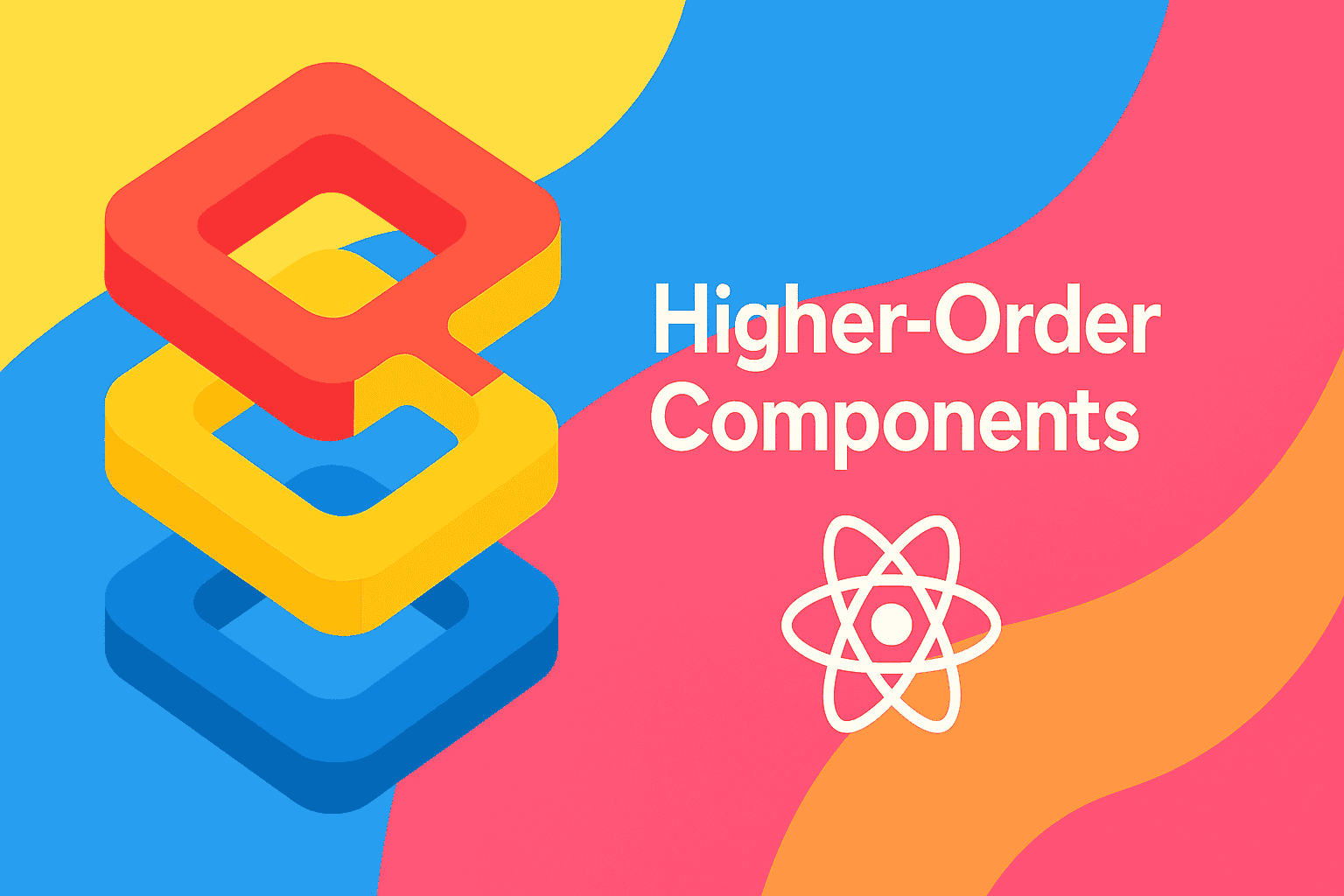
Fast Answers
Understanding Higher-Order Components in React
Higher-Order Components (HOCs) are a powerful pattern in React. They allow developers to enhance the functionality of components. By wrapping a component, an HOC can add extra props or logic.
