntax extension for JavaScript. It is primarily used with React to describe UI components. JSX looks like HTML, but it functions like JavaScript, allowing developers to write concise and expressive code.
When using JSX, you can create React elements with a familiar syntax. By embedding expressions in curly braces, you can dynamically render content. This feature makes it intuitive for developers who are accustomed to HTML but want the power of JavaScript.
One of the main benefits of JSX is its ability to create interactive and dynamic interfaces. Instead of manipulating the DOM directly, you write components that React efficiently renders. This declarative approach improves code readability and maintainability.
Transitioning to JSX is smooth for those with a grounding in HTML and JavaScript. With JSX, you can leverage the full power of JavaScript. Functions, loops, and conditions can be used directly within your markup, making your code more dynamic and responsive.
While JSX is not required to use React, it simplifies the process of building component-based architectures. It allows developers to write cleaner and more organized code. As a result, your applications can become more scalable and easier to debug.
Origin and Evolution of JSX
JSX, or JavaScript XML, emerged as a powerful extension of JavaScript. It was introduced by Facebook in 2013 alongside the React library. The aim was to streamline the process of creating interactive user interfaces.
Before JSX, developers used JavaScript to manipulate the DOM directly. This approach was often cumbersome and error-prone. JSX simplified the process by allowing developers to write HTML-like syntax directly within JavaScript code. This innovation made the code more readable and approachable.
React’s rise in popularity further propelled the adoption of JSX. Developers quickly recognized its benefits, such as improved code clarity and easier debugging. JSX made it possible to visualize the UI structure while coding, bridging the gap between design and logic.
Over time, the syntax and functionality of JSX have evolved. Initially, some developers were skeptical about mixing markup with logic. However, the benefits of a unified syntax outweighed these concerns. It enabled a more cohesive development process, reducing context switching between files.
Modern development practices often involve using JSX with Babel, a JavaScript compiler. Babel transforms JSX into standard JavaScript, ensuring compatibility across different environments. This transformation is seamless, allowing developers to write JSX without worrying about browser support.
Today, JSX is not limited to React alone. Other frameworks and libraries have adopted similar concepts, recognizing the advantages of a hybrid syntax. The evolution of JSX highlights the ongoing quest for more efficient and intuitive coding paradigms in web development.
Why Use JSX in Development?
JSX, or JavaScript XML, is a syntax extension that allows developers to write HTML structures within JavaScript code. This approach brings a host of benefits, especially when working with React. Let’s explore why developers prefer JSX in their development process.
Firstly, JSX makes the code more readable and elegant. By allowing HTML-like syntax within JavaScript, it bridges the gap between design and functionality. This readability reduces the cognitive load on developers, making it easier to understand and maintain the codebase.
Secondly, JSX is powerful in terms of componentization. It helps in building reusable components with ease. This reusability saves time and effort, ensuring consistency across different parts of the application.
Moreover, JSX can help catch errors early. During the compilation process, JSX identifies and flags syntax errors before the code runs. This preemptive error detection is crucial in maintaining a robust application.
Another key advantage is the virtual DOM. JSX is designed to work seamlessly with React’s virtual DOM, which optimizes updates and improves performance. This efficiency is vital for applications that demand high responsiveness and speed.
Additionally, JSX enables developers to leverage JavaScript’s full power. You can integrate expressions, loops, and conditions directly into your markup. This flexibility allows for dynamic and interactive user interfaces.
In summary, JSX is not just a syntactic sugar but a tool that enhances development productivity and application performance. By combining the best of HTML and JavaScript, JSX offers a streamlined and efficient approach to building modern web applications.
Key benefits of using JSX:
- Improved code readability
- Enhanced component reusability
- Early error detection
- Optimized performance with virtual DOM
- Dynamic and interactive UI possibilities
Syntax and Structure of JSX
JSX, or JavaScript XML, enables developers to write HTML within JavaScript. It allows a seamless blend of HTML and JavaScript, making the code more readable and expressive. But, understanding its syntax is crucial for effective use.
JSX looks similar to HTML, but it has its quirks. For instance, in JSX, you can’t use HTML attributes directly. Instead, you must use camelCase for attributes. For example, instead of “class,” you use “className” because “class” is a reserved keyword in JavaScript.
Let’s see how JSX works with a simple example:
const element =Hello, world!;
In this example, an HTML-like structure is embedded within JavaScript. The JSX code gets transpiled into regular JavaScript using a tool like Babel, which converts it to React.createElement()
calls.
JSX also allows embedding JavaScript expressions within curly braces. This feature is powerful for rendering dynamic content. Here’s an example:
const name = "John"; const element =Hello, {name}!;
Here, the {name}
is a JavaScript expression within JSX, which evaluates to “John.” This makes JSX versatile for creating dynamic and interactive UIs.
JSX syntax also supports nesting elements, just like HTML. You can create parent and child elements effortlessly:
const element = ();Hello, world!Welcome to learning JSX.
JSX is a potent tool for building user interfaces in React applications. Understanding its syntax helps developers write cleaner, more efficient code.
JSX vs Traditional JavaScript
When working with web development, developers often encounter JSX and traditional JavaScript. Understanding the differences is crucial for crafting efficient, maintainable code. Let’s dive into how JSX stands apart from the standard JavaScript.
Traditional JavaScript uses functions like document.createElement
to manipulate the DOM. This approach can be tedious and error-prone, especially for complex UI structures. Consider the example below.
