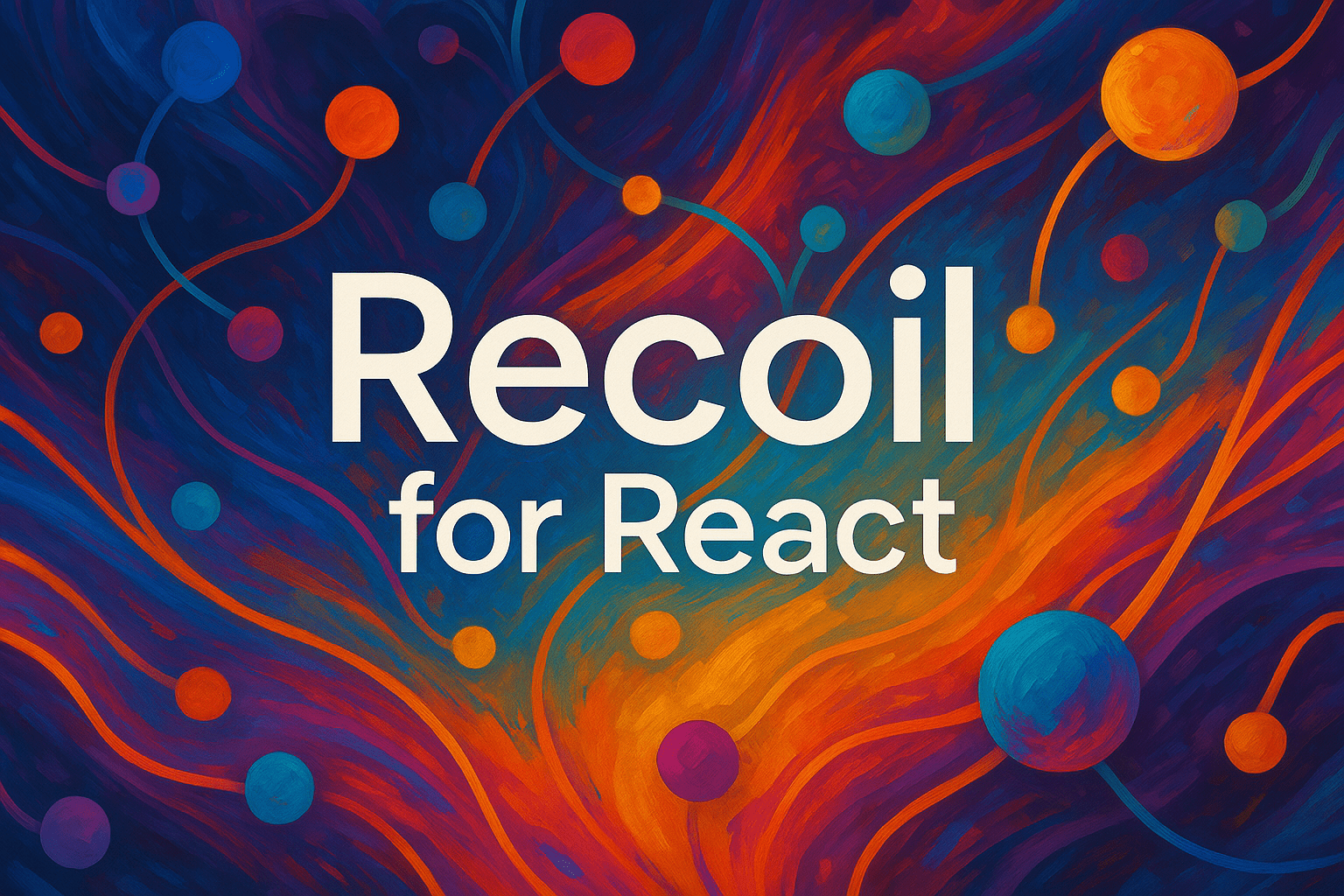
What is Recoil?
Recoil is a state management library specifically designed for React applications. It was developed by Facebook to address limitations found in other state management solutions. Recoil offers a fresh approach, making it easier to manage complex state logic.
Recoil allows you to share state across your application using atoms and selectors. Atoms are units of state that can be read and written from any component. Selectors are functions that compute derived state based on atoms or other selectors.
- Atoms: These are the building blocks of state in Recoil. They are like pieces of Lego, flexible and easy to use.
- Selectors: These enable you to create dynamic data transformations without altering the underlying atoms.
With Recoil, state becomes more predictable and easier to debug. It seamlessly integrates with React’s concurrent mode, providing a smooth user experience. Moreover, it supports asynchronous data fetching, allowing developers to manage remote data effortlessly.
In conclusion, Recoil provides a modern, efficient way to manage state in React applications. It simplifies state management while enhancing performance and scalability.