One of the main benefits of HOCs is code reuse. Instead of duplicating logic, you can create an HOC to share it across components. This makes your codebase more manageable and easier to maintain.
HOCs work by accepting a component as an argument and returning a new component. This new component can modify the behavior of the original one. For example, you can create an HOC for data fetching. It could handle API calls and pass the data down as props.
However, be careful with HOCs. They can lead to a complex component tree if overused. Always ensure that your HOCs are clear and focused on a single responsibility.
To implement an HOC, start by defining a function that takes a component as its parameter. Inside this function, you can add any logic you need. Finally, return a new component that renders the original one.
Using HOCs can make your code more modular and testable. They provide a clean way to separate concerns in your application. As you build more complex React apps, understanding HOCs becomes essential.
How HOCs Enhance React Components
Higher-Order Components (HOCs) are a powerful tool in React for reusing component logic. They allow developers to abstract shared functionalities into a single unit, enhancing component efficiency and maintainability. By wrapping a component in an HOC, we can augment its behavior without modifying the component itself.
HOCs offer the advantage of code reuse. Imagine you have multiple components that need to fetch data from an API. Instead of implementing the data-fetching logic in each component, you can create a single HOC to manage this task. This approach reduces redundancy and streamlines updates across your application.
Moreover, HOCs enable separation of concerns by isolating logic from the UI. This separation helps maintain a clean and readable codebase. For example, you can have an HOC handle authentication checks, allowing the main component to focus solely on rendering UI elements.
Here’s a simple code example demonstrating an HOC in action:
// HOC to add logging functionality function withLogging(WrappedComponent) { return class extends React.Component { componentDidMount() { console.log('Component mounted:', WrappedComponent.name); } render() { return; } }; } // Example usage const EnhancedComponent = withLogging(MyComponent);
In this example, the HOC withLogging
adds logging functionality to any component it wraps. This pattern is not only efficient but also keeps your components focused on their core responsibilities.
In conclusion, HOCs are a valuable pattern for enhancing React components. They provide a clean way to share logic, promote code reusability, and maintain a modular architecture.
The Core Principles of HOCs
Higher-Order Components (HOCs) in React are an essential pattern for reusing component logic. They are a technique in which you create a function that takes a component and returns a new component. This function is what we call a Higher-Order Component.
One of the core principles of HOCs is that they do not modify the original component. Instead, they create a new wrapper component that renders the original component and enhances it with additional props or behavior.
Another important aspect is the ability to abstract shared logic. By using HOCs, developers can extract common functionalities from multiple components into a single, reusable piece of code. This promotes the DRY (Don’t Repeat Yourself) principle.
HOCs are also excellent for cross-cutting concerns, like logging, access control, and caching. They allow you to encapsulate these behaviors once and apply them to any component without altering the component’s code.
Here’s a simple example of a Higher-Order Component:
const withLogging = (WrappedComponent) => { return class extends React.Component { componentDidMount() { console.log('Component Mounted'); } render() { return; } }; };
In this example, the withLogging
HOC adds logging functionality to any component it wraps, without modifying the original component’s logic.
Implementing HOCs: A Step-by-Step Guide
Higher-Order Components (HOCs) in React are a powerful pattern that allows developers to reuse component logic. This guide will walk you through the process of implementing HOCs in a React application.
Step 1: Understand the Purpose of an HOC
An HOC is a function that takes a component and returns a new component. It’s often used for cross-cutting concerns like logging, authentication, or data fetching.
Step 2: Create the HOC Function
Start by defining a function that accepts a component as an argument.
const withExtraInfo = (WrappedComponent) => { return (props) => { return; }; };
Step 3: Use the HOC
Wrap your existing component with the HOC to enhance its functionality.
const BasicComponent = (props) => { return{props.extraInfo}; }; const EnhancedComponent = withExtraInfo(BasicComponent);
Benefits of Using HOCs
- Code Reusability: Share logic between components without repeating code.
