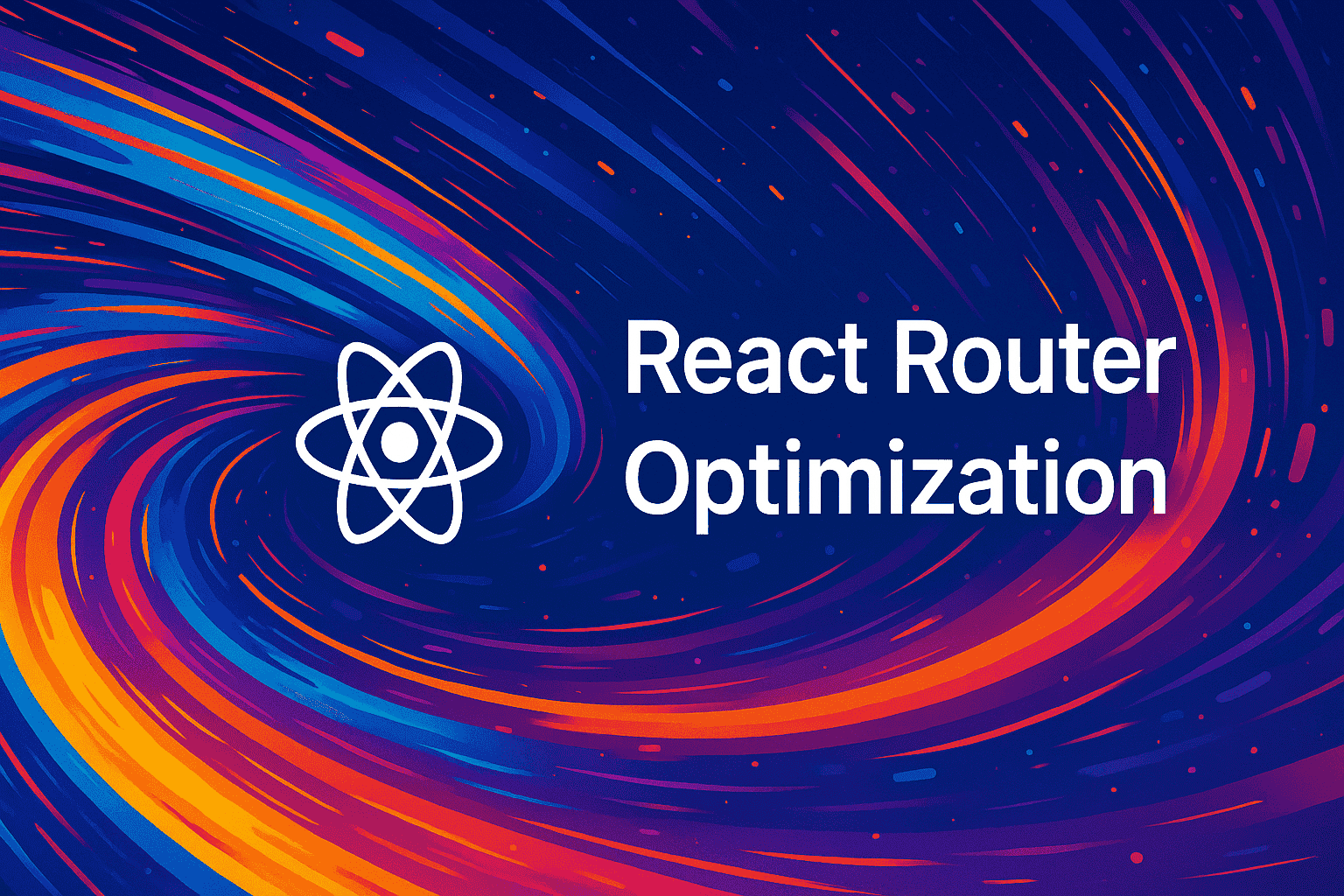
Fast Answers
Introduction to React Router Optimization
React Router is essential for creating seamless navigation in Single Page Applications (SPAs). However, optimizing it can significantly enhance load times. This is crucial for developers aiming to improve user experience and performance.
Optimizing React Router involves several strategies. First, consider code splitting. By using dynamic imports, you can load routes only when needed. This reduces the initial load time since less code is downloaded upfront.
Another technique is lazy loading components. React’s built-in React.lazy
function, combined with Suspense
, allows components to load only when they are rendered. This ensures that users only download the code necessary for their current view.
Furthermore, enhancing caching strategies can dramatically improve performance. Utilizing the browser’s caching capabilities, or implementing service workers, helps in reducing load times for repeat visitors.
Additionally, consider reducing the number of routes if possible. Each route adds complexity and overhead, which can slow down the application. Streamlining routes ensures faster navigation and responsiveness.
