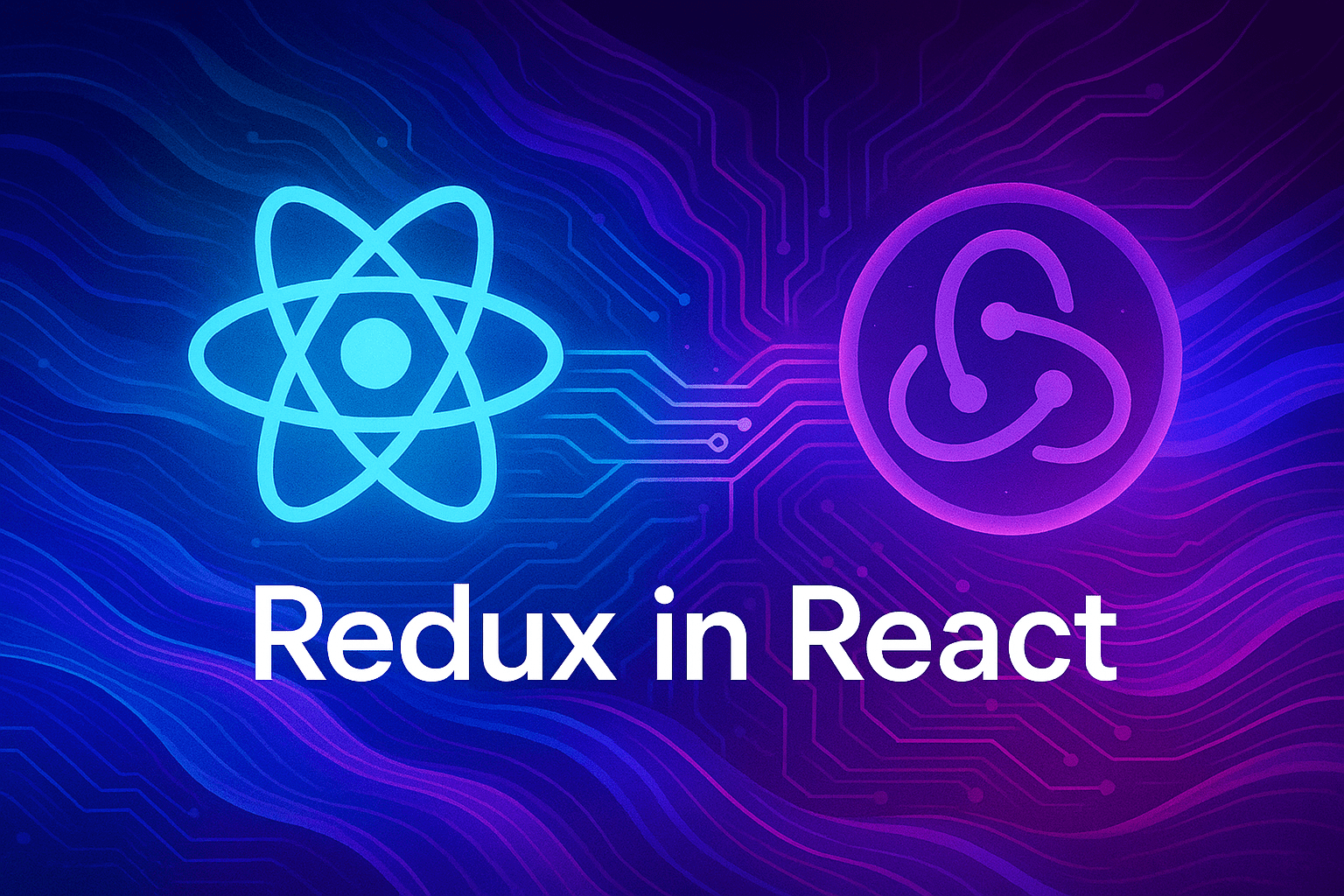
Introduction to React and Redux Integration
React is a powerful library for building user interfaces. Its component-based architecture promotes reusable, efficient code. However, as applications grow, managing state can become complex. This is where Redux comes in handy.