- Separation of Concerns: Keep components focused on their primary function.
- Enhanced Readability: Simplify complex components by breaking them into smaller, reusable functions.
Final Thoughts on HOCs
Implementing HOCs can greatly improve your codebase. They provide a clean and efficient way to share functionality across components. By using this pattern, you can enhance the scalability and maintainability of your React applications.
Comparing HOCs to Other Patterns
In the React ecosystem, developers often encounter various patterns to enhance component functionality. One such pattern is the Higher-Order Component (HOC). But how does it stack up against other patterns like Render Props, Hooks, or Context API?
Higher-Order Components are functions that take a component and return a new component. This pattern is excellent for code reuse and adding functionality without modifying existing components.
Advantages of HOCs Over Other Patterns
- Code Reusability: HOCs allow for the reuse of component logic, making your codebase more maintainable.
- Separation of Concerns: They help in separating component logic from the UI, leading to cleaner code.
- Enhanced Readability: With HOCs, your main component logic remains uncluttered.
Code Example
const withLogging = (WrappedComponent) => { return class extends React.Component { componentDidMount() { console.log('Component Mounted'); } render() { return; } }; };
Comparison with Render Props
Render Props offer a flexible way to share code between components. However, they can lead to deeply nested components, making the code harder to read. HOCs, in contrast, keep the component tree shallower.
HOCs vs Hooks
With the introduction of Hooks, many developers shifted towards using them due to their simplicity and direct state management. However, HOCs still hold value when you need to wrap components with additional functionality without altering their structure.
HOCs vs Context API
Context API is perfect for passing data deeply through a component tree without prop drilling. But, when it comes to adding behavior, HOCs provide a more structured approach.
Common Use Cases for Higher-Order Components
Higher-Order Components (HOCs) are an advanced technique in React for reusing component logic. They offer a flexible way to enhance components with additional behavior or data. Let’s explore some common use cases for HOCs that developers often find beneficial.
Code Reusability
One of the primary advantages of HOCs is code reusability. By wrapping components with an HOC, you can share common functionality across multiple components without duplicating code. For instance, an HOC can manage authentication logic and apply it to different parts of an application.
State Management
HOCs are excellent for managing state that needs to be shared across several components. Instead of lifting state up and passing it down through props, you can use an HOC to encapsulate and distribute state efficiently.
Conditional Rendering
Conditional rendering often involves repetitive logic. HOCs can encapsulate this logic, allowing components to focus solely on their rendering logic. For example, you can use an HOC to render loading spinners or error messages based on network requests.
Logging and Analytics
If you need to log certain user interactions or track analytics, HOCs can help. They can wrap components and handle logging without altering the components’ internal logic. This separation of concerns makes the codebase cleaner and more maintainable.
Example of a Simple HOC
Here’s a basic example of how you might create an HOC to add additional props:
const withExtraProps = (WrappedComponent) => { return (props) => { const extraProps = { additionalData: "Some extra data" }; return; }; };
Performance Considerations with HOCs
Higher-Order Components (HOCs) are a powerful tool in React for reusing component logic. However, they can introduce performance issues if not used carefully.
One key concern is that HOCs can lead to unnecessary re-renders. Since HOCs wrap components, each update to the HOC can trigger a re-render of the wrapped component. This can degrade performance, especially if the HOC is applied to multiple components.
Another consideration is the potential for increased component tree depth. HOCs add an additional layer to the component tree, which can make debugging more complex and potentially slow down rendering. It’s important to be mindful of how many layers of HOCs are applied to a component.
To mitigate these issues, developers can use memoization or shouldComponentUpdate to optimize rendering. Memoization helps by caching results of expensive calculations, while shouldComponentUpdate can prevent unnecessary re-renders.
