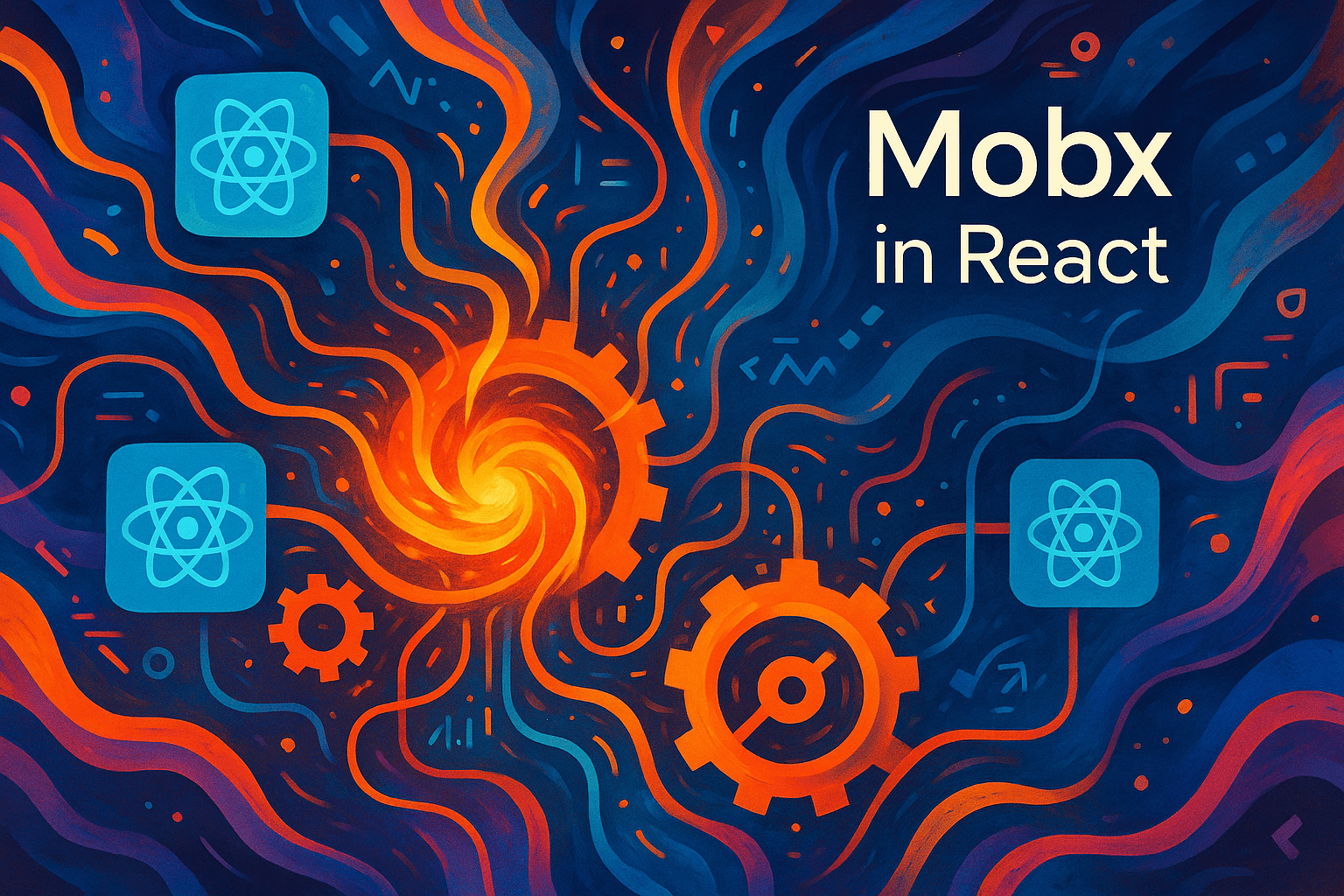
Why choose Mobx? It’s intuitive and flexible. Unlike other state management libraries, Mobx allows for mutable state, making it easier for developers to adapt and evolve their applications without cumbersome boilerplate code. This adaptability makes Mobx a favorite among developers who appreciate clarity and simplicity.
