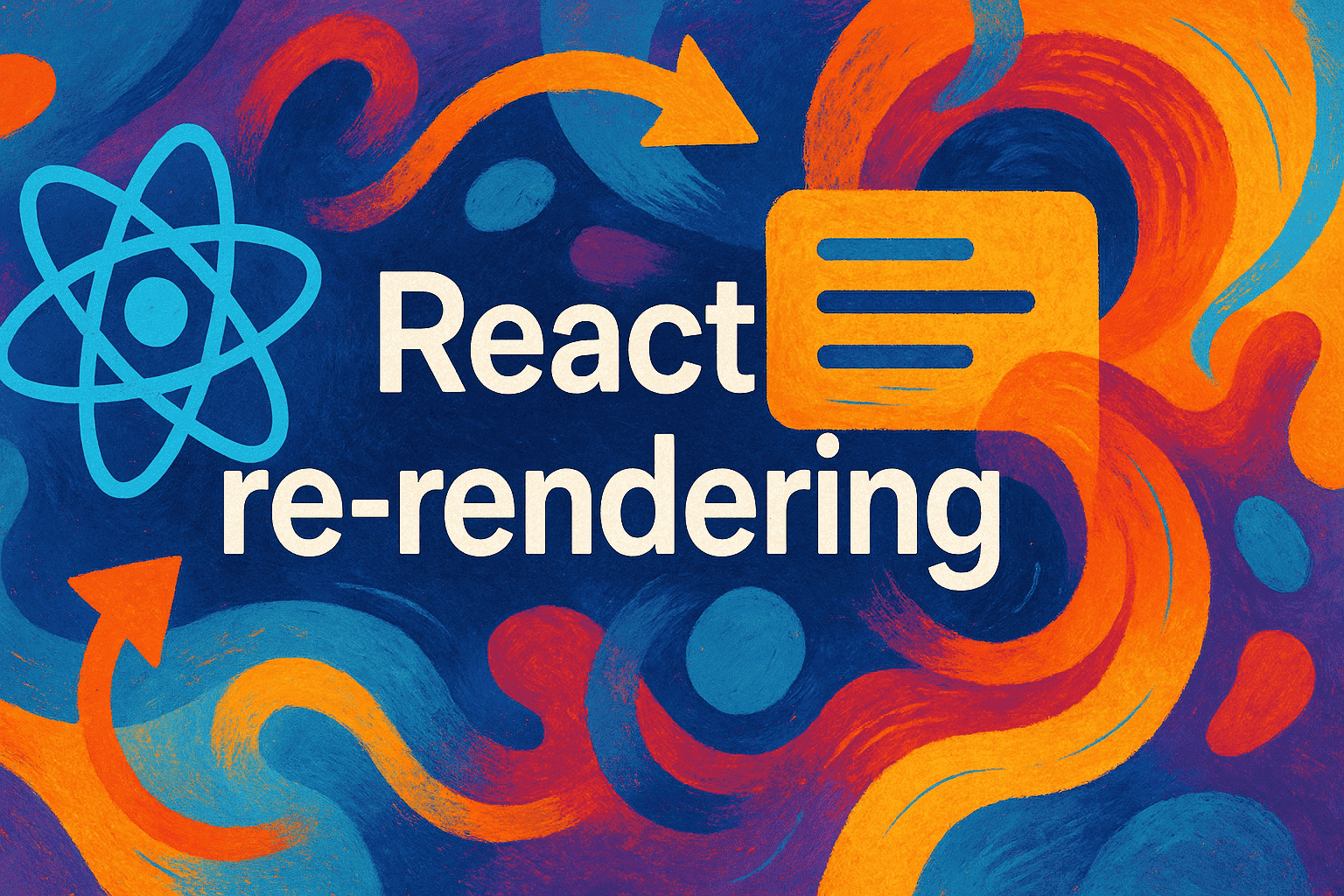
Fast Answers
Introduction to React Re-Rendering
React is a powerful JavaScript library for building user interfaces. It provides an efficient way to update the UI in response to changes in application state. However, React components can re-render frequently, which might impact performance. Understanding React’s re-rendering behavior is crucial for developers aiming to optimize their applications.
React re-renders components when their state or props change. This ensures that the UI reflects the latest data. Yet, unnecessary re-renders can occur, wasting resources. Recognizing when these render cycles happen helps developers optimize their code effectively.
One key aspect of React’s re-rendering is the virtual DOM. React uses this virtual representation to efficiently update the real DOM. When a component’s state changes, React compares the virtual DOM with the previous one. It then updates only the parts that have changed, minimizing performance costs.
Developers should also be aware of how React handles component updates. Parent component updates can trigger re-renders in child components. To prevent unnecessary re-renders, developers can use techniques like memoization. Using React.memo or useMemo can help control when a component should update, thus improving performance.
Understanding React’s lifecycle methods is also beneficial. Methods such as shouldComponentUpdate provide more control over re-renders. By leveraging these methods, developers can ensure that components only re-render when necessary, optimizing their application’s performance.
