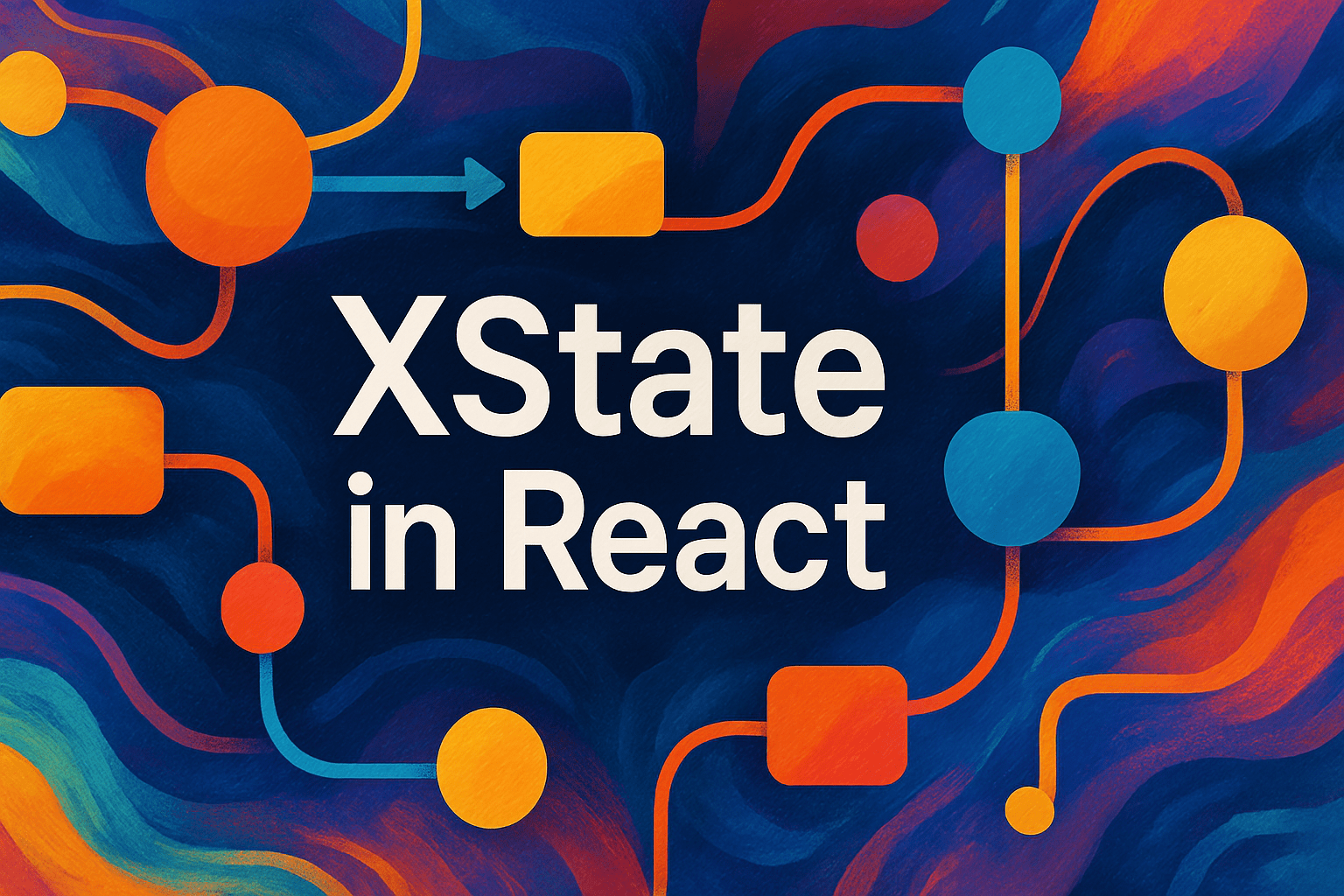
Introduction to XState and Its Importance in React
Managing state in React applications can be challenging, especially as the complexity grows. This is where XState becomes invaluable. It is a library that brings the power of state machines and statecharts to JavaScript applications, providing a robust solution for state management.
XState stands out because it offers a visual representation of state transitions. Developers can see the entire state logic, improving both debugging and collaboration. With state machines, you define a finite set of states and their transitions. This approach reduces errors and enhances code predictability.
In React, XState integrates seamlessly, offering a structured way to handle component states. It replaces intricate conditional logic with clear, declarative state machines. This not only simplifies code but also aligns with React’s declarative nature. Moreover, XState’s ability to manage side effects further enriches its utility in complex applications.
By adopting XState, React developers can elevate their state management strategy. The library ensures that state transitions are explicit and well-defined. This clarity leads to more maintainable and scalable applications. Additionally, with XState, testing becomes more straightforward. You can simulate state changes and validate expected outcomes, ensuring robust application behavior.
Understanding State Machines and State Charts
State machines and state charts are crucial concepts in managing complex state logic in applications. They offer a clear, visual representation of how an application can transition between different states. This concept is essential for developers who aim to implement robust state management in React using XState.
State machines define a finite number of states and the transitions allowed between them. You can visualize them as a graph where nodes represent states, and edges represent transitions. State charts extend this concept, allowing for hierarchical states, parallel states, and more advanced features.
Why Use State Machines?
- Predictability: They ensure that only valid transitions occur.
- Maintainability: Visual representation aids in understanding and maintaining complex logic.
- Scalability: They can easily grow with the application’s complexity.
Benefits of Using XState with React
- Enhanced state visualization
- Simplified logic for complex state transitions
- Improved predictability and reliability of state management
By integrating XState with React, your application can handle complex state transitions more effectively. This integration leads to cleaner, more maintainable code, especially as the application scales.
Managing Complex States with XState
As modern applications grow in complexity, state management becomes a critical concern for developers. Enter XState, a powerful tool for managing complex states in React applications. XState employs the concept of finite state machines, enabling developers to model complex behaviors in a more predictable manner.
Why Use XState?
- Declarative Statecharts: XState allows you to define states and transitions clearly, enhancing readability.
- Visual Tools: It provides visualizations to help understand state transitions, making debugging easier.
- Side Effects Management: XState efficiently handles side effects, reducing the complexity of asynchronous operations.
Implementing XState in React
To start using XState, install the XState library and its React integration:
npm install xstate @xstate/react
Next, define a state machine. Here’s a simple example of a toggle button:
import { createMachine } from 'xstate'; const toggleMachine = createMachine({ id: 'toggle', initial: 'inactive', states: { inactive: { on: { TOGGLE: 'active' } }, active: { on: { TOGGLE: 'inactive' } } } });
Using the Machine in a React Component
Integrate the machine with a React component using the useMachine
hook:
import React from 'react'; import { useMachine } from '@xstate/react'; import { toggleMachine } from './toggleMachine'; function ToggleButton() { const [state, send] = useMachine(toggleMachine); return ( ); } export default ToggleButton;
This setup ensures that the toggle button reflects its current state accurately, maintaining a clean and predictable state flow.
Benefits of XState
Feature | Description |
---|---|
Predictability | Finite state machines reduce unforeseen states. |
Previous
Zustand in React: Your Ultimate Guide to State Management
Next