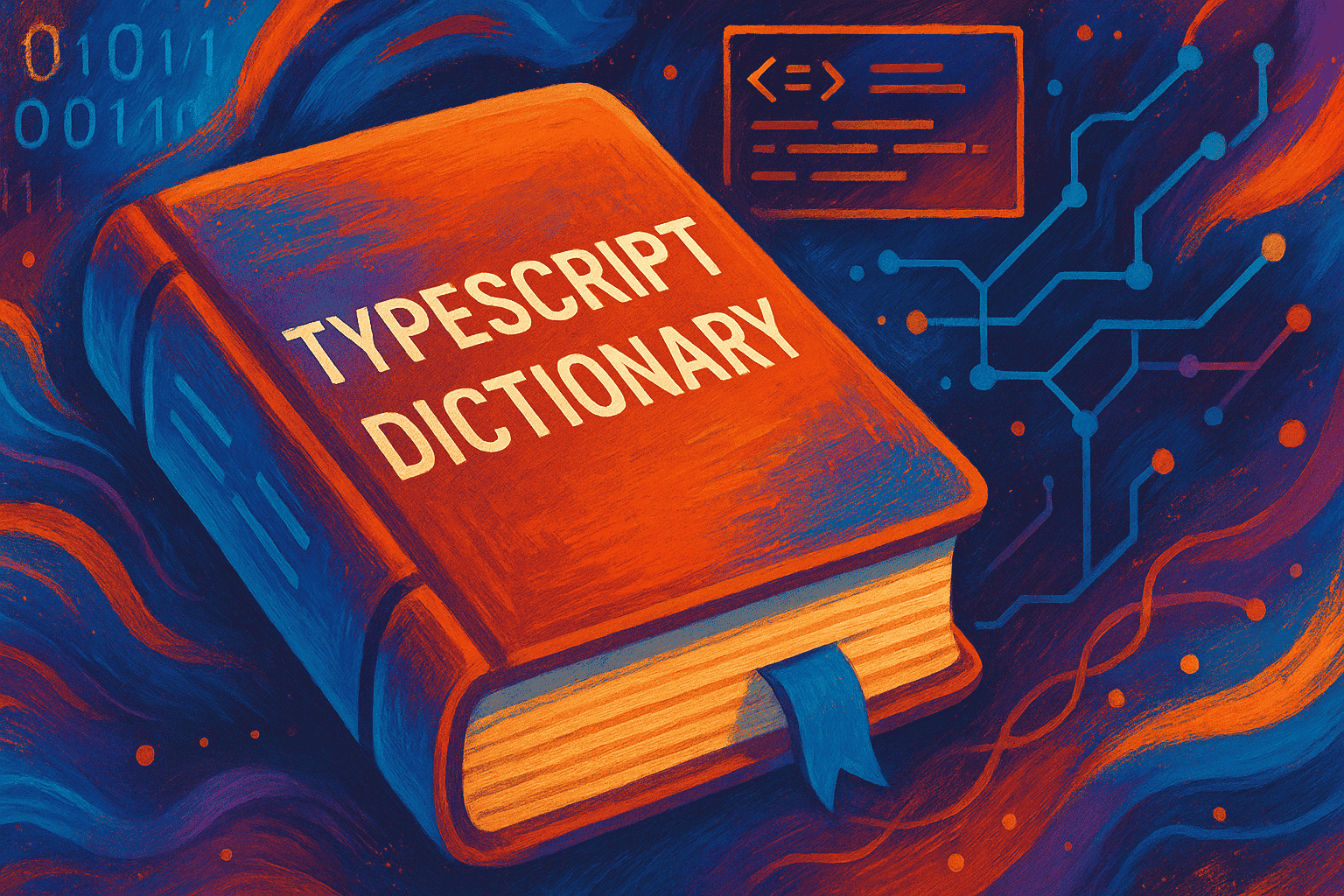
Fast Answers
Introduction to TypeScript Dictionaries
TypeScript, a superset of JavaScript, offers a robust typing system. Among its many features, dictionaries stand out due to their flexibility and utility. As a developer, you often need to handle collections of key-value pairs. This is where TypeScript dictionaries come into play.
