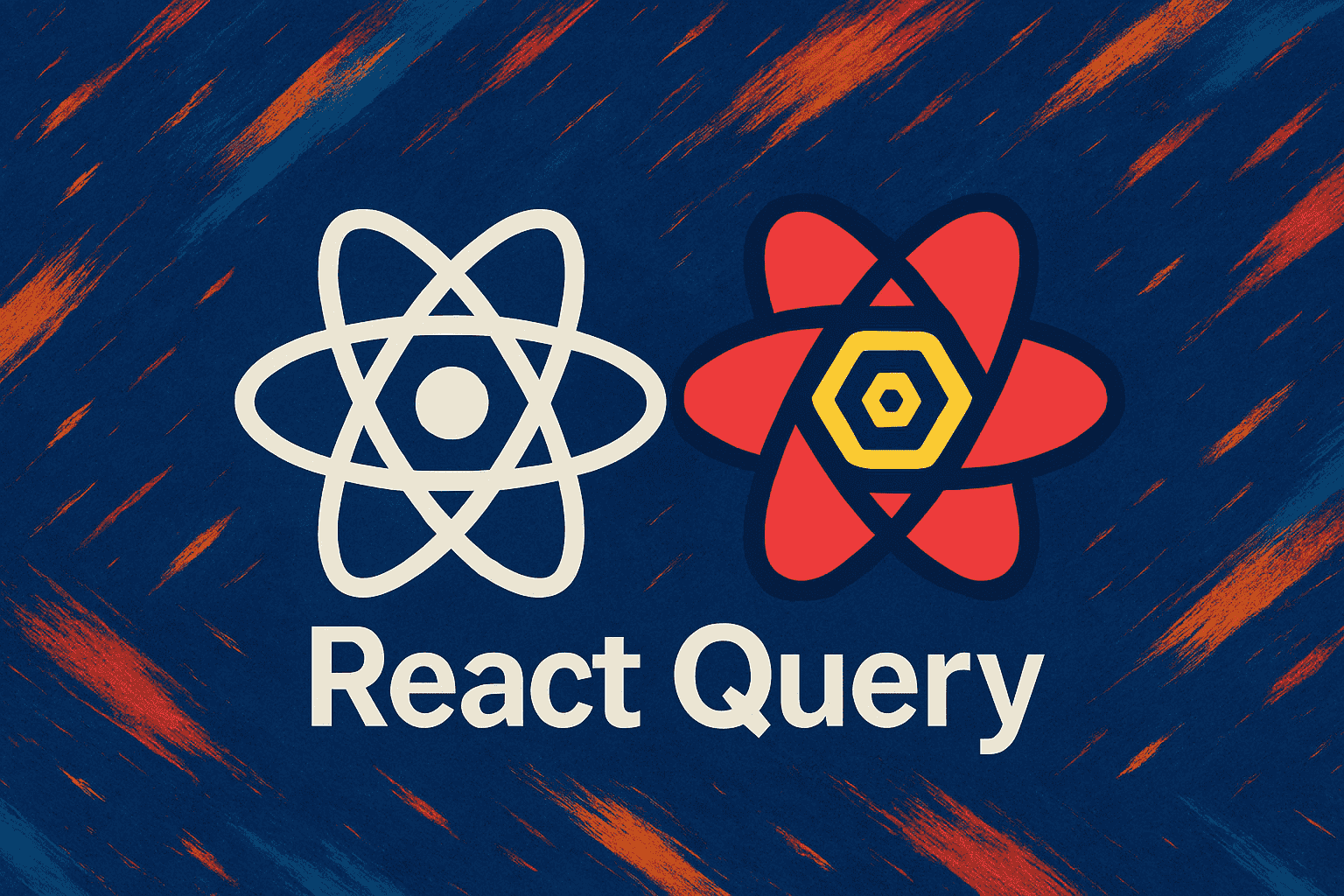
Introduction to React Query
In the bustling ecosystem of JavaScript libraries, React Query stands out as a powerful tool for managing server state in React applications. For developers who crave efficiency, simplifies data fetching, caching, and synchronization.
Imagine building a React app without the hassle of handling asynchronous data. React Query abstracts the complexities, allowing you to focus on crafting a seamless user experience. By leveraging the power of hooks, it integrates seamlessly into your component logic.
React Query’s automatic caching is a game-changer. It intelligently caches your data, reducing unnecessary network requests and ensuring your app remains responsive. This efficient caching mechanism means less boilerplate code and more productivity.
Furthermore, React Query offers out-of-the-box support for pagination and infinite scrolling. This is particularly useful for applications dealing with large datasets. By abstracting these features, React Query allows you to implement complex data patterns with ease.