To implement Mobx in a React application, start by installing the Mobx and Mobx React packages. Then, create observable states using the `makeAutoObservable` function. This function automatically tracks state changes, reducing the need for explicit configuration. You can then use the observer function to wrap React components, allowing them to reactively respond to state changes.
One of the best practices when using Mobx is to keep your state structures simple. Avoid deeply nested states, as they can become difficult to manage. Instead, break down your state into smaller, manageable pieces. This approach not only improves the performance but also enhances the maintainability of your application.
Additionally, leverage Mobx’s computed values for derived state. These values are functions that automatically re-compute when their underlying state changes, ensuring efficient updates without redundant calculations. This feature is particularly useful for complex state transformations.
In conclusion, Mobx offers a straightforward, efficient approach to state management in React. By embracing its observable architecture, developers can create responsive applications with minimal effort. Keep it simple, leverage computed values, and enjoy the reactive power of Mobx in your next React project.
Why Choose Mobx for State Management?
State management is crucial in building scalable and maintainable applications, especially with React. While there are several options available, Mobx stands out for its simplicity and efficiency.
Firstly, Mobx is highly intuitive, making it easy for developers to learn and implement. Its usage of observable data structures allows for automatic updates to the UI, simplifying the codebase and reducing boilerplate.
Moreover, Mobx is highly flexible. It doesn’t enforce a specific architecture, allowing developers to adapt it to their unique project needs. This flexibility also means Mobx can be easily integrated into existing projects without a complete overhaul.
Key Advantages of Mobx:
- Reactiveness: Mobx automatically tracks dependencies and updates components efficiently.
- Scalability: It handles large-scale applications seamlessly, ensuring performance remains optimal.
- Minimal Boilerplate: Developers can focus more on functionality rather than setup and configuration.
Furthermore, Mobx has a vibrant community and extensive documentation, providing ample resources for troubleshooting and learning. Its active development ensures that it remains up-to-date with the latest best practices and improvements in state management.
In conclusion, choosing Mobx for state management in React projects can lead to more maintainable, efficient, and scalable applications. Its ease of use and flexibility make it a compelling choice for both new and seasoned developers.
Setting Up Mobx in a React Application
Integrating Mobx with React can streamline your state management, making it more predictable and easier to manage. To get started, ensure you have both Mobx and Mobx React installed in your project.
Step 1: Install Mobx and Mobx React
First, you need to add Mobx and Mobx React to your project. Open your terminal and run:
npm install mobx mobx-react
Step 2: Create a Store
Next, you should create a store to manage your application state. Here’s a simple example:
import { makeAutoObservable } from "mobx"; class TodoStore { todos = []; constructor() { makeAutoObservable(this); } addTodo = (todo) => { this.todos.push(todo); } } export const todoStore = new TodoStore();
Step 3: Connect Store to React Components
To make your React components aware of the Mobx store, you can use the observer
function from Mobx React. This allows components to react to any changes in the store’s state.
import React from 'react'; import { observer } from 'mobx-react'; import { todoStore } from './TodoStore'; const TodoList = observer(() => { return ({todoStore.todos.map((todo, index) => (); }); export default TodoList;{todo}
))}
With these steps, you have set up Mobx in your React application. This setup will help you manage state efficiently, keeping your application logic clear and organized.
Key Concepts of Mobx: Observables and Actions
Mobx, a powerful state management library, operates on two core concepts: observables and actions. Understanding these is crucial for seamless integration with React.
Observables: Reactions to State Changes
Observables are at the heart of Mobx. They convert your application state into reactive data. When state changes, observers react automatically. This ensures that UI components always reflect the current state.
To declare an observable, use the observable
decorator. Here’s a basic example:
const { observable } = require("mobx"); class TodoStore { @observable todos = []; }
Actions: Intentional State Modifications
Actions define how the state should be modified. They encapsulate state changes to maintain predictable application behavior. This makes debugging easier, as actions provide a clear path of state transitions.
The action
decorator is used to mark functions that will modify the state. Consider the following:
const { action } = require("mobx"); class TodoStore { @observable todos = []; @action addTodo = (todo) => { this.todos.push(todo); }; }
Implementing in React
When implementing Mobx in React, it’s essential to leverage observables and actions correctly. This ensures that components remain performant and responsive to state changes.