In JavaScript, objects are commonly used as dictionaries. However, TypeScript enhances this by allowing you to define the types of both keys and values. This not only improves code readability but also helps catch errors at compile time. In TypeScript, dictionaries can be created using interfaces or types. This adds a layer of type safety that JavaScript lacks.
To create a dictionary in TypeScript, you define an interface or a type with an index signature. This allows the dictionary to accept keys of a specified type and ensures that the values conform to a particular type. Here’s a simple example to illustrate this concept:
interface StringDictionary { [key: string]: string; } const myDictionary: StringDictionary = { firstName: "John", lastName: "Doe", };
In this example, the StringDictionary
interface defines a dictionary where both keys and values are strings. This means you can add any key-value pairs as long as they adhere to the defined types. TypeScript will enforce these constraints, making your code less prone to runtime errors.
Additionally, you can use types to achieve the same result. For instance:
type NumberDictionary = { [key: string]: number; }; const ageDictionary: NumberDictionary = { alice: 25, bob: 30, };
Here, the NumberDictionary
type ensures that all values are numbers. This flexibility allows you to craft dictionaries tailored to your application’s needs. Whether you’re dealing with strings, numbers, or even custom objects, TypeScript dictionaries provide the structure and type safety you need to build reliable software.
Understanding Types and Interfaces
In TypeScript, understanding types and interfaces forms the backbone of creating a robust and efficient dictionary. Types define how variables, functions, and return values should behave. On the other hand, interfaces provide a structured way to define object shapes, making code more readable and maintainable.
When creating a dictionary in TypeScript, leveraging interfaces allows you to specify the exact structure of the key-value pairs. This provides clarity and reduces errors, especially in complex applications. Interfaces can also extend other interfaces, allowing for reusable and scalable code.
Consider a scenario where you need a dictionary to store user information. You can define an interface to outline the structure of each entry:
Code Example interface UserInfo { name: string; age: number; email: string; } interface UserDictionary { [id: string]: UserInfo; } const userDict: UserDictionary = { 'user1': { name: 'Alice', age: 30, email: 'alice@example.com' }, 'user2': { name: 'Bob', age: 25, email: 'bob@example.com' } };
This example showcases how interfaces help structure the dictionary. The UserInfo interface specifies what each user entry should contain, while the UserDictionary interface uses an index signature to allow dynamic keys. This approach ensures type safety and makes your code self-documenting.
Types add another layer of flexibility, allowing you to define complex data structures. You can use union and intersection types to combine different types, enhancing the functionality of your dictionary.
Types and interfaces, when used effectively, can significantly improve the quality of your TypeScript code. They help you avoid common pitfalls by providing a clear contract for your data structures. This leads to code that is not only easier to debug but also easier to scale and evolve with your application.
Creating a Simple Dictionary in TypeScript
In the world of TypeScript, creating a simple dictionary can be both efficient and straightforward. TypeScript’s type system allows you to define a dictionary with clear, understandable types, making your code robust and maintainable. Developers often need to store key-value pairs, and TypeScript provides an elegant solution for this.
A dictionary in TypeScript can be implemented using an interface. This interface defines the type of the keys and values. Typically, keys are strings, and values can be any type, giving you flexibility. Here’s a simple example:
interface Dictionary{ [key: string]: T; }
In this example, the Dictionary
interface allows for any type of value. The key is defined as a string. You can now create a dictionary to store, for example, numbers:
let numberDictionary: Dictionary= {}; numberDictionary['one'] = 1; numberDictionary['two'] = 2;
This setup is not only simple but also type-safe. It ensures that the values stored are of the specified type. By using TypeScript’s type inference, you can avoid common runtime errors. Moreover, you can also iterate over this dictionary with ease:
for (let key in numberDictionary) { console.log(`${key}: ${numberDictionary[key]}`); }
The dictionary pattern is versatile. You can define dictionaries with complex objects as values, enhancing your application’s capabilities. TypeScript’s dictionary feature can handle various scenarios, making it a powerful tool for developers. This approach not only improves code readability but also aligns with best practices for modern web development.