Redux is a predictable state container for JavaScript apps. It helps manage application state in a single store, making state management more predictable and easier to debug. Integrating Redux with React can streamline data flow and state management.
Why Integrate React with Redux?
- Centralized State: Maintain a single source of truth for your app’s state.
- Predictability: Pure functions called reducers specify how state changes in response to actions.
- Debugging: Tools like Redux DevTools make state changes traceable and debuggable.
How React and Redux Work Together
React components render UI based on the state. Redux manages the state and provides it to React components. When the state changes, components re-render accordingly.
To integrate, you need the react-redux
library. This library provides the Provider
component and connect
function. Provider
makes the Redux store available to the React components. The connect
function connects React components to the Redux store.
Steps to Integrate React and Redux
- Install Redux and react-redux packages.
- Create a Redux store using
createStore
. - Wrap your app with the
Provider
component. - Define your reducers to manage the state.
- Use the
connect
function to connect components to the store.
Following these steps will set up a robust architecture for scalable applications. As you dive deeper, you’ll appreciate the clarity and efficiency Redux brings to your React applications.
Installing Redux and Required Packages
When starting with Redux in your React project, the first step is to install the necessary packages. This sets the stage for efficient state management across your application. Let’s dive into the installation process.
Step 1: Install Redux
To get Redux up and running, you need to install the core Redux package. Open your terminal and navigate to your project directory. Then, run the following command:
npm install redux
This command downloads the latest version of Redux, adding it to your project’s dependencies. With Redux installed, you’re ready to manage application state more effectively.
Step 2: Install React-Redux
React-Redux is the official binding library for integrating Redux with React applications. It provides React components the ability to read data from a Redux store. To install React-Redux, execute the following command:
npm install react-redux
With React-Redux, you can connect your React components to the Redux store seamlessly, enabling state updates to flow throughout your app.
Step 3: Install Redux DevTools (Optional)
For debugging purposes, Redux DevTools can be incredibly helpful. This tool allows you to inspect every state change and action dispatched within your application. To integrate Redux DevTools, follow these steps:
- Install Redux DevTools Extension in your browser.
- Incorporate the DevTools setup into your Redux store configuration.
Understanding the Redux Architecture
Redux is a powerful library for managing state in JavaScript applications. It’s especially useful when working with React because it helps maintain a predictable state structure. At the core of Redux is the concept of a single source of truth, making state management easier to debug and reason about.
In Redux, the state of your application is stored in a single JavaScript object called the store. The store holds the entire state tree of your application. This means that every piece of state is stored in one place, making it easier to track changes and understand the flow of data.
Core Concepts
Redux revolves around three key principles:
- Single Source of Truth: The state of your application is stored in one place, providing a single source of truth.
- State is Read-Only: To change the state, you must dispatch an action. This ensures that the state can only be changed in a predictable way.
- Changes are Made with Pure Functions: Reducers are pure functions that take the current state and an action, and return a new state.
How Redux Works
Actions are payloads of information that send data from your application to the Redux store. You can think of them as the only source of information for the store. From there, reducers receive these actions and decide how to update the state.
Reducers are pure functions that take the current state and an action, and return a new state. They specify how the state changes in response to actions. By keeping reducers pure, they remain predictable and easy to test.
Connecting Redux to React
To connect Redux with a React component, you use the connect
function from the react-redux
library. This function connects a React component to the Redux store, allowing it to access the state and dispatch actions.
Using Redux with React ensures that your components can access and update the shared state, making your application more robust and easier to manage.
Creating a Redux Store
When integrating Redux into your React application, the first step is to create a Redux store. The store is the heart of Redux, holding the state of your entire application. Let’s explore how to set it up efficiently.
