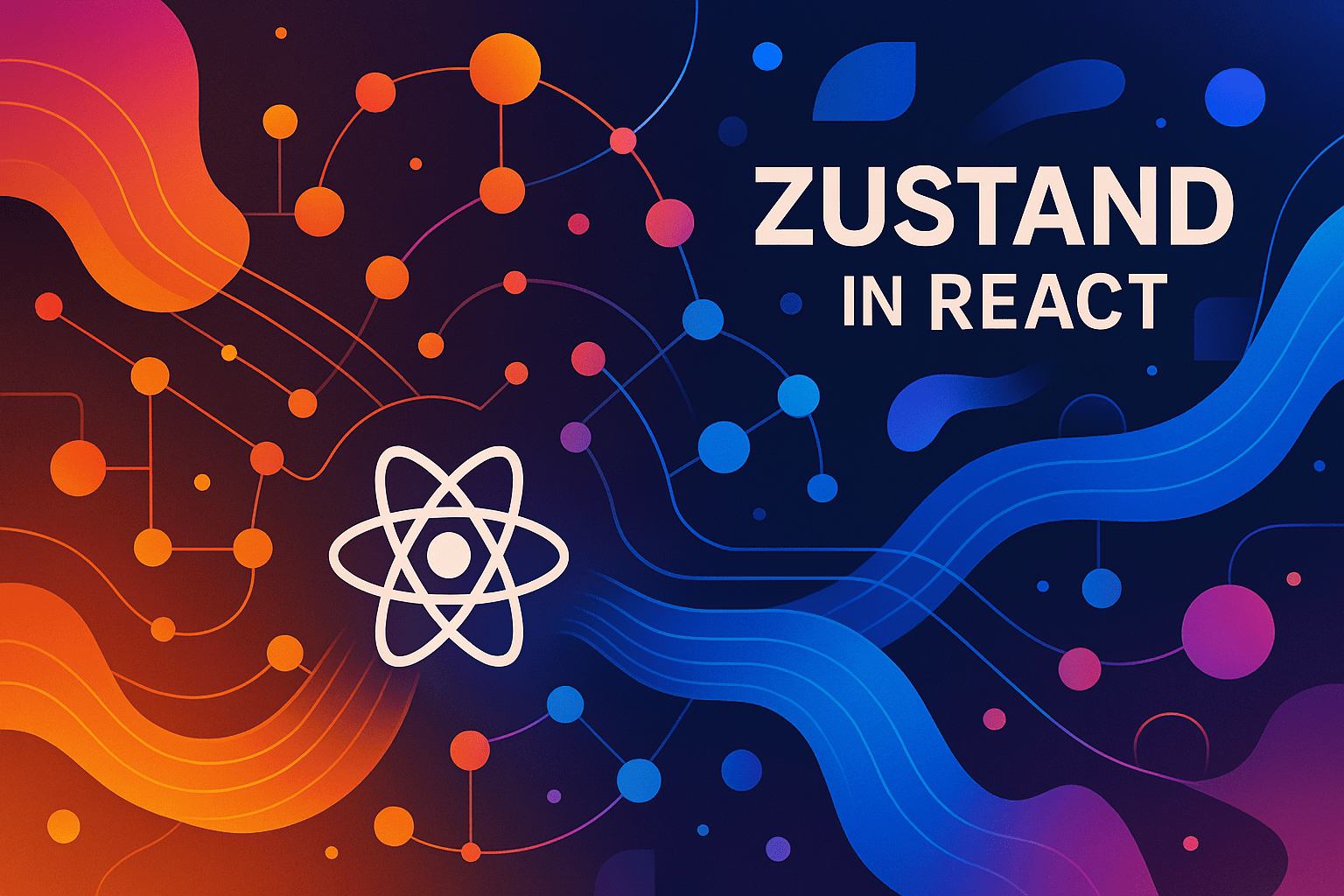
In the realm of React state management, developers often face a myriad of choices. Zustand in React, a minimalistic state management library, emerges as a preferred option for many. It offers a simple and scalable alternative to more complex solutions like Redux.
Zustand is designed with a focus on ergonomics and performance. It leverages hooks to manage state, making it a natural fit for modern React applications. By reducing boilerplate code, Zustand allows developers to concentrate on building features rather than managing state intricacies.
One of the standout features of Zustand is its API simplicity. With just a few lines of code, you can create a global store and access it throughout your application. This is a game-changer for developers seeking efficiency without sacrificing functionality.
Furthermore, Zustand is highly performant. It minimizes unnecessary re-renders, ensuring that your application runs smoothly even as it scales. This is crucial for maintaining a responsive user experience as your app grows in complexity.
Moreover, Zustand is versatile. It integrates seamlessly with both small and large applications, offering flexibility without imposing a steep learning curve. Whether you are building a simple to-do app or a complex enterprise solution, Zustand adapts to your needs.
In essence, Zustand empowers developers by simplifying state management while maintaining high performance. It’s a tool that aligns with the core principles of React, making it a worthy addition to your development toolkit.
