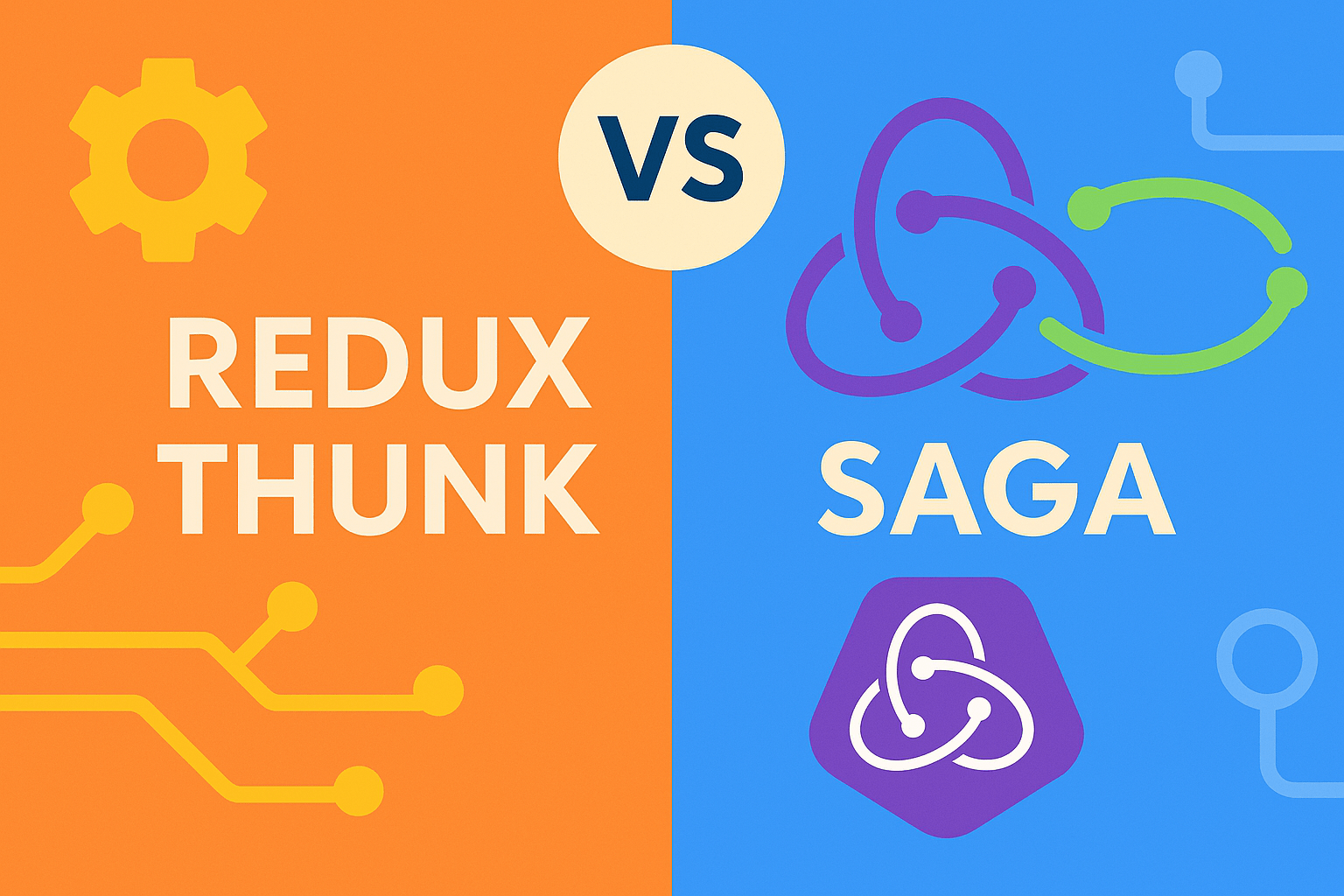
Introduction to Middleware in Redux
Middleware in Redux acts as a bridge between the dispatching of actions and the moment they reach the reducer. It’s like a customizable filter that can intercept, modify, or completely halt actions before they proceed through the Redux store. Middleware is crucial for handling asynchronous operations, logging, crash reporting, and more.