In summary, understanding React’s re-rendering process is essential for performance optimization. By identifying when and why components re-render, developers can make informed decisions to enhance their applications. Techniques like memoization, lifecycle methods, and virtual DOM diffing are valuable tools in this optimization journey.
Understanding the React Rendering Process
As developers, understanding how React’s rendering process works is crucial for optimizing your application’s performance. React’s rendering is based on a virtual DOM, which efficiently updates only the parts of the DOM that have changed. However, unnecessary re-rendering can still occur, impacting performance.
React components re-render when state or props change. But not all changes need to trigger re-renders. Knowing when and why a component re-renders helps in making informed decisions about optimization. React uses a reconciliation process to determine the minimal number of updates needed, but developers can further optimize this process.
To optimize React re-rendering, consider the following tips:
- Utilize
React.memo
to prevent unnecessary re-rendering of functional components. - Implement
shouldComponentUpdate
in class components to control updates. - Use the
useCallback
anduseMemo
hooks to memoize functions and values. - Leverage the key prop effectively for dynamic lists to maintain identity across renders.
- Profile your application using React Developer Tools to identify components that re-render unnecessarily.
Transitioning from understanding to action can significantly enhance your React app’s performance. Thus, mastering React’s rendering process is a vital skill for developers seeking to build efficient web applications.
Identifying Performance Bottlenecks
As developers, optimizing React applications is crucial for a seamless user experience. Identifying performance bottlenecks is the first step toward achieving this goal. Here’s how you can spot them effectively.
Use React’s built-in tools to analyze component render times. React DevTools is indispensable for visualizing component hierarchies and render durations. These insights help pinpoint components that render more often than necessary.
Keep an eye on your network requests. Slow network responses can lead to delayed component updates. Use Chrome DevTools to monitor network activity and ensure you’re not fetching data more than needed.
Examine your application for unnecessary re-renders. Use tools like why-did-you-render to detect avoidable re-renders. This tool alerts you when components render with the same props or state.
Profiling with the Performance tab in Chrome DevTools is another technique. It helps track JavaScript execution time and identify long-running tasks. This information is essential for optimizing your code.
Consider the following checklist to identify bottlenecks effectively:
- Use React DevTools for render insights.
- Monitor network requests and responses.
- Leverage why-did-you-render to catch unnecessary re-renders.
- Profile with Chrome DevTools’ Performance tab.
By focusing on these areas, you can make your React applications faster and more efficient. Understanding and addressing performance bottlenecks is key to delivering a superior user experience.
Using React.memo for Component Optimization
As a developer, you often face performance bottlenecks caused by unnecessary re-renders in React applications. React.memo is a powerful tool in your optimization toolkit. It helps you prevent these unnecessary updates, thus boosting performance.
React.memo is a higher-order component (HOC) that memoizes functional components. It caches the result of a render and reuses it when the component receives the same props. This prevents React from re-rendering a component unnecessarily.
Consider the following situation: you have a parent component that passes props down to a child component. If the parent re-renders due to state changes, the child will also re-render, even if its props remain unchanged. This is where React.memo comes into play.
const MyComponent = React.memo(function MyComponent({ value }) { console.log('Rendering MyComponent'); return{value}; });
In this code example, MyComponent
will only re-render if its value
prop changes. If the parent component re-renders with the same value
, MyComponent
will skip rendering. This reduces unnecessary operations and improves performance.
It’s important to note that React.memo performs a shallow comparison of props by default. If your component receives complex objects or functions, consider providing a custom comparison function to React.memo for accurate control over re-rendering.
const areEqual = (prevProps, nextProps) => { return prevProps.value === nextProps.value; }; const MyComponent = React.memo(function MyComponent({ value }) { console.log('Rendering MyComponent'); return{value}; }, areEqual);
This approach gives you fine-tuned control over component updates, allowing you to manage complex scenarios more effectively. By using React.memo, you create faster, more efficient React applications that respond seamlessly to user interactions.
Implementing useCallback Hook for Better Performance
As developers, we often face performance challenges while building React applications. One of the key tools to enhance performance is the useCallback
hook. Understanding how to utilize this hook effectively can prevent unnecessary re-renders and optimize your application.
The useCallback
hook returns a memoized callback function. This means that the function is recreated only when its dependencies change, not on every render. This is particularly useful when passing callbacks to child components, as it ensures that the child component only re-renders when necessary.
Here’s a simple example to illustrate its usage:
import React, { useState, useCallback } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = useCallback(() => { setCount(c => c + 1); }, []); return (); }Count: {count}
In this example, the increment
function is memoized using useCallback
. This prevents its recreation on every render, thus avoiding unnecessary re-renders of the button component.
By using useCallback
, developers can ensure that their React applications perform efficiently. This small change can lead to significant performance improvements, especially in larger applications with many nested components.
Leveraging useMemo to Prevent Unnecessary Calculations
As developers, we constantly strive to make our React applications perform better. One way to achieve this is by leveraging the useMemo
hook. It helps prevent unnecessary calculations and enhances rendering performance.