// Traditional JavaScript Example const element = document.createElement('div'); element.className = 'container'; const text = document.createTextNode('Hello, World!'); element.appendChild(text); document.body.appendChild(element);
In contrast, JSX simplifies this process by allowing developers to write HTML-like syntax directly within JavaScript. This blend of HTML and JavaScript offers a more intuitive way to create UI components. Here’s the same example in JSX:
// JSX Example const element =Hello, World!; ReactDOM.render(element, document.getElementById('root'));
JSX accelerates development by making code more readable and reducing boilerplate. However, it’s important to remember that JSX is not a complete replacement for JavaScript. It is a syntax extension that ultimately compiles to JavaScript.
- Pros:
- JSX syntax is more readable and intuitive.
- Combines HTML and JavaScript seamlessly.
- Cons
- Requires a build step to transform to JavaScript.
- Learning curve for developers new to React.
Transitioning from traditional JavaScript to JSX offers a modern approach to building dynamic web applications. While it introduces some initial complexity with build tools, the long-term benefits in readability and maintainability are substantial.
Integration with React
JSX, or JavaScript XML, is a critical part of working with React. It allows developers to write HTML elements directly in JavaScript, enhancing the synergy between JavaScript logic and HTML content. When you integrate JSX with React, you benefit from a more intuitive and readable code structure.
In a typical React application, JSX enables you to define UI components succinctly. These components are the building blocks of React applications. With JSX, you can mix JavaScript expressions and HTML-like syntax, making the code more expressive.
Consider a simple React component. It can render a message using JSX:
const Greeting = () => { const name = 'Developer'; returnHello, {name}!; };
In this example, the function Greeting
returns an h1
element. The curly braces allow you to embed JavaScript expressions within the JSX, providing dynamic content rendering.
JSX also simplifies the creation of complex UIs by allowing developers to nest components. This modularity promotes code reuse and maintainability. Here’s an example of a parent component rendering child components:
const App = () => { return (); };Welcome to the React world!
JSX transforms into regular JavaScript calls via tools like Babel. This conversion ensures compatibility with browsers, which do not understand JSX directly. As a result, developers can write code that’s both human-readable and machine-compatible.
In conclusion, integrating JSX with React streamlines the development process. It brings a unique blend of HTML and JavaScript, fostering a more efficient coding experience. With practice, developers can harness the full potential of JSX to build dynamic and interactive user interfaces.
Benefits of Using JSX
JSX, or JavaScript XML, is a syntax extension for JavaScript. It allows developers to write HTML directly within React components, blending the strength of JavaScript with the readability of HTML.
Here are some key benefits of using JSX:
- Improved Readability: JSX syntax closely resembles HTML, making it easier to read and understand. This is especially useful for teams collaborating on large codebases.
- Component Logic and UI in One Place: By writing UI and logic together, JSX improves maintainability. This reduces the cognitive load as developers don’t need to switch between files.
- Enhanced Error Detection: JSX includes compile-time checks, catching errors early in the development process. This leads to fewer bugs and smoother debugging.
- Full JavaScript Support: With JSX, you can leverage JavaScript’s powerful features directly in your UI code. This includes loops, conditionals, and object destructuring.
- Consistent Development Experience: JSX provides a consistent development experience across browsers and devices. It ensures that your code behaves as expected, regardless of the environment.
JSX also facilitates dynamic rendering. For example, you can embed JavaScript expressions within JSX:
const name = 'World'; const element =Hello, {name}!;
In this example, the name
variable is seamlessly embedded within the JSX. This flexibility makes JSX a powerful tool for creating interactive and dynamic UIs.
Common Pitfalls and How to Avoid Them
JSX is an incredible tool that enhances JavaScript with a syntax extension allowing you to write HTML directly within JavaScript. However, developers often face some common pitfalls with JSX. Being aware of these can significantly improve your development experience.
return (HelloWorld
> );
Another pitfall is confusing HTML attributes with JSX attributes. JSX uses camelCase for attribute names instead of the usual HTML attribute names. For example, instead of class
, use className
. This might seem trivial, but it can lead to unexpected bugs.
returnContent;
Furthermore, always ensure that your expressions in JSX are wrapped in curly braces. Forgetting this can lead to syntax errors. Keep your code clean and readable by consistently using curly braces for dynamic expressions.
const element =Hello, {userName}!;
By being cautious of these pitfalls, you can make the most out of JSX and ensure your JavaScript code is robust and efficient. Happy coding!
Conclusion: Embracing JSX
JSX, or JavaScript XML, offers a powerful way to build dynamic user interfaces in React applications. Its syntax extension allows developers to write HTML elements directly in JavaScript, bridging the gap between JavaScript and UI components.
By embracing JSX, developers can create more readable and maintainable code. It enhances productivity by eliminating the need to juggle between multiple files for HTML and JavaScript logic. This streamlined approach leads to faster development cycles and a more cohesive coding experience.
Moreover, JSX encourages a more intuitive way of thinking about UI components as a blend of logic and presentation. This blending fosters a more integrated development process, where developers can focus on creating rich user experiences without getting bogged down by traditional separation between JavaScript and HTML.
In the ever-evolving landscape of web development, adopting JSX can be a game-changer. Its ability to simplify complex interfaces while maintaining a clean codebase makes it an invaluable tool for modern developers. As you delve deeper into JSX, you’ll find it not only enhances your code but also elevates your overall development strategy.
Previous
How to create a dictionary in typescript?
Next