Finally, always monitor and profile your application. Tools like React DevTools and Lighthouse can identify bottlenecks and suggest improvements. Regular monitoring helps in maintaining optimal performance as the application grows.
Incorporating these strategies not only optimizes React Router but also aligns with Google’s algorithm, which prioritizes fast and responsive websites. Developers should continuously strive for efficient routing to enhance both user experience and SEO.
Best Practices for Improving Load Times
In the fast-paced world of web development, optimizing load times is crucial. A slow-loading site can lead to frustrated users and reduced engagement. To enhance performance, consider these best practices when working with React Router.
Code Splitting with React Router
Code splitting is a powerful optimization technique. By breaking your application into smaller chunks, you can load components only when needed. This reduces the initial load time significantly.
import { lazy, Suspense } from 'react'; const Home = lazy(() => import('./Home')); const About = lazy(() => import('./About')); function App() { return (Loading...}> ); }
Utilize Browser Caching
Leverage browser caching to store static resources. This reduces the need to reload resources, decreasing overall load times. Set appropriate cache headers on your server to enable this feature.
Minify and Compress Files
Minifying JavaScript and CSS files reduces their size. Use tools like Terser for JavaScript and CSSNano for CSS. Additionally, enable GZIP compression on your server to further improve performance.
Optimize Images
Images can be a significant part of load times. Use tools like ImageOptim or TinyPNG to compress images without losing quality. Also, consider serving images in next-gen formats like WebP.
Implement Prefetching and Preloading
Prefetching and preloading resources can improve perceived load times. Use the tag to prefetch resources that are likely to be used soon.
Implementing Code Splitting in React
Code splitting is a powerful optimization technique to enhance your React application’s performance. It enables you to break down your app into smaller, manageable chunks. By loading these chunks on demand, you significantly reduce the initial load time. This not only improves the user experience but also aligns with Google’s page speed algorithms.
React’s lazy and Suspense are tools that make code splitting straightforward. Lazy loading allows you to import components only when needed. As a result, the browser downloads only the necessary code, reducing the bundle size.
How to Implement Code Splitting with React Router
Integrating code splitting with React Router involves a few simple steps. Start by importing React.lazy
to dynamically load components. Then, wrap these components with React.Suspense
to handle the loading state.
const Home = React.lazy(() => import('./Home')); const About = React.lazy(() => import('./About')); function App() { return (); } Loading...}>
In this example, components Home
and About
are loaded only when their routes are accessed. The Suspense
component provides a fallback UI during the loading phase, ensuring a seamless experience.
By implementing code splitting, you optimize your React Router setup. This approach not only decreases initial load times but also improves navigational efficiency. Users experience faster content delivery, keeping them engaged while navigating through your application.
Lazy Loading Routes for Performance Boost
When optimizing React Router for fast loading times, lazy loading routes can be a game-changer. As developers, we strive to create seamless user experiences. One effective way to accomplish this is by implementing lazy loading, which can significantly enhance performance.
Lazy loading allows you to load components only when they are needed. By doing so, the initial bundle size decreases, leading to faster page loads. This method aligns well with Google’s algorithm, which favors faster loading sites. As a result, implementing lazy loading can improve your search engine ranking and user retention.
Implementing Lazy Loading in React Router
To implement lazy loading, you can use React’s React.lazy
and Suspense
components. These tools allow you to split your code at logical points, loading only what’s necessary for the current view. Here’s a quick example:
const HomeComponent = React.lazy(() => import('./HomeComponent')); function App() { return (); } Loading...}>
In the code above, the HomeComponent
only loads when the user navigates to the root path. This approach reduces the initial load time, providing a smoother experience.
Benefits of Lazy Loading
- Reduces initial load time, enhancing the user experience.
- Improves SEO by aligning with Google’s focus on speed.
- Decreases bandwidth usage, which is beneficial for users on mobile networks.
With lazy loading, you can optimize React Router effectively. By focusing on performance, you create applications that not only engage users but also perform well in search rankings. Embrace lazy loading and see the difference in your application’s speed and responsiveness.
Utilizing React’s Suspense for Smooth Transitions
React’s Suspense is a powerful tool for managing asynchronous operations. It enhances user experience by creating smoother transitions between pages. When paired with React Router, it ensures components load gracefully without abrupt interruptions.
Implementing Suspense in your React applications can significantly reduce loading times. It allows you to define fallback UI for components that are still fetching data. This approach lets users interact with the application while content loads in the background.
To leverage Suspense effectively, wrap your lazy-loaded components. This ensures that while data is being fetched, users see a placeholder. Such seamless transitions keep users engaged and reduce bounce rates.