Redux by default is synchronous, which means actions are completed one at a time. However, most real-world applications require asynchronous operations, such as API calls or delayed actions. This is where middleware comes into play, enabling developers to handle complex scenarios efficiently.
Common Middleware Use Cases
- Asynchronous Data Fetching: Middleware enables the dispatching of actions after fetching data from an API.
- Logging: Middleware can log actions and states, useful for debugging.
- Error Reporting: It can catch and report errors without crashing the app.
Developers often choose between Redux Thunk and Redux Saga for handling side effects in Redux. Thunk allows you to write action creators that return a function instead of an action. This function can then perform asynchronous tasks. On the other hand, Saga uses generator functions to manage side effects, offering a more declarative approach.
Understanding the role of middleware is essential for making informed decisions about which tool to use between Redux Thunk and Redux Saga. Both have their pros and cons, but they share the common goal of managing side effects in Redux applications efficiently.
Understanding Redux Saga
Redux Saga is a middleware library that helps manage complex side effects in Redux applications. It uses generator functions to control the flow of asynchronous tasks, making it a powerful tool for handling tasks like API calls, data fetching, and more.
Unlike Redux Thunk, which relies on functions returning functions, Redux Saga uses “sagas” that are easier to test and debug. These sagas act like background processes, continuously listening for specific actions and executing tasks in response. This pattern allows for more organized and maintainable code.
Moreover, Redux Saga’s use of ES6 generators introduces a synchronous-looking syntax for asynchronous code, which can significantly improve readability. As a developer, understanding this can reduce cognitive load and make your codebase more accessible to others.
Key Features of Redux Saga
- Generator Functions: Simplify async calls with a synchronous look.
- Effect Creators: Trigger side effects in an organized manner.
- Task Management: Start, pause, or cancel long-running tasks seamlessly.
In addition, Redux Saga provides better error handling capabilities. You can catch errors in sagas and decide on fallback procedures, which is crucial in building resilient applications.
Transitioning to Redux Saga might have a steeper learning curve due to generators, but the benefits, especially in large applications, are substantial. If you’re working on projects with complex asynchronous flows, understanding Redux Saga will be a game changer.
Understanding Redux Thunk
As developers dive into state management in React applications, Redux often emerges as a popular choice. However, managing asynchronous operations can be challenging. This is where Redux Thunk enters the scene, offering a straightforward approach to handling asynchronous logic.
Redux Thunk is a middleware that allows you to write action creators that return a function instead of an action. This function can then perform side effects, including asynchronous API calls, and dispatch actions conditionally based on the results. By doing so, Redux Thunk enables you to manage asynchronous operations in a clean and organized manner.
Why Use Redux Thunk?
- Simplicity: Redux Thunk is easy to understand and implement.
- Flexibility: It provides complete control over the dispatching process.
- Integration: Redux Thunk integrates seamlessly with existing Redux setups.
As demonstrated, Redux Thunk simplifies the process of handling asynchronous logic within Redux. By utilizing functions instead of plain action objects, developers gain the ability to control the flow of actions dynamically.
Comparing Thunk and Saga
When it comes to managing side effects in Redux, developers often find themselves choosing between Redux Thunk and Redux Saga. Both middleware solutions have their strengths and weaknesses. Understanding them can help you make an informed decision.
Redux Thunk
Redux Thunk is perhaps the simplest middleware for handling side effects. It allows you to write action creators that return a function instead of an action, delaying the dispatch of an action or dispatching actions conditionally.
- Pros:
- Easy to understand and implement.
- Less boilerplate code required.
- Cons:
- Can become difficult to maintain with complex asynchronous logic.
Redux Saga
Redux Saga, on the other hand, leverages generator functions to handle side effects in a more manageable and testable way. It allows you to write more complex asynchronous flows without sacrificing readability.
- Pros:
- Handles complex asynchronous operations gracefully.
- Makes asynchronous flows easier to test.
- Cons:
- Has a steeper learning curve compared to Thunk.
Use Cases
Choosing between Thunk and Saga largely depends on the complexity of your application:
- For simple asynchronous logic, Redux Thunk is sufficient.
- If your application has complex side effects, consider using Redux Saga.