Transitioning to React Query also means embracing a more organized codebase. With its built-in dev tools, you gain real-time insights into your data-fetching operations. The tools provide a visual representation of queries, mutations, and cache state.
Incorporating React Query into your React app not only boosts performance but also enhances scalability. As your application grows, React Query’s robust architecture ensures that data management remains seamless and intuitive.
Embrace the power and transform the way you handle server state. With its efficient caching, intuitive API, and powerful dev tools, React Query is the secret weapon every React developer needs.
Why Choose React Query?
React Query is a powerful tool that can transform how you handle data fetching in your React applications. It’s not just about fetching data; it’s about making your app faster, more efficient, and easier to manage.
Effortless Data Management
React Query simplifies data management by abstracting the complexities of state management. It handles caching, background updates, and error handling, so you can focus on building features.
Optimized Performance
When using React Query, you get automatic caching and refetching. This means your app will always display the most up-to-date data without unnecessary network requests.
Developer Experience
React Query provides a great developer experience with its easy-to-understand API. You can integrate it into your existing projects without a steep learning curve.
Benefits at a Glance:
- Automatic caching and refetching
- Focus on features, not boilerplate code
- Seamless integration with React
Rich Tooling
The React Query Devtools offer insights into your queries, making debugging and optimization straightforward. You can easily see the state of your queries and mutations.
SEO and User Engagement
React Query can improve SEO by ensuring your app loads data efficiently. Users will enjoy a smoother, faster experience, which can lead to higher engagement.
Getting Started with React Query
React Query is a powerful tool for managing server state in React applications. It simplifies data fetching, caching, and synchronizing server state with UI. If you’re looking to boost your React app’s performance, React Query is a game changer. Let’s dive into how you can get started.
Installation
First, you need to install React Query. You can do this using npm or yarn:
npm install react-query
or
yarn add react-query
Setting Up the Query Client
After installation, set up the QueryClient and QueryClientProvider in your application. This is essential for managing queries globally.
import { QueryClient, QueryClientProvider } from 'react-query'; const queryClient = new QueryClient(); function App() { return ({/* Your app components */} ); }
Fetching Data with useQuery
React Query provides a hook called useQuery for fetching data. This hook takes a unique key and a fetch function as parameters.
import { useQuery } from 'react-query'; function fetchUserData() { return fetch('https://api.example.com/user').then(response => response.json()); } function UserProfile() { const { data, error, isLoading } = useQuery('userData', fetchUserData); if (isLoading) returnLoading...; if (error) returnError loading data; return{data.name}; }
Handling Server State in React
Managing server state in React applications can be challenging due to asynchronous nature and potential for stale data. Using React Query simplifies this process, offering a robust solution for fetching, caching, and updating data efficiently.
Fetching Data
Define a query using the useQuery
hook. It automatically handles caching and re-fetching:
import { useQuery } from 'react-query'; function fetchUser() { return fetch('/api/user').then(response => response.json()); } function UserProfile() { const { data, error, isLoading } = useQuery('user', fetchUser); if (isLoading) returnLoading...
; if (error) returnError loading data
; return{data.name}; }
Tips for Using React Query
- Combine with context providers for global state management.
- Use query keys to manage dependent queries.
- Leverage mutation hooks for sending data.
Benefits of React Query
React Query is a powerful tool for managing server state in React applications. It’s designed for developers who seek efficiency and reliability. Using it, you can effortlessly fetch, cache, and update data without the hassle of managing the state manually.
One of the standout features of React Query is its ability to reduce boilerplate code. This means you spend less time writing repetitive code and more time enhancing your application’s functionality. The library abstracts away the complexities of data fetching, allowing you to focus on building features.
Another significant benefit is automatic caching. React Query stores fetched data and reuses it, which minimizes server requests. This not only improves performance but also provides a seamless user experience.
Additionally, React Query supports background data synchronization. When your data source updates, React Query ensures that your application reflects these changes promptly. This keeps your app’s data fresh and consistent without manual intervention.
- Automatic caching improves performance.
- Background synchronization ensures fresh data.
- Reduces boilerplate code dramatically.
- Handles complex data fetching logic.
Optimistic updates and error recovery are also part of the package. When a mutation occurs, React Query allows you to update the UI optimistically, providing instant feedback to users. If an error arises, the library offers robust recovery mechanisms, maintaining the integrity of your application.
In summary, React Query simplifies state management, enhances performance, and improves user experience. It’s a must-have tool for any React developer aiming to build efficient and resilient applications.