Start by installing Redux and React-Redux if you haven’t already. You can do this by running:
npm install redux react-redux
Next, you’ll need to import the necessary functions and create your store. Here’s a quick example:
import { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer);
The createStore
function takes a single argument, your root reducer. This reducer is a combination of all your application’s reducers. It’s essential to structure it well for scalability.
Using the Store
Now that your store is set up, you’ll want to provide it to your React app. You can do this with the Provider
component from React-Redux:
import { Provider } from 'react-redux'; import App from './App'; const Root = () => (); export default Root;
By wrapping your application with the Provider
, you give it access to the Redux store. This setup is crucial for connecting your React components with the Redux state.
Additional Enhancements
While this is a basic setup, consider using middleware like Redux Thunk for handling asynchronous actions. Additionally, tools like Redux DevTools can help in debugging and monitoring your state.
Connecting React Components to Redux
Integrating React components with Redux can elevate your application’s state management. This process involves connecting your UI components to the Redux store, allowing them to access and update the global state seamlessly.
Using React-Redux
React-Redux is the official binding library for Redux and React. It simplifies the process of connecting components to the Redux store. With React-Redux, the key function is connect
, which links your component to Redux.
Steps to Connect
- Create a Redux store.
- Wrap your React app with the
Provider
component, passing the store as a prop. - Use the
connect
function to link state and dispatch to your component’s props.
Code Example
Here’s a simple example to illustrate connecting a component to Redux:
import React from 'react'; import { connect } from 'react-redux'; // A simple React component const Counter = ({ count, increment }) => (); // Map Redux state to component props const mapStateToProps = state => ({ count: state.count }); // Map Redux actions to component props const mapDispatchToProps = dispatch => ({ increment: () => dispatch({ type: 'INCREMENT' }) }); // Connect component to Redux export default connect(mapStateToProps, mapDispatchToProps)(Counter);Count: {count}
By following these steps, your React components can now interact with the Redux store. This setup allows for better state management and a more organized application structure. Remember, the key is to keep your components as pure as possible while offloading state logic to Redux.
Dispatching Actions in Redux
As a developer, understanding how to dispatch actions in Redux is crucial for managing state in your React applications. Actions are payloads of information that send data from your application to the Redux store. They are the only source of information for the store.
How to Dispatch Actions
In Redux, you can dispatch actions using the dispatch()
function. This function is available on the store instance. When you dispatch an action, you send it to the reducer to update the state based on the action type and payload.
Basic Example
Let’s look at a simple example to understand how dispatching works. Consider an action to add a new item to a list:
const addItemAction = { type: 'ADD_ITEM', payload: { item: 'Learn Redux' } }; store.dispatch(addItemAction);
Using Action Creators
Action creators are functions that create actions. They make your code more readable and manageable. Here’s how you can use an action creator:
function addItem(item) { return { type: 'ADD_ITEM', payload: { item } }; } store.dispatch(addItem('Learn Redux'));
By following these steps, you can efficiently dispatch actions in Redux, enabling better state management in your React applications.
Working with Redux Middleware
Redux Middleware serves as a powerful extension point between dispatching an action and the moment it reaches the reducer. It allows for more complex asynchronous logic, logging, and error handling.
What is Redux Middleware?
Middleware lets you intercept actions before they reach the reducers. It can modify, delay, log, or perform asynchronous tasks, enriching the way you manage state in your application.
How to Implement Middleware?
To implement middleware, Redux provides the applyMiddleware
function. This function is used when creating the Redux store.
import { createStore, applyMiddleware } from 'redux'; import thunk from 'redux-thunk'; import rootReducer from './reducers'; const store = createStore( rootReducer, applyMiddleware(thunk) );
Common Middleware Examples
- Redux Thunk: Handles asynchronous actions in Redux.
- Redux Logger: Logs actions and state every time an action is dispatched.
- Custom Middleware: Create your own to handle specific needs like request caching.