When to Use Redux Thunk
Choosing between Redux Thunk and Saga can be challenging. However, understanding when to use Thunk is essential for developers.
Redux Thunk is suitable for simple asynchronous operations. It allows you to write action creators that return a function instead of an action.
This middleware is perfect for straightforward API calls and side effects management. It’s easy to understand and integrate into existing projects.
Benefits of Using Redux Thunk
- Minimal setup and configuration
- Straightforward syntax for async operations
- Good for small to medium-sized applications
- Compatible with existing Redux architecture
Developers appreciate its simplicity, especially when the project doesn’t require complex side effects handling.
Additionally, Thunk’s lightweight nature ensures that it doesn’t bloat the project. This makes it a go-to choice for many developers.
In conclusion, if your project involves basic async tasks and you’re looking for a no-fuss solution, Redux Thunk is an excellent choice.
When to Opt for Redux Saga
As a developer, choosing between Redux Thunk and Redux Saga can be challenging. Redux Saga is a powerful middleware library that handles side effects in a Redux application. It shines in specific scenarios. Here’s when you should consider using it:
- Complex Asynchronous Operations: If your application requires handling complex async workflows, Redux Saga’s generator functions provide a more elegant solution than promises or async/await.
- Improved Readability: Generator functions allow you to write asynchronous code that looks synchronous. This can make your code easier to read and maintain, especially in large projects.
- Canceling Tasks: Redux Saga offers built-in support for task cancellation. This is crucial in scenarios where you need to abort ongoing API calls or operations based on user actions.
- Managing Multiple Parallel Processes: If your application needs to handle multiple concurrent processes, Redux Saga provides tools to manage these efficiently, such as running multiple Sagas in parallel.
- Advanced Error Handling: It allows for more sophisticated error handling strategies than Redux Thunk, which can be beneficial in robust applications.
Understanding these scenarios can assist in deciding when Redux Saga is the appropriate choice. By leveraging its capabilities, you can manage side effects effectively in your Redux application.
Performance Considerations
When it comes to performance, both Redux Thunk and Redux Saga have their strengths and trade-offs. Understanding these can help you make an informed choice.
Redux Thunk, being a middleware function, is generally simpler and more lightweight. It allows you to write action creators that return a function instead of an action. This can be beneficial for straightforward asynchronous logic. However, as your application grows, thunks can become difficult to manage. Complex async flows might lead to performance bottlenecks due to increased re-renders.
On the other hand, Redux Saga introduces a more robust way to handle side effects using ES6 generators. This can lead to more organized and scalable code. Sagas run independently of the Redux actions and reducers, which can enhance performance by reducing unnecessary re-renders. However, the learning curve for Redux Saga is steeper. The complexity of generators can be overwhelming for those unfamiliar with the concept.
Comparative Performance Table
Aspect | Redux Thunk | Redux Saga |
---|---|---|
Simplicity | High | Moderate |
Scalability | Low to Moderate | High |
Learning Curve | Low | High |
Performance | Good for simple apps | Better for complex flows |
Ultimately, the choice between Redux Thunk and Saga should consider both the current needs and the long-term project vision. While Thunk is easy to start with, Saga offers a powerful tool to manage sophisticated side effects without compromising on performance.
Conclusion
Choosing between Redux Thunk and Redux Saga depends on your project’s specific needs. Both offer impressive capabilities for managing side effects in Redux applications. However, understanding their differences is crucial for making the right decision.
Redux Thunk provides a simple and straightforward approach to handling asynchronous logic by allowing you to return functions from your action creators. It is ideal for smaller projects or when you need a quick and easy way to handle async actions without much overhead.
On the other hand, Redux Saga shines in complex applications where side effects are numerous and intricate. Its use of generator functions makes it powerful for managing more elaborate async workflows, offering better control and predictability.
Ultimately, consider the complexity of your application and the team’s familiarity with the middleware. If you prioritize simplicity and ease of learning, Redux Thunk could be your choice. However, if you require a robust and scalable solution for handling side effects, Redux Saga might be the better fit.
In summary, both tools have their strengths and can significantly enhance your Redux development workflow. The key lies in evaluating your project’s requirements and choosing the middleware that aligns best with those needs.
Previous
React & Redux: Step-by-Step Integration Guide
Next