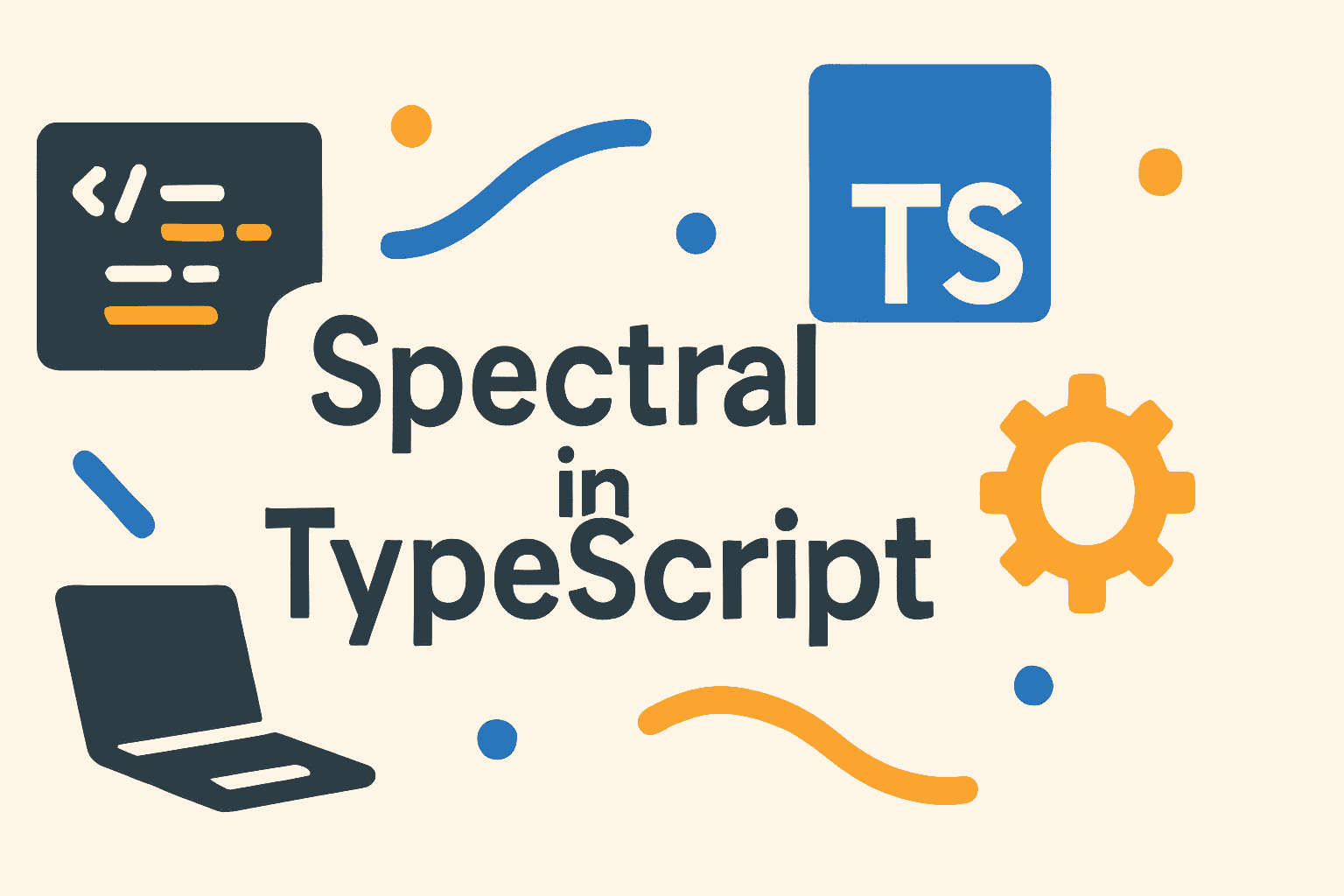
Fast Answers
Introduction to Spectral in TypeScript
In the world of API development, maintaining consistency and ensuring quality is paramount. This is where Spectral comes into play. Spectral is a flexible JSON/YAML linter that helps developers enforce rules on API specifications. When working with TypeScript, integrating Spectral can significantly streamline your workflow.
Spectral allows you to create custom rules or use predefined ones for OpenAPI, AsyncAPI, and other formats. It acts as a safeguard against common pitfalls by automating checks on your API definitions. With TypeScript, Spectral’s TypeScript SDK offers type safety, enhancing the robustness of your code.