Core Concepts of Zustand Explained
Zustand is a simple yet powerful state management library for React. Its name, meaning “state” in German, hints at its primary function: managing application state efficiently. Understanding its core concepts is crucial for leveraging its full potential.
Store Creation
At the heart of Zustand is the store. This is where your application’s state lives. You create a store using the create
function. The function takes a callback that defines the state and actions.
const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), }));
Immutable State
Zustand ensures state immutability automatically. You can safely update state without worrying about accidental mutations, which helps maintain application stability and predictability.
Selector Functions
Selectors allow you to retrieve specific parts of the state. This feature optimizes performance by preventing unnecessary re-renders.
const count = useStore((state) => state.count);
Middleware Support
Zustand supports middleware, enhancing functionality with features like logging, persistence, or even async actions. This flexibility allows developers to tailor the store to their specific needs.
Reactivity
With Zustand, reactivity is straightforward. Components that consume the state will automatically re-render when the state changes, ensuring a seamless user experience.
Integration with React
Integrating Zustand with React is hassle-free. Use the useStore
hook within your components to access and manipulate the state, making Zustand a convenient choice for managing complex state in React applications.
Conclusion
By understanding these core concepts, developers can effectively use Zustand to manage state in their React applications. Its simplicity and power make it an excellent choice for projects of any size.
Comparing Zustand with Other State Management Libraries
When it comes to managing state in React applications, developers often find themselves choosing between several libraries. Zustand, Redux, and MobX are some of the popular options. Each has its strengths and weaknesses. Here, we’ll compare Zustand with these libraries to help you make an informed decision.
Zustand vs. Redux
- Boilerplate: Redux is known for its extensive boilerplate. Zustand, on the other hand, requires minimal setup, making it quicker to implement.
- Learning Curve: Redux can be complex for beginners due to its middleware and action concepts. Zustand is more straightforward and easier to grasp.
- Performance: Zustand is optimized for performance with a smaller bundle size, which can be crucial for large applications.
Zustand vs. MobX
- Reactivity: MobX provides automatic reactivity, which can be powerful but sometimes unpredictable. Zustand offers a more controlled approach.
- Debugging: Zustand’s simpler structure makes debugging more manageable compared to MobX’s complex observable patterns.
- Community: While MobX has a vibrant community, Zustand’s popularity is growing, providing increasing support and resources.
Zustand’s simplicity is evident. With fewer lines of code, you can manage state efficiently. Whether you’re working on a small project or a large-scale application, Zustand provides a scalable solution without the complexity of other libraries.
Implementing Zustand: A Step-by-Step Guide
Zustand offers a simple and efficient way to manage state in React applications. This guide will take you through the process of implementing Zustand, ensuring your application is both scalable and maintainable.
1. Install Zustand
First, you need to add Zustand to your project. Use the following command:
npm install zustand
2. Create a Store
Next, create a store that will hold your application’s state. Start by creating a new file named store.js:
import create from 'zustand'; const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), decrement: () => set((state) => ({ count: state.count - 1 })), })); export default useStore;
3. Use the Store in Components
With the store set up, you can now use it within your React components. For example, in a component named Counter.js:
import React from 'react'; import useStore from './store'; const Counter = () => { const { count, increment, decrement } = useStore(); return (); }; export default Counter;{count}
4. Integrate with React Application
Finally, integrate the component with your existing React application. Import and use the Counter component in your App.js:
import React from 'react'; import Counter from './Counter'; function App() { return (); } export default App;
Advanced Techniques with Zustand
Zustand, a small and fast state management tool, has revolutionized how developers manage state in React applications. As you delve deeper, several advanced techniques can supercharge your Zustand experience.
Selectors for Efficient State Access
Selectors allow you to extract specific slices of state, optimizing component rendering. Instead of passing the entire state, you can efficiently select only what’s necessary.
const useBearCount = (state) => state.bears; const bearCount = useStore(useBearCount);
Middleware for Enhanced State Management
Zustand supports middleware to extend its functionality. Useful for logging, debugging, or handling more complex state logic.
import { devtools } from 'zustand/middleware'; const useStore = create(devtools((set) => ({ bears: 0 })));
Persisting State Across Sessions
State persistence can significantly enhance user experience. Zustand integrates seamlessly with local storage, making state persistence straightforward.
import { persist } from 'zustand/middleware'; const useStore = create(persist((set) => ({ bears: 0 }), { name: 'bear-storage' }));
Hydration for Server-Side Rendering
For server-side rendered apps, Zustand provides hydration to synchronize server and client state, ensuring a smooth user experience.
useEffect(() => { const stateFromServer = getStateFromServer(); useStore.setState(stateFromServer); }, []);
Combining Multiple Stores
As applications grow, you might need multiple stores. Zustand’s modular approach allows you to easily combine stores.
const useStoreA = create((set) => ({ bears: 0 })); const useStoreB = create((set) => ({ trees: 0 }));
Real-world Use Cases of Zustand
When it comes to state management in React, Zustand offers a simple and scalable alternative to more complex libraries. Its lightweight nature makes it suitable for a variety of real-world applications. Below are some practical use cases where Zustand shines.