Using the Index Signature
As developers, we often need to work with collections of data, and creating a dictionary in TypeScript is a common task. One powerful feature of TypeScript that facilitates this is the index signature. Let’s dive into how you can use it effectively.
An index signature allows you to define objects with dynamic keys, where each key is associated with a value of a specific type. This is particularly useful when you don’t know the exact properties in advance but know the shape of the data you’ll handle.
Here’s how you can define a dictionary using the index signature in TypeScript:
interface StringDictionary { [key: string]: string; } const userRoles: StringDictionary = { admin: "Administrator", user: "Regular User", guest: "Guest User" };
In the example above, the StringDictionary
interface utilizes an index signature, defined by [key: string]
. This indicates that any string key is valid and the corresponding value should be a string. This flexibility makes it easy to manage dynamic sets of data.
Moreover, you can combine the index signature with other properties in an interface. For instance:
interface EnhancedDictionary { default: string; [key: string]: string; } const appSettings: EnhancedDictionary = { default: "defaultSetting", theme: "dark", language: "en" };
In this scenario, EnhancedDictionary
has a predefined property default
, alongside other dynamic properties. This flexibility enhances the way you can structure your data.
Using index signatures is an effective way to create adaptable data structures in TypeScript. They provide a clean and efficient approach to handle keyed collections without sacrificing type safety. As you continue to build complex applications, the ability to manage dynamic data flexibly will be invaluable.
Defining Value Types in a Dictionary
In TypeScript, creating a dictionary involves more than just key-value pairs. It’s crucial to define the types for the values, ensuring consistency and avoiding runtime errors. This step is vital as it leverages TypeScript’s powerful type-checking capabilities, providing developers with a robust coding environment.
When defining value types in a dictionary, you start by specifying the key type and the value type. Typically, keys are strings or numbers, while values can be any data type. This flexibility allows developers to create dictionaries tailored to their needs, whether simple or complex.
To define a dictionary, use an interface or a type alias. This provides a blueprint for what the dictionary should look like. By doing so, you prevent common errors and maintain a clean codebase. Here’s an example of defining a dictionary where the values are numbers:
type NumberDictionary = { [key: string]: number; }; const scores: NumberDictionary = { "Alice": 90, "Bob": 85, "Charlie": 92 };
In this example, we declare a type alias NumberDictionary
. It expects string keys and number values. This enforces that all values in the scores
dictionary are numbers, ensuring type safety. If you attempt to assign a non-number value, TypeScript will catch it during compilation.
For more complex scenarios, you might define dictionaries with object values. This is useful when you need to store additional information about each entry. Here’s a brief example:
type User = { name: string; age: number; }; type UserDictionary = { [key: string]: User; }; const users: UserDictionary = { "user1": { name: "Alice", age: 30 }, "user2": { name: "Bob", age: 25 } };
By using the UserDictionary
, you ensure that each value is an object with a specific structure. This adds another layer of reliability and clarity to your code. Defining value types in a dictionary is a best practice that can significantly enhance your TypeScript projects.
Iterating Over Dictionary Elements
When working with dictionaries in TypeScript, you often need to iterate through each element. This task is straightforward and can be accomplished using several methods. Let’s explore some of the most common ways to iterate over dictionary elements in TypeScript.
The simplest method to iterate over a dictionary is by using the for...in
loop. It allows you to loop through the keys of the dictionary, which you can then use to access the values.
let myDictionary: { [key: string]: number } = { "one": 1, "two": 2, "three": 3 }; for (let key in myDictionary) { if (myDictionary.hasOwnProperty(key)) { console.log(key + ": " + myDictionary[key]); } }
Using the Object.keys()
method is another efficient way. It returns an array of the keys, which can be iterated over using forEach
or a for...of
loop.