To get started, install Spectral via npm. Use the following command in your terminal: npm install @stoplight/spectral
. Once installed, you can begin by creating a configuration file, typically named .spectral.yml
. This file is where you define the rules you want Spectral to enforce.
Integrating Spectral into your TypeScript project involves writing a script that utilizes the Spectral SDK. This script will parse your API documents and validate them against the rules defined in your configuration file. The following is a simple example:
import { Spectral, Document } from '@stoplight/spectral-core'; import { readFileSync } from 'fs'; const spectral = new Spectral(); async function lintDocument(filePath: string) { const documentContent = readFileSync(filePath, 'utf-8'); const document = new Document(documentContent, filePath); await spectral.loadRuleset('spectral:oas'); const results = await spectral.run(document); results.forEach(result => { console.log(`${result.code} - ${result.message}`); }); } lintDocument('path/to/your/api-document.yaml');
This script reads an API document and lints it using Spectral’s OpenAPI ruleset. The results are logged, highlighting any issues found. Transitioning to using Spectral can seem daunting at first, but its integration within TypeScript makes the process smoother and more efficient.
By leveraging Spectral, developers can ensure their API specifications adhere to industry standards and best practices. This not only improves the quality of the APIs but also fosters collaboration and consistency across teams.
Installing and Setting Up Spectral
To effectively use Spectral in TypeScript, you must first install and configure it properly. Spectral is a fantastic tool for linting and validating your OpenAPI specifications. Here’s a step-by-step guide to get you started on the right foot.
First, ensure you have Node.js and npm installed on your system. These are essential for managing and installing packages. If you haven’t done so yet, download and install them from the official Node.js website.
Once your environment is ready, you can install Spectral using npm. Simply run the following command in your terminal:
npm install -g @stoplight/spectral
This command installs Spectral globally, making it available for use in any project. After installation, you can verify the installation by running:
spectral --version
If the version number appears, you’re all set. Next, let’s configure Spectral for TypeScript. Create a configuration file named .spectral.yml
in your project’s root directory. This file will define the rules you’d like Spectral to enforce.
Here’s an example configuration:
rules: operation-2xx-response: description: "Operations must have at least one 2xx response" recommended: true type: "style"
After setting up your rules, you can run Spectral against your OpenAPI files. Use the following command to lint your specification:
spectral lint openapi.yaml
With these steps, you have successfully installed and configured Spectral. Now, you’re ready to enhance your API development workflow with improved specification linting!
Understanding Spectral Rules
As developers, maintaining consistency and ensuring quality in API specifications are crucial. Spectral is a powerful tool that helps enforce rules and best practices in your OpenAPI, AsyncAPI, and JSON Schema documents. Understanding spectral rules can significantly enhance your API development process.
Spectral rules are essentially guidelines that your API specifications must adhere to. These rules can range from ensuring property names follow a specific naming convention to enforcing that every endpoint has a description. The beauty of Spectral lies in its flexibility to allow custom rule creation to fit your project’s specific needs.
To get started with Spectral in TypeScript, first install Spectral using npm:
npm install @stoplight/spectral-cli
Once installed, you can define your rules in a configuration file, typically named .spectral.yml
. Here’s a simple example of a spectral rule configuration:
rules: description-must-not-be-empty: description: "All operations should have a non-empty description" given: "$.paths[*][*]" then: field: description function: truthy
In this example, the rule checks that every operation in your API has a non-empty description. The given
keyword specifies the target paths to evaluate, and then
defines the condition to be satisfied.
Running Spectral against your API specification is straightforward:
npx spectral lint my-api.yaml
This command will validate your API file my-api.yaml
against the rules defined in your .spectral.yml
configuration file.
By leveraging Spectral rules, you can automate the enforcement of your API standards, leading to more robust and maintainable specifications. Transitioning to using Spectral in your workflow can save time and reduce errors, ensuring a smoother development process.
How to Create Custom Rules
When using Spectral in TypeScript, creating custom rules can enhance your code quality. Custom rules allow developers to enforce specific coding standards and catch errors early in the development process. This section will guide you through the steps of creating custom rules with Spectral.
First, understand that Spectral is a tool designed for linting JSON and YAML files. It is often used for API specifications like OpenAPI or AsyncAPI. To create a custom rule, you’ll need to define the rule in a way that Spectral can interpret and apply it during the linting process.