1. E-commerce Platforms
In e-commerce applications, managing product states and user interactions is crucial. Zustand can handle cart states, user authentication, and product filtering efficiently. Its minimal API allows developers to create customizable state logic without the overhead.
2. Real-time Applications
Real-time applications like chat apps or live dashboards benefit significantly from Zustand’s fast state updates. It can manage multiple user states and synchronize real-time data across components seamlessly.
3. Form Management
Forms are a backbone of many applications. Zustand provides an easy way to manage form inputs, validation states, and submission processes, making it ideal for complex form handling.
4. Game Development
For developers building games with React, Zustand can manage game states, player progress, and in-game events with ease. Its performance efficiency is a boon for rendering-intensive applications.
5. Micro-Frontend Architectures
Zustand’s flexibility ensures it fits well in micro-frontend architectures where different parts of an application require independent state management. This approach keeps state logic modular and maintainable.
Common Pitfalls and How to Avoid Them
State management in React can be tricky, and using Zustand is no exception. Here are some common pitfalls developers face and how you can avoid them.
1. Overcomplicating the Store
Developers often create overly complex stores, leading to hard-to-maintain code. Keep your store simple and focused. Divide state into logical parts and avoid storing unnecessary data.
2. Ignoring Selector Functions
Selectors help optimize performance by allowing components to subscribe to specific parts of the state. Use selector functions to avoid unnecessary re-renders.
// Avoid this const user = useStore(state => state.user); // Use selectors const userName = useStore(state => state.user.name);
3. Forgetting to Unsubscribe
When using Zustand, it’s easy to forget to unsubscribe from state updates. Always clean up subscriptions to prevent memory leaks.
4. Not Using Middleware
Middleware can enhance your store’s functionality, yet it’s often underutilized. Integrate middleware for logging, persisting state, or handling asynchronous actions.
5. Mismanaging Async Actions
Handling async actions improperly can lead to inconsistent state. Use libraries like Redux Thunk or Zustand’s built-in async support for better async handling.
// Async action example const useStore = create((set) => ({ data: null, fetchData: async () => { const response = await fetch('api/data'); const data = await response.json(); set({ data }); } }));
By being mindful of these pitfalls, you can harness the full power of Zustand in your React applications.
Best Practices for Using Zustand Efficiently
In the realm of state management, Zustand offers a lightweight and scalable solution for React applications. To harness its full potential, developers should adhere to certain best practices.
Keep State Structure Flat
Avoid deeply nested states. Instead, maintain a flat structure to ensure better performance and easier updates. This aligns with React’s philosophy of immutability.
Modularize Your Stores
Break down your state into smaller, focused stores. This modular approach allows for better organization and promotes reusability across different components.
Leverage Zustand’s Middleware
Use middleware for logging, persisting, and rehydrating states. Zustand supports middleware out-of-the-box, enhancing functionality without additional complexity.
Utilize Selectors for Performance
Reduce unnecessary re-renders by using selectors. Selectors help in extracting only the needed parts of the state, optimizing component rendering.
Implement TypeScript for Type Safety
Integrate TypeScript with Zustand for better type safety and code predictability. This practice minimizes bugs and enhances code maintainability.
Avoid Overuse of Global State
While Zustand makes global state management easy, avoid overusing it. Keep local state where possible to prevent unnecessary complexity.
- Keep states simple and focused
- Use the right tool for the right job
- Balance between local and global state
Optimize Updates with Batching
Zustand allows batching of state updates. Group multiple state changes together to improve performance and reduce re-renders.
Practice | Benefit |
---|---|
Flat State Structure | Improved performance |
Modular Stores | Enhanced reusability |
Conclusion: Why Zustand is the Future of State Management in React
In the evolving world of React, Zustand emerges as a compelling choice for state management. Its simplicity and flexibility make it a preferred option for many developers. With minimal boilerplate and an intuitive API, Zustand allows for a more streamlined development process.
Unlike more complex libraries, Zustand offers a clean and efficient way to manage state. It supports both small and large applications with ease. This adaptability is crucial for developers looking to scale projects without overhauling their state management strategy.
Moreover, Zustand’s performance is noteworthy. It minimizes re-renders and optimizes updates, leading to faster applications. This efficiency not only improves user experience but also aids in maintaining high-performance standards.
Another advantage is Zustand’s compatibility with modern React features like hooks and concurrent mode. This ensures that it remains relevant and effective as React continues to evolve. Developers can leverage these features without friction, making Zustand a future-proof choice.
As the React ecosystem grows, the demand for lightweight and effective state management solutions will increase. Zustand, with its robust design and developer-friendly approach, is well-positioned to meet this demand. Its community support and continuous updates further cement its place as a leader in the field.
In summary, Zustand combines simplicity, performance, and modern compatibility. These attributes make it an ideal candidate for future state management in React applications. Developers seeking an efficient and forward-thinking solution will find Zustand to be a valuable asset.
Previous
Implement Mobx in React: Best Practices
Next