Benefits of Using Recoil
Recoil offers a fresh approach to state management in React applications. It provides simplicity and flexibility, making it a popular choice among developers. Let’s explore some of the key benefits of using Recoil.
- Minimal Boilerplate: Recoil requires less boilerplate code compared to other state management libraries. This reduces complexity and speeds up development.
- Scalable Architecture: Recoil’s atom-based structure allows for scalable state management. You can easily manage both small and large state trees.
- Concurrent Mode Support: Recoil integrates seamlessly with React’s Concurrent Mode. This enhances performance and improves user experience.
- Efficient Updates: Recoil optimizes state updates by only re-rendering components that depend on changed state. This boosts app performance.
- Flexible State Sharing: Recoil allows easy state sharing across components without prop drilling. This simplifies component interactions.
By leveraging these benefits, developers can build robust and performant React applications. Recoil’s intuitive API and powerful features make it a compelling choice for state management.
Recoil vs Other State Management Libraries
When it comes to state management in React, developers have a variety of libraries to choose from. Each has its own strengths and weaknesses. Recoil, a relatively new player, offers a fresh perspective on how to manage state effectively. Let’s explore how it stacks up against other popular libraries like Redux and MobX.
Why Consider Recoil?
Recoil is designed with simplicity and performance in mind. It provides an intuitive API that integrates seamlessly with React’s component architecture. This makes it easier for developers to manage complex state without unnecessary boilerplate.
Comparing Features
- Recoil integrates directly with React, offering a more native feel.
- Redux is known for its predictability and vast ecosystem.
- MobX shines with its reactivity and minimalistic approach.
Pros and Cons
- Pros:
- Recoil provides out-of-the-box support for concurrent mode.
- It allows for fine-grained state management and easy debugging.
- Cons:
- As a newer library, Recoil has a smaller community compared to Redux.
- It may lack some advanced features available in established libraries.
Use Cases
Recoil is ideal for projects where state management needs to be simple yet powerful. It’s perfect for applications that require a responsive UI and complex state interactions.
Ease of Integration with React
Recoil’s seamless integration with React makes it a preferred choice for developers. Its design aligns perfectly with React’s component-driven architecture. This allows for effortless state management without disrupting existing code structures.
One of the standout features of Recoil is its minimal API surface. This means developers can start using Recoil with minimal boilerplate. Here’s a quick example of how easy it is to integrate Recoil into a React application:
import { atom, useRecoilState } from 'recoil'; const textState = atom({ key: 'textState', default: '', }); function TextInput() { const [text, setText] = useRecoilState(textState); return ( setText(e.target.value)} /> ); }
In this example, you only need to import Recoil’s atom and useRecoilState hooks. This demonstrates how Recoil fits naturally into the React ecosystem. Furthermore, Recoil’s state can be shared across components, reducing the need for prop drilling.
Recoil’s ability to handle both local and global state makes it versatile. This flexibility is particularly beneficial in complex applications where state management can become challenging.
Moreover, Recoil supports asynchronous queries, which is crucial for modern web applications. This feature makes it easy to fetch data, keeping your state in sync with server responses.
With Recoil, you can focus more on building features rather than dealing with intricate state management. Its simplicity and power make it an excellent tool for React developers looking to streamline their workflow.
Performance Improvements with Recoil
Recoil offers a more efficient approach to state management in React applications.
It enhances performance by reducing the need for unnecessary re-renders and optimizing state updates.
Traditionally, managing state in React can lead to performance bottlenecks.
This is especially true when dealing with large applications.
However, Recoil introduces a mechanism called “atoms” and “selectors” which help in finely tuning your state updates.
Atoms are the units of state in Recoil.
They act as a shared state across components.
When an atom’s state changes, only the components subscribing to that atom re-render.
This targeted re-rendering significantly boosts performance.
Selectors, on the other hand, are pure functions that allow you to compute derived state.
They cache their results and only recompute when their dependencies change, preventing redundant calculations.
Why This Matters
- Minimized re-renders improve the user experience by making the interface more responsive.
- Reduced computational overhead by updating only necessary components.
- Scalable state management as your application grows.
By leveraging these features, developers can build high-performance, scalable applications.
Recoil’s architecture ensures that your React application runs smoothly, even as it becomes more complex.
Recoil’s Developer-Friendly API
In the ever-evolving world of React state management, Recoil stands out with its developer-friendly API. This characteristic is a major reason why many developers are choosing Recoil over other state management tools. With Recoil, you can handle complex state logic without the usual headaches.
Simplifying State Management
Recoil’s API allows developers to manage state in a way that feels intuitive. For instance, atoms and selectors are the fundamental building blocks of Recoil state. An atom represents a piece of state, while selectors can compute derived state. This clear distinction simplifies the process of managing and deriving state.