In React, every time a component re-renders, it recalculates all the values within it. This might not be a big deal for simple calculations, but for complex ones, it could slow down the app. Here’s where useMemo
comes into play.
useMemo
allows you to memorize the result of a calculation. It only recalculates when its dependencies change. This means you can skip recalculations when they are not needed, saving precious rendering time.
Let’s look at a basic example of using useMemo
to optimize a React component:
const MyComponent = ({ numbers }) => { const expensiveCalculation = (numList) => { console.log('Calculating...'); return numList.reduce((acc, num) => acc + num, 0); }; const total = useMemo(() => expensiveCalculation(numbers), [numbers]); returnTotal: {total}; };
In this example, the function expensiveCalculation
is wrapped with useMemo
. This ensures it only runs when the numbers
array changes. This small tweak can significantly boost performance, especially for components dealing with large datasets or complex calculations.
By thoughtfully implementing useMemo
, developers can make React applications more efficient and responsive. It’s a tool that, when used correctly, can prevent costly re-renders and improve user experience.
Optimizing Context API for Reduced Re-Rendering
When working with React, the Context API is a popular tool to manage state globally. However, it can lead to unnecessary re-rendering if not used optimally. To enhance performance, it’s crucial to minimize these re-renders.
One effective approach is to split your context into smaller, more focused contexts. This way, components consume only the exact data they need, avoiding the ripple effect of re-rendering when unrelated data changes.
Another technique is memoizing context values. You can use the useMemo
hook to memoize the values provided by the context. This ensures that components only re-render when the actual data they depend on changes.
const MyProvider = ({ children }) => { const [state, setState] = useState(initialState); const value = useMemo(() => ({ state, setState }), [state]); return ({children} ); };
Additionally, consider using the React.memo
higher-order component for components consuming the context. This helps prevent re-renders unless the props truly change.
Lastly, evaluate if the components need to subscribe to the entire context. Sometimes, only specific parts of the state are relevant. Use custom hooks to select the data that each component needs.
By applying these strategies, you can significantly reduce unnecessary re-renders, leading to a more responsive and efficient React application.
Effective Use of React Profiler for Insights
React Profiler is a powerful tool that helps developers gain insights into their applications’ performance. By understanding the behavior of components during rendering, you can optimize your React app to minimize unnecessary re-renders.
When using React Profiler, focus on identifying components that render frequently. This helps you pinpoint performance bottlenecks. Start by accessing the Profiler in the React Developer Tools. You can then record interactions and analyze the “flame graph” and “ranked chart” views.
Look for components with long render times or those that re-render more often than necessary. These are your primary targets for optimization. Use the data from the Profiler to refactor components and improve performance.
Steps to Use React Profiler Effectively
- Open React Developer Tools and navigate to the Profiler tab.
- Click “Start Profiling” and interact with your application.
- After recording, stop profiling and analyze the charts.
- Identify components with high rendering times.
- Optimize by memoizing components or using React.memo.
React Profiler not only highlights inefficient components but also encourages better coding practices. Transitioning to optimized components ensures a smoother user experience. In turn, this can lead to more satisfied users and improved app performance.
Avoiding Common Pitfalls in React Rendering
React’s rendering process can be tricky. Developers often encounter unnecessary re-renders, which can affect performance. Understanding how React updates components is vital.
One common pitfall is not using keys properly in lists. Keys help React identify which items have changed, are added, or removed. Without unique keys, React may not render efficiently.
Another issue is binding functions inside render methods. This creates a new function on every render, causing child components to re-render unnecessarily. Instead, bind functions in the constructor or use class properties.
State management is crucial. Changing state inappropriately can lead to multiple re-renders. Use functional updates or libraries like Redux for better control over state changes.
Memoization can prevent unnecessary renders. Use React.memo for functional components and shouldComponentUpdate for class components. These tools allow React to skip rendering when props and states haven’t changed.
Also, beware of deeply nested components. Each change can cause a cascade of renders. Consider using Context API or component composition to manage data flow efficiently.
- Ensure each list item has a unique key.
- Avoid binding functions in render methods.
- Manage state changes efficiently.
- Use memoization wisely.
- Reduce nesting in component structures.
By understanding these pitfalls, developers can optimize React rendering. This leads to faster applications and smoother user experiences.
Conclusion on Optimizing React Re-Rendering
Optimizing React re-rendering is essential for building high-performance applications. By focusing on this aspect, developers can drastically improve the user experience. The key is identifying unnecessary re-renders and eliminating them effectively.
Utilizing tools like React Developer Tools can help in pinpointing components that re-render excessively. After identifying these components, one can strategize to optimize performance. Implementing memoization techniques like React.memo and useMemo can prevent unnecessary renders.
Furthermore, the useCallback hook is invaluable for optimizing functions passed as props. It ensures that functions are not recreated on every render, thus reducing overhead. Another tip is to manage state wisely by lifting state up or using state management libraries.
Don’t forget the importance of key props in lists. They provide React with a stable identity for each component, facilitating efficient updates. Also, lazy loading components and splitting code can drastically reduce the initial load time.
Remember to regularly profile your components. This helps in understanding the rendering behavior and identifying bottlenecks. Lastly, always keep an eye on the latest best practices and updates from the React community.
Previous