Begin by adding a custom rule to your Spectral configuration file. The configuration file is usually a YAML or JSON file that Spectral reads to determine which rules to apply.
rules: my-custom-rule: description: "Ensure all paths start with /api" message: "Path should start with /api" given: "$.paths[*]~" then: function: pattern functionOptions: match: "^/api"
The example above demonstrates a custom rule that checks if all paths in an API specification start with “/api”. The given field specifies the JSON path to evaluate. The then field specifies the function Spectral should use to enforce the rule. In this case, it’s a pattern match.
To implement this, you’ll need to write the logic for the rule in a JavaScript file. Spectral allows you to use custom JavaScript functions for more complex validation scenarios.
module.exports = { rules: { 'my-custom-rule': { description: 'Ensure all paths start with /api', given: '$.paths[*]~', then: { function: 'pattern', functionOptions: { match: '^/api', }, }, }, }, };
After defining your custom rule, you can run Spectral using your configuration file. This will evaluate your API specifications against the custom rules you’ve set. If any paths do not start with “/api”, Spectral will flag them, helping you maintain a consistent API design.
Creating custom rules in Spectral is a powerful way to enforce coding standards and catch potential issues early. By tailoring rules to your project’s needs, you can ensure code quality and consistency across your development team.
Integrating Spectral with Your Development Workflow
Integrating Spectral into your TypeScript development workflow can significantly enhance your code quality and maintainability. Spectral is a powerful tool for linting and validating OpenAPI documents. Here’s how you can seamlessly integrate it into your workflow.
Why Use Spectral?
Spectral helps you catch errors early in the development cycle. It provides rules that ensure your API specifications are consistent and adhere to best practices. This proactive approach saves time and reduces bugs in production.
Setting Up Spectral
To begin, you need to install Spectral. You can do this using npm:
npm install -g @stoplight/spectral
Once installed, you can use Spectral from the command line to lint your OpenAPI documents:
spectral lint openapi.yaml
Integrating with TypeScript
Integrating Spectral into a TypeScript project can further streamline your process. You can automate linting with npm scripts or integrate it into your CI/CD pipeline. Here’s a simple npm script example:
{ "scripts": { "lint:api": "spectral lint openapi.yaml" } }
By adding this script to your package.json
, you can run the lint command with npm run lint:api
.
Customizing Spectral Rules
You can customize Spectral rules to fit your project’s specific needs. Create a .spectral.yml
file to define custom rules.
rules: my-custom-rule: description: "Check for a custom condition" given: "$" then: field: "info" function: "truthy"
With this rule, Spectral will ensure that the info
field in your OpenAPI document is always truthy.
Benefits of Automation
Automating Spectral in your development workflow ensures consistent quality checks. It reduces manual errors and keeps your API documentation up to standard effortlessly.
Embrace Spectral to maintain high-quality API specifications and streamline your development process.
Using Spectral for API Validation
APIs are the backbone of modern applications, enabling diverse systems to communicate. Ensuring their reliability and consistency is crucial. This is where Spectral comes into play. It is a flexible JSON/YAML linter for creating quality APIs. In this section, we explore how to use Spectral in a TypeScript environment.
Spectral allows developers to define rules that validate API specifications, such as OpenAPI or AsyncAPI documents. It can catch issues early, improving the overall API quality. By integrating Spectral into your development workflow, you can automate the validation process and reduce the risk of deploying flawed APIs.
Setting Up Spectral in TypeScript
To use Spectral in a TypeScript project, you need to install it first. You can do this using npm or yarn. Here’s how to get started:
npm install -g @stoplight/spectral-cli
After installation, you can create a Spectral configuration file. This file defines the rulesets that Spectral will use during validation. You can extend existing rules or create custom ones to suit your project’s specific needs.
Here’s a simple example of how you might configure Spectral:
{ "extends": ["spectral:oas", "spectral:asyncapi"], "rules": { "info-contact": "warn", "info-description": "off" } }
Integrating Spectral in Your Workflow
Once configured, integrate Spectral into your development process. You can run Spectral as part of your CI/CD pipeline to ensure every change to your API specs is validated. This practice helps maintain consistent and error-free APIs throughout the development lifecycle.
Use Spectral via the command line to validate your API specifications:
spectral lint openapi.yaml
Spectral’s detailed feedback will guide you in addressing any issues, ensuring your APIs are robust and reliable. By incorporating Spectral into your toolkit, you empower your team to build better APIs with confidence, fostering a culture of quality and precision in API development.