// Creating an atom import { atom } from 'recoil'; const textState = atom({ key: 'textState', // unique ID (with respect to other atoms/selectors) default: '', // default value (aka initial value) });
The above example shows how simple it is to create an atom in Recoil. The API is intuitive, enabling developers to quickly grasp how to define state. This ease of use is crucial for maintaining productivity in fast-paced development environments.
Seamless Integration
Recoil’s API integrates seamlessly with React, making it a natural choice for React developers. You can use hooks like useRecoilState
or useRecoilValue
to interact with your atoms and selectors just like you would with React’s built-in useState
or useEffect
hooks.
// Using useRecoilState hook import { useRecoilState } from 'recoil'; function TextInput() { const [text, setText] = useRecoilState(textState); const onChange = (event) => { setText(event.target.value); }; return ( ); }
This example demonstrates how Recoil hooks work similarly to React’s hooks. The consistency in API design reduces the learning curve for React developers, allowing them to integrate Recoil into their projects with minimal friction.
Scalability and Flexibility with Recoil
When building applications, scalability and flexibility are crucial. Recoil offers a robust solution for managing state in React, addressing these needs effectively. As your application grows, managing state can become complex. Recoil is designed to handle this complexity efficiently.
Recoil provides a flexible state management approach, allowing you to manage shared and local states seamlessly. It achieves this by using a graph structure to track state dependencies. This ensures that only the components affected by state changes are re-rendered, optimizing performance.
Recoil’s atom and selector architecture allows developers to easily scale their applications. Atoms are units of state that can be shared across components. This makes your state predictable and easy to manage. For example:
const textState = atom({ key: 'textState', default: '', });
As your application grows, you can add more atoms without affecting existing ones. This modularity ensures that your application remains maintainable and scalable.
Flexibility is also a significant advantage of Recoil. It integrates seamlessly with React’s concurrent mode, allowing developers to build applications that are responsive and performant. Recoil’s asynchronous selectors make it easy to manage asynchronous data fetching:
const asyncData = selector({ key: 'asyncData', get: async ({get}) => { const response = await fetch('https://api.example.com/data'); return response.json(); }, });
This flexibility allows developers to handle complex state management scenarios with ease, all while keeping the codebase clean and efficient.
Moreover, Recoil’s atom and selector concepts allow for granular control over state management. Atoms represent the smallest state units, while selectors are pure functions derived from one or more atoms. This structure supports scalability by enabling developers to break down state management into smaller, manageable parts.
Recoil’s flexibility is evident in its ability to integrate with concurrent React features. It works well with React’s Suspense and concurrent rendering, allowing developers to build highly responsive applications. This integration is crucial for modern web applications where user experience is a priority.
Community Support and Resources
When choosing a state management library like Recoil for your React applications, a vibrant community can be a game-changer. Developers benefit from a robust support network that provides answers, tools, and shared knowledge.
Recoil boasts an active community, making it easier to troubleshoot issues. You can find guidance and solutions on platforms like GitHub, Stack Overflow, and Discord. These resources are invaluable for both beginners and experienced developers.
Moreover, Recoil’s documentation is comprehensive and well-structured. It offers tutorials, examples, and explanations that cater to various learning styles. This makes diving into Recoil straightforward and less daunting.
- GitHub: Access the latest updates and contribute to the project.
- Stack Overflow: Find answers to common issues and ask questions.
- Discord: Engage in real-time discussions with fellow developers.
With these resources at your disposal, tackling complex state management problems becomes a collaborative effort. The community’s enthusiasm for Recoil fosters an environment of constant learning and innovation.
Conclusion
In the dynamic world of React development, managing state effectively is crucial. Recoil emerges as a powerful tool, addressing some of the common challenges developers face with state management. Its ability to handle complex state logic and provide enhanced performance makes it a compelling choice. By simplifying state sharing across components, Recoil streamlines development and boosts productivity.
Furthermore, Recoil’s seamless integration with React allows for a more intuitive development process. Its minimalistic syntax and efficient state management capabilities enable developers to focus more on building features and less on managing state intricacies. This can lead to faster development cycles and more maintainable codebases.
- Pros:
- Efficient state management with minimal boilerplate.
- Seamless integration with React ecosystem.
- Improves performance with fine-grained updates.
- Cons:
- Relatively new, with a smaller community compared to Redux.
- Learning curve for developers new to the paradigm.
Ultimately, choosing Recoil can lead to more efficient and scalable React applications. Its innovative approach to state management paves the way for more robust and responsive user interfaces. For developers seeking a modern solution, Recoil offers a promising path forward.
Previous
Step-by-Step Guide to Using Context API in React
Next