Here are some steps to implement React Suspense:
- Use
React.lazy()
to dynamically import components. - Wrap lazy components with
<Suspense>
and provide a fallback UI. - Combine Suspense with
React Router
to manage route-based code splitting. - Ensure fallback components are minimal for quick loading.
By utilizing React’s Suspense, developers can create applications that feel faster and more responsive. It reduces perceived loading times and keeps users focused on the content.
Leveraging Browser Caching Strategies
For developers optimizing React Router, browser caching is crucial. It significantly reduces loading time by storing static assets locally. When users revisit a page, the browser doesn’t need to reload unchanged resources. This reduces server load and speeds up page rendering.
To implement this, configure your server to send appropriate cache-control headers. These headers instruct the browser on how long to cache resources. Here’s a basic example using an Express server:
app.use(express.static('public', { maxAge: '1y', // Cache for one year etag: false }));
Using maxAge
ensures the browser caches files for a specified period. Disabling etag
avoids unnecessary validation with the server. This approach is beneficial for assets like images, stylesheets, and scripts that rarely change.
Additionally, consider implementing service workers for enhanced caching capabilities. Service workers act as a proxy between your app and the network. They can pre-cache important files, ensuring your app loads even when offline. Here’s a simple service worker registration:
if ('serviceWorker' in navigator) { navigator.serviceWorker.register('/sw.js').then(function(register) { console.log('Service Worker registered with scope:', register.scope); }).catch(function(error) { console.error('Service Worker registration failed:', error); }); }
By integrating browser caching strategies and service workers, you optimize React Router. This results in faster loading times, improving user experience and potentially boosting SEO rankings. Remember, a fast website keeps users engaged and satisfied.
Optimizing Bundle Sizes with Tree Shaking
Developers are always on the lookout for ways to optimize their applications. One effective technique is tree shaking, which can significantly reduce your bundle size. By shaking off the unused parts of your JavaScript code, you enhance the loading speed of your React applications.
Tree shaking is a form of dead code elimination. It analyzes your code to detect and remove unused imports. This results in smaller bundle sizes, leading to faster loading times and improved performance.
To implement tree shaking in your project, ensure that your module bundler, like Webpack, is properly configured. Webpack 2 and later versions have built-in support for tree shaking. Configure the bundler to use ES6 module syntax, as tree shaking relies on static analysis.
// Example: Importing only the necessary components import { BrowserRouter, Route } from 'react-router-dom';
In the example above, only the necessary components from ‘react-router-dom’ are imported. This helps to avoid bundling the entire library, which can be quite large.
Additionally, consider using tools like webpack-bundle-analyzer to visualize your bundle content. This helps you identify areas for further optimization.
- Ensure your dependencies are updated.
- Use ES6 imports and exports.
- Regularly analyze your bundle size.
By adopting these practices, you ensure that your React applications are not only fast and efficient but also maintainable in the long run. Implementing tree shaking is a small step with a significant impact on performance.
Monitoring and Analyzing Route Performance
As developers, ensuring fast loading times is crucial for a smooth user experience in React applications. To optimize React Router, monitoring and analyzing route performance is essential. This involves keeping an eye on how routes load and interact with different components.
Firstly, consider using the browser’s developer tools to monitor network activity. By examining network requests, you can identify which resources take the longest to load. This information is valuable for pinpointing bottlenecks in your routes.
Additionally, employing performance monitoring libraries like Lighthouse or WebPageTest can provide deeper insights. These tools evaluate the speed and efficiency of your routes and offer actionable suggestions to enhance performance.
Implementing lazy loading is another effective strategy. It ensures that only the necessary components are loaded when a route is accessed, reducing initial load times. React’s React.lazy()
function can be a game-changer in this regard.
Finally, regularly analyzing user behavior through logs or analytics can reveal patterns in route usage. Understanding which routes are most frequently accessed can help prioritize optimization efforts.
By consistently monitoring and analyzing route performance, developers can make informed decisions to optimize React Router, ensuring a faster and more efficient application.
Conclusion: Bringing It All Together for Faster Load Times
Optimizing React Router can significantly enhance your app’s performance. By implementing code splitting, you ensure only the necessary components load initially. This approach reduces the initial bundle size, speeding up load times.
Server-side rendering can further improve performance. It allows the server to generate HTML content, which then gets sent to the client. This method ensures faster rendering and better SEO rankings.
Additionally, consider lazy loading images and components. Lazy loading defers the loading of non-essential resources, enhancing the user experience by prioritizing visible content. This technique is crucial for apps with heavy media content.
Lastly, leverage browser caching. By instructing browsers to store certain resources, subsequent page loads become quicker. Cached resources load from the user’s device, reducing server requests.
Integrating these strategies will create a seamless and fast user experience. As developers, focusing on load times and performance is essential. Happy optimizing!
Previous
How to Optimize React Re-Rendering for Better Performance
Next