Object.keys(myDictionary).forEach(key => { console.log(key + ": " + myDictionary[key]); });
If you prefer to work directly with entries, the Object.entries()
method is a great choice. It provides key-value pairs from the dictionary, allowing for easy access to both elements in a single iteration.
for (let [key, value] of Object.entries(myDictionary)) { console.log(key + ": " + value); }
These techniques not only make your code cleaner but also offer flexibility when dealing with complex data structures. By choosing the appropriate method, you can effectively manage and manipulate dictionary elements in TypeScript.
Advanced Techniques with TypeScript Dictionaries
TypeScript dictionaries, also known as maps or objects, are a powerful tool for developers. They allow you to store key-value pairs, providing flexible and efficient data management. Let’s delve into advanced techniques that can enhance your TypeScript dictionaries.
One essential technique is utilizing the Record
utility type. This type ensures that all keys are of a specific type, enhancing type safety and reducing errors. Here’s an example:
type UserRoles = 'admin' | 'editor' | 'viewer'; const userPermissions: Record<UserRoles, string> = { admin: 'all-access', editor: 'write-access', viewer: 'read-only' };
Another technique is leveraging intersection types. This allows you to combine multiple types into one, creating a more comprehensive dictionary. Consider this example:
interface BaseUser { name: string; age: number; } interface AdminPrivileges { accessLevel: string; } type AdminUser = BaseUser & AdminPrivileges; const admin: AdminUser = { name: 'Alice', age: 30, accessLevel: 'superuser' };
TypeScript also allows you to create dictionary types with optional properties. This is particularly useful when some data might be missing or undefined. Here’s how you can define optional properties:
interface UserProfile { username: string; email?: string; // Optional property } const user: UserProfile = { username: 'john_doe' // email is optional };
These advanced techniques make TypeScript dictionaries more robust and adaptable. By ensuring type safety and flexibility, you can write cleaner and more maintainable code. As you experiment with these techniques, you’ll find TypeScript offers a wealth of features to streamline your development process.
Practical Use Cases for TypeScript Dictionaries
TypeScript dictionaries, or objects, are powerful tools. They are ideal for managing collections of key-value pairs. Developers often use them in a variety of contexts. Let’s explore some practical use cases.
One common use case is storing configuration settings. Instead of hard-coding values, store them in a dictionary. This makes it easy to change settings without altering code logic. Here’s an example:
const configSettings = { apiUrl: "https://api.example.com", timeout: 5000, retryAttempts: 3 };
Another use case is mapping data from APIs. When working with external data sources, the structure might not match your application. Use a dictionary to map incoming data to your application’s format:
const apiResponse = { "user_name": "JohnDoe", "user_email": "john@example.com" }; const userMapping = { username: apiResponse.user_name, email: apiResponse.user_email };
Dictionaries can also manage collections of functions. This pattern is useful for executing different operations based on dynamic keys. Consider the following example:
const operations = { add: (a, b) => a + b, subtract: (a, b) => a - b }; const result = operations["add"](5, 3); // 8
These examples demonstrate the versatility of TypeScript dictionaries. They simplify complex operations and enhance code readability. Embrace them to improve your development workflow.
Summary
Creating a dictionary in TypeScript allows developers to manage key-value pairs efficiently. TypeScript enhances JavaScript with strong typing, making dictionaries powerful and reliable. In TypeScript, dictionaries are often represented using objects or maps.
Developers can use the type system to define the shape of their data. This ensures that only the specified types are used as keys and values. Objects can serve as dictionaries when keys are strings or symbols. For more complex requirements, the Map
object offers flexibility with any data type as keys.
TypeScript’s type inference and interfaces help in defining dictionary types explicitly. This approach improves code readability and maintainability. By leveraging TypeScript’s features, developers can create robust applications that handle data efficiently.
Moreover, using TypeScript dictionaries promotes cleaner code by preventing runtime errors. With TypeScript, developers gain the confidence that their dictionaries behave as expected. This ultimately results in more stable and predictable applications.
Previous
How to use spectral in typescript?
Next