Common Issues and Troubleshooting
When using Spectral in TypeScript, developers often encounter a few common issues. Troubleshooting these effectively can save time and reduce frustration. Here’s a guide to help you navigate these challenges.
1. Type Definitions Conflict
Type conflicts can arise when Spectral is used alongside other libraries. Ensure you have the correct type definitions installed for Spectral.
- Solution: Use TypeScript’s “skipLibCheck” option to bypass type checking for all declaration files.
2. Configuration Errors
Improper configuration is a frequent issue. Spectral relies on a configuration file to define rules.
- Solution: Validate your
.spectral.yml
file syntax, ensuring all rules are correctly defined.
3. Inconsistent Rule Execution
Sometimes, rules do not execute as expected. This can be due to rule specificity or conflicts.
- Solution: Check rule priorities and make sure no rules are overriding each other.
4. Performance Bottlenecks
Large files or complex rules can lead to performance issues.
- Solution: Optimize by breaking down large files or simplifying rules. Use Spectral’s built-in performance profiling to identify slow parts.
5. Debugging Rule Logic
Debugging custom rules can be challenging without the right tools.
- Solution: Use console logging within rules to monitor execution flow.
Example Code for Troubleshooting
// Example of setting the skipLibCheck in tsconfig.json { "compilerOptions": { "skipLibCheck": true } }
By understanding these common issues and applying the troubleshooting steps, you can effectively use Spectral within your TypeScript projects, enhancing code quality and consistency.
Real-World Use Cases of Spectral
In the fast-paced world of software development, ensuring the quality of APIs is crucial. Spectral, an open-source JSON/YAML linter, can be a game-changer for developers. Let’s dive into some real-world applications where Spectral shines.
1. API Contract Validation
API contracts are the backbone of any web service. Validating these contracts can prevent miscommunications between teams. With Spectral, you can automate this process. Define custom rules to check for consistency and adherence to standards.
// Example rule for checking API version format { "rules": { "api-version": { "description": "API version must follow semantic versioning", "given": "$..info.version", "then": { "function": "pattern", "functionOptions": { "match": "^[0-9]+\\.[0-9]+\\.[0-9]+$" } } } } }
2. Continuous Integration and Deployment (CI/CD)
Incorporate Spectral into your CI/CD pipelines. This ensures that every API change is validated before deployment. It reduces the risk of introducing breaking changes. Integrating Spectral in your build process can be seamless with the right setup.
// Example CI/CD integration "scripts": { "lint:api": "spectral lint api-spec.yaml" }
3. Documentation Consistency
Documentation is vital for API users. Spectral can enforce consistent documentation formats. This ensures that developers get the information they need without confusion. Define rules for required fields and format checks.
// Example rule for documentation consistency { "rules": { "operation-description": { "description": "Operations must have a non-empty description", "given": "$..paths[*][*]", "then": { "field": "description", "function": "truthy" } } } }
Using Spectral in these scenarios not only enhances API quality but also streamlines development workflows. By automating checks, developers can focus more on building features and less on manual validation.
Unlocking the Power of Spectral in Your TypeScript Projects
In the world of TypeScript development, ensuring code quality is paramount. That’s where Spectral comes in. This powerful tool acts as a linchpin in maintaining API standards, catching errors, and enforcing best practices. It’s not just about finding bugs; it’s about improving the overall structure of your code.
Spectral integrates seamlessly with TypeScript, offering a streamlined approach to linting. By leveraging Spectral, developers can automate the validation of their OpenAPI documents, ensuring they adhere to predefined rules. This automation reduces manual checks, saving time and minimizing errors.
Moreover, Spectral’s flexibility is unmatched. It allows developers to create custom rules specific to their project’s needs. This customization ensures that your code not only meets industry standards but also aligns with your team’s unique requirements. As projects grow, maintaining consistency becomes challenging, but Spectral simplifies this process.
Implementing Spectral in your TypeScript projects enhances collaboration. With clear guidelines and automated checks, teams can focus more on innovation and less on nitpicking code details. It fosters a culture of quality, where every developer knows what is expected, reducing the friction that often arises in large teams.
Finally, the benefits of using Spectral extend beyond immediate code quality. It contributes to a sustainable codebase, making future development smoother. By catching potential issues early, you ensure that your projects are scalable and maintainable in the long run. Spectral is not just a tool; it’s a strategic investment in your project’s success.
Previous
What is HOC in React?
Next