// Example of using React.memo with HOC const EnhancedComponent = React.memo(HOC(WrappedComponent));
Using React.memo alongside HOCs can prevent re-renders when props haven’t changed, enhancing performance. Additionally, developers should be cautious with the number of HOCs used in a single component tree.
In conclusion, while HOCs are beneficial for reusing logic, developers should be aware of the performance implications. By using best practices such as memoization and limiting component tree depth, performance can be optimized effectively.
Best Practices for Writing HOCs
Higher-Order Components (HOCs) are a powerful pattern in React. They allow for code reusability by transforming components. To write effective HOCs, follow some best practices. These practices ensure your HOCs are efficient, maintainable, and easy to understand.
Firstly, keep your HOCs simple. Aim for single responsibility. Each HOC should handle one concern. This makes them easier to test and debug. Also, ensure that they are reusable and can be applied to multiple components without modification.
Secondly, avoid side effects in your HOCs. They should be pure and predictable. This means no direct DOM manipulation or network requests inside them. Instead, handle such logic within the wrapped component or in a separate utility.
Thirdly, be mindful of props. Pass down all props to the wrapped component. This ensures the component receives everything it needs. Use the spread operator for this task:
const withEnhancement = (WrappedComponent) => { return (props) => { // Enhance or modify props as needed return; }; };
Furthermore, provide meaningful display names. This aids in debugging and development. Set the display name of your HOC to reflect both the HOC and the component it wraps:
const enhance = (WrappedComponent) => { const EnhancedComponent = (props) =>; EnhancedComponent.displayName = `enhance(${getDisplayName(WrappedComponent)})`; return EnhancedComponent; }; function getDisplayName(WrappedComponent) { return WrappedComponent.displayName || WrappedComponent.name || 'Component'; }
Lastly, document your HOCs well. Include comments and usage examples. This will help other developers understand and use them effectively.
Potential Pitfalls and How to Avoid Them
Higher-Order Components (HOCs) in React can greatly enhance code reusability. However, developers may run into pitfalls if not implemented carefully. Understanding these potential issues can save you time and frustration.
Performance Issues
One common problem is performance degradation. HOCs can introduce additional layers of abstraction, which might slow down rendering. To avoid this, ensure that you use React’s memo
function to prevent unnecessary re-renders.
State Management
Another pitfall is the complexity of state management. HOCs might cause confusion about which state belongs where. Avoid this by clearly defining state responsibilities and using hooks like useState
and useReducer
for local state management.
Debugging Challenges
Debugging can become difficult with HOCs. Since they wrap components, it can be challenging to trace issues back to the source. To mitigate this, use descriptive names for HOCs and take advantage of React Developer Tools.
Code Example: Applying HOCs
const withLogging = (WrappedComponent) => { return (props) => { console.log('Component is rendering'); return; }; };
In this example, the withLogging
HOC adds logging functionality. Use such patterns wisely to maintain code clarity and efficiency.
Future Trends in React and HOCs
As the JavaScript ecosystem evolves, so do the patterns we use in React. High-Order Components (HOCs) are no exception. They have played a significant role in enhancing component logic and reusability. However, the future of React might see a shift in how we utilize these patterns.
With the introduction of React Hooks, developers have found a more intuitive way to manage state and side effects. Hooks allow for better separation of concerns and cleaner code. This shift has led many to question the continued relevance of HOCs.
Nonetheless, HOCs still have their place in the React landscape. They offer a powerful way to inject logic into components. They are particularly useful in scenarios where component logic needs to be shared across multiple components. Yet, the trend leans towards combining HOCs and Hooks for optimized performance and readability.
In the future, we may see HOCs being used in more specific cases. For instance, they could be ideal for implementing cross-cutting concerns like analytics or error handling. Meanwhile, Hooks will likely handle state management and side effects.
Furthermore, the React team continues to innovate. They introduce new patterns and APIs that might redefine how we think about HOCs. Developers should stay updated with these changes. Understanding these trends ensures that we use the best tools for our projects.
Previous
How to Optimize React Router for Fast Loading
Next