Use observer
from Mobx to wrap React components. This binds them to the state, automatically re-rendering when observables change.
Here’s a quick example:
import React from 'react'; import { observer } from 'mobx-react'; const TodoList = observer(({ todoStore }) => ({todoStore.todos.map((todo, index) => ()); export default TodoList;{todo}
))}
Integrating MobX with React Components
MobX offers a straightforward approach to managing state in React applications. It excels in scenarios where state needs to be shared across components, providing a reactive and efficient way to handle data changes. Let’s dive into how to integrate MobX into your React components.
Setting Up MobX in React
First, install MobX and MobX React bindings in your project. Use the following command in your terminal:
npm install mobx mobx-react-lite
Creating a MobX Store
Begin by creating a simple MobX store. This store will hold the state and actions related to your application logic.
import { makeAutoObservable } from "mobx"; class TodoStore { todos = []; constructor() { makeAutoObservable(this); } addTodo = (todo) => { this.todos.push(todo); }; } export const todoStore = new TodoStore();
Connecting MobX to React Components
Use the observer function from MobX React to make React components react to changes in the MobX store. This ensures that components update when the observable data changes.
import React from "react"; import { observer } from "mobx-react-lite"; import { todoStore } from "./TodoStore"; const TodoList = observer(() => { return ({todoStore.todos.map((todo, index) => (); }); export default TodoList;{todo}
))}
Adding Actions to the Store
Actions in MobX are functions that modify the state. You can invoke these directly from your React components to interact with the store.
import React, { useState } from "react"; import { observer } from "mobx-react-lite"; import { todoStore } from "./TodoStore"; const AddTodo = observer(() => { const [newTodo, setNewTodo] = useState(""); const handleAddTodo = () => { if (newTodo.trim()) { todoStore.addTodo(newTodo);
Implement Mobx in React: Best Practices
When working with Mobx and React, it’s essential to follow best practices to ensure that your application remains scalable and maintainable. Here are some key practices to consider:
1. Structure Your Stores
Organize your stores logically. Group related data and actions together. This improves readability and helps in managing state efficiently.
2. Use Decorators Wisely
Decorators like @observable
and @action
simplify your code. Use them to mark observables and actions clearly.
import { observable, action } from 'mobx'; class TodoStore { @observable todos = []; @action addTodo = (todo) => { this.todos.push(todo); } }
3. React Components as Observers
Wrap components with observer
to make them reactive. This ensures they re-render when observables change.
import React from 'react'; import { observer } from 'mobx-react'; const TodoList = observer(({ store }) => ({store.todos.map(todo =>));{todo}
)}
4. Minimize State in Components
Keep state in Mobx stores, not in React components. This separation of concerns leads to cleaner and more manageable code.
5. Use Computed Values
Leverage computed
values for derived data. They automatically update when dependencies change, reducing the need for manual calculations.
import { computed } from 'mobx'; class TodoStore { @observable todos = []; @computed get unfinishedCount() { return this.todos.filter(todo => !todo.finished).length; } }
6. Debugging with DevTools
Utilize Mobx DevTools for debugging. They provide insights into your store’s state and actions, aiding in troubleshooting.
Common Pitfalls and How to Avoid Them
Implementing Mobx in React can enhance your application’s performance and state management. However, certain pitfalls can impair your project’s success. Let’s explore these challenges and how to avoid them.
Overusing Observables
Overusing observables can lead to performance bottlenecks. Not every piece of data needs to be observable. Instead, make only those state parts reactive that require automatic updates.
const store = observable({ count: 0, increment() { this.count++; } });
Neglecting Computed Values
Ignoring computed values can result in excessive re-renders. Use computed values to derive data efficiently and reduce unnecessary updates.
const store = observable({ todos: [], get unfinishedTodoCount() { return this.todos.filter(todo => !todo.finished).length; } });
Incorrect Component Decoration
Forgetting to use the observer wrapper can cause components to not re-render when observable data changes. Always wrap your components with observer.
import { observer } from "mobx-react"; const TodoList = observer(({ store }) => ({store.unfinishedTodoCount} tasks remaining));
Uncontrolled Side Effects
Unmanaged side effects can lead to unpredictable behavior. Use actions to wrap any code that modifies observables to ensure consistency.
const store = observable({ count: 0, increment: action(function() { this.count++; }) });
Performance Optimization with Mobx
Optimizing performance in applications using Mobx involves several strategies to ensure smooth and efficient state management. In React, where performance is crucial, Mobx shines by minimizing re-renders and efficiently managing observable data.