Creating Custom Middleware
Creating custom middleware is straightforward. It’s a function that returns another function, which takes the next middleware in the chain as a parameter.
const myMiddleware = store => next => action => { console.log('Dispatching:', action); let result = next(action); console.log('Next State:', store.getState()); return result; };
Integrating Middleware
Integrate your custom middleware using applyMiddleware
as shown in the following example:
const store = createStore( rootReducer, applyMiddleware(thunk, myMiddleware) );
Middleware enhances the power of Redux, making it more flexible and capable of handling complex application requirements.
Handling Asynchronous Actions with Redux Thunk
In the world of React applications, handling asynchronous actions can be challenging. Redux Thunk offers a solution by allowing you to write action creators that return a function instead of an action. This function can then perform side effects like data fetching.
To get started with Redux Thunk, first, make sure it is installed in your project:
npm install redux-thunk
Next, apply the middleware to your Redux store. This enables you to dispatch functions:
import { createStore, applyMiddleware } from 'redux'; import thunk from 'redux-thunk'; import rootReducer from './reducers'; const store = createStore( rootReducer, applyMiddleware(thunk) );
Now, let’s create an asynchronous action using Redux Thunk. Suppose you need to fetch user data from an API. You can define an action creator as follows:
export const fetchUserData = () => { return async (dispatch) => { dispatch({ type: 'FETCH_USER_REQUEST' }); try { const response = await fetch('https://api.example.com/user'); const data = await response.json(); dispatch({ type: 'FETCH_USER_SUCCESS', payload: data }); } catch (error) { dispatch({ type: 'FETCH_USER_FAILURE', payload: error }); } }; };
This function dispatches different actions based on the state of the request. Using Redux Thunk, you can handle asynchronous logic seamlessly within your React application.
Debugging and Optimizing Redux Applications
Redux is powerful, but debugging and optimizing can be challenging. Here, we’ll explore strategies to enhance your workflow. You’ll learn how to efficiently track state changes and boost performance.
Utilize Redux DevTools
First, integrate Redux DevTools. This browser extension provides a visual representation of state changes. It helps you track actions and mutations over time. By doing so, you can quickly identify unexpected behavior.
Implement Action Logging
Next, use middleware for action logging. Middleware sits between dispatching an action and the moment it reaches the reducer. Here’s an example:
// Logger middleware example const logger = store => next => action => { console.group(action.type); console.info('dispatching', action); let result = next(action); console.log('next state', store.getState()); console.groupEnd(); return result; };
Optimize State Management
Keep your state minimal. Store only necessary data to reduce the complexity of your reducers. For instance, instead of storing derived data, calculate it on the fly.
Use Reselect for Memoization
Memoization optimizes performance by caching the results of expensive function calls. Use Reselect to create memoized selectors:
// Reselect example import { createSelector } from 'reselect'; const itemsSelector = state => state.items; const filteredItemsSelector = createSelector( [itemsSelector], (items) => items.filter(item => item.active) );
Profile and Analyze Performance
Finally, use React’s Profiler API. It helps you understand which components render frequently. This insight guides you in optimizing component rendering.
Conclusion
React and Redux together create a powerful combination that boosts the efficiency and scalability of your applications. Developers can harness this power to build robust, dynamic interfaces that cater to complex user needs.
Key Takeaways
- React provides a component-based architecture for building user interfaces.
- Redux offers a centralized store to manage the application’s state.
- Integration involves setting up actions, reducers, and the Redux store.
- React-Redux bindings facilitate seamless communication between components and the store.
- Middleware like Redux Thunk can handle asynchronous actions effectively.
Pros and Cons
- Pros:
- Facilitates scalable state management across large applications.
- Promotes a clear separation of concerns between UI and state logic.
- Cons:
- Can introduce boilerplate code, increasing initial setup time.
- May have a learning curve for beginners.
Previous
Why Use Recoil for React State Management?
Next