Reducing Unnecessary Re-renders
Mobx optimizes reactivity by automatically tracking dependencies. This ensures that components only re-render when the data they use changes. For example, by using observer
from Mobx, you can wrap your React components to make them reactive.
import { observer } from 'mobx-react'; const MyComponent = observer(() => { return{store.myObservableData}; });
Utilizing Computed Values
Computed values in Mobx are derived from observables and are recalculated only when necessary. This reduces the computational overhead by ensuring that dependent data is updated efficiently.
import { computed, observable } from 'mobx'; class Store { @observable numbers = [1, 2, 3]; @computed get total() { return this.numbers.reduce((sum, num) => sum + num, 0); } }
Using Mobx’s action
for State Mutations
Actions in Mobx batch state updates, minimizing reactivity overhead. This means that changes to observables within an action are processed together, reducing the number of re-renders.
import { action } from 'mobx'; class Store { @observable count = 0; @action increment() { this.count += 1; } }
Testing Mobx in Your React Applications
When implementing Mobx in React, thorough testing ensures your state management works seamlessly. Testing Mobx stores and reactions is crucial for maintaining robust applications. Here’s how you can approach it effectively.
Unit Testing Mobx Stores
Unit tests help you verify the logic within your Mobx stores. Jest, a popular testing framework, integrates well with Mobx, allowing you to mock and test efficiently. Use the observable
and action
methods from Mobx to structure your tests.
import { observable } from 'mobx'; import { myStore } from './stores/myStore'; test('should update store value', () => { myStore.someAction(); expect(myStore.someValue).toBe('expectedValue'); });
Testing Mobx with React Components
Testing React components that use Mobx requires setting up the MobX provider. You can use enzyme
or @testing-library/react
for rendering components and interacting with them.
import React from 'react'; import { render } from '@testing-library/react'; import { Provider } from 'mobx-react'; import MyComponent from './MyComponent'; import { myStore } from './stores/myStore'; test('renders component and checks store', () => { const { getByText } = render(); expect(getByText(/expected text/i)).toBeInTheDocument(); });
End-to-End Testing with Mobx
End-to-end (E2E) testing tools like Cypress can simulate user interactions and test the application as a whole. Ensure your Mobx state changes reflect accurately in the user interface.
By integrating Mobx testing in your development process, you ensure consistent and predictable application behavior, resulting in more reliable React applications.
Integrating MobX into your React applications can significantly enhance state management efficiency. This powerful library provides a simple and scalable way to manage complex states. By using MobX, developers can create reactive applications where the UI automatically updates in response to state changes.
One of the primary advantages of MobX is its simplicity. Unlike other state management tools, MobX requires minimal boilerplate code. This simplicity allows developers to focus more on building features rather than managing state logic. Additionally, MobX’s observable state makes it easy to track changes and debug issues, which improves overall code maintainability.
MobX also excels in performance. It optimizes rendering by ensuring that only the necessary components update when the state changes. This optimization is beneficial in large-scale applications where performance can be a concern. As a result, users experience faster load times and smoother interactions.
Moreover, MobX’s flexibility allows it to integrate seamlessly with TypeScript, enhancing type safety in your applications. This integration helps prevent runtime errors and makes refactoring easier, giving developers more confidence in their code.
In summary, leveraging MobX for state management in React applications offers a multitude of benefits. Its simplicity, performance optimization, and flexibility make it a valuable tool for developers aiming to build efficient and scalable applications. By adopting MobX, developers can create highly reactive and maintainable applications that provide a superior user experience.
Previous
Redux Thunk vs Saga: Which to Use?
Next