Optimistic Updates and Caching in React Query
Optimistic updates are a powerful technique that can make your React applications feel faster and more responsive. By assuming that a request will succeed, you can update the UI instantly. This gives users the impression of immediate feedback, even if the network request is still in progress.
How Optimistic Updates Work
Imagine a to-do list app where users can add new tasks. Instead of waiting for the server to confirm the task is added, you optimistically update the UI. The new task appears instantly, and React Query takes care of syncing with the server.
Implementing Optimistic Updates
Let’s look at an example to understand how to implement this with React Query.
const mutation = useMutation(addTodo, { onMutate: async (newTodo) => { await queryClient.cancelQueries('todos'); const previousTodos = queryClient.getQueryData('todos'); queryClient.setQueryData('todos', (old) => [...old, newTodo]); return { previousTodos }; }, onError: (err, newTodo, context) => { queryClient.setQueryData('todos', context.previousTodos); }, onSettled: () => { queryClient.invalidateQueries('todos'); }, });
Caching with React Query
React Query’s caching mechanism stores the server response and retrieves it for future use. This reduces the number of requests sent to the server and speeds up the app.
Benefits of Caching
- Improved performance by reducing network requests
- Instant UI updates with cached data
- Seamless user experience
By combining optimistic updates with caching, your React app becomes incredibly efficient. Users enjoy a fast, seamless experience as data updates and syncs in the background.
Error Handling and Retrying in React Query
Error handling is crucial for any application, and React Query makes this process smoother. It provides built-in mechanisms to tackle errors efficiently. By default, if a query fails, React Query will automatically retry the query three times before marking it as an error.
Understanding Retries
When an error occurs, React Query’s automatic retry mechanism kicks in. This feature is especially useful for network-related issues where a temporary glitch might cause a failure. You can customize the number of retries or disable them altogether.
Custom Retry Example
To adjust the retry logic, you can use the following configuration:
const queryClient = new QueryClient({ defaultOptions: { queries: { retry: 2, // Retry a query twice before failing }, }, });
Error Handling Strategies
React Query also provides a way to handle errors gracefully. You can use the onError
callback to display error messages or track errors for analysis.
OnError Callback Example
Here is a simple implementation of an error handling strategy:
useQuery('data', fetchData, { onError: (error) => { console.error('An error occurred:', error.message); }, });
Benefits of Error Handling
Efficient error handling enhances user experience by ensuring that temporary issues don’t disrupt the application. It also provides developers with insights into the issues, helping them create more resilient applications.
Tips for Effective Error Handling
- Always inform the user about the error.
- Try to recover gracefully.
- Log errors for further analysis.
Integrating React Query with Other Libraries
Integrating React Query with other libraries can significantly enhance your React application. React Query’s ability to manage server state efficiently complements many popular libraries, creating a seamless user experience.
When working with state management libraries like Redux or MobX, React Query can handle asynchronous data fetching while these libraries manage local UI state. This separation of concerns keeps your code clean and maintainable. For instance, use React Query to fetch data from an API and store user input in Redux.
Let’s see an example of integrating React Query with Axios for data fetching:
import { useQuery } from 'react-query'; import axios from 'axios'; const fetchUsers = async () => { const { data } = await axios.get('https://api.example.com/users'); return data; }; const UsersList = () => { const { data, error, isLoading } = useQuery('users', fetchUsers); if (isLoading) returnLoading...
; if (error) returnError fetching data
; return ({data.map(user => (); };{user.name}
))}
Additionally, you can integrate React Query with UI libraries like Material-UI or Tailwind CSS to create visually appealing components. Use React Query’s hooks to fetch data and Material-UI’s components to present data elegantly.
Flexibility allows it to work alongside form libraries, such as React Hook Form. Handle form submissions and validations with the form library while using React Query for data mutations.
Overall, React Query’s compatibility with other libraries makes it a powerful choice for building robust React applications. By combining the strengths of different tools, you can create a more efficient and user-friendly application.
Integrating React Query with Other Libraries
One of the strengths is its ability to integrate seamlessly with other libraries. This can enhance your app’s functionality and performance. Let’s explore how you can do this effectively.
1. React Router
React Router is commonly used for handling navigation in React apps. Combining it with React Query can improve data fetching based on route changes. Here’s how you can integrate them:
import { useQuery } from 'react-query'; import { useParams } from 'react-router-dom'; function UserProfile() { const { userId } = useParams(); const { data } = useQuery(['user', userId], fetchUserById); return{data ? data.name : 'Loading...'}; }
2. Axios
Axios is a popular library for making HTTP requests. When paired with React Query, it can handle caching and background updates. Here’s a simple example:
import axios from 'axios'; import { useQuery } from 'react-query'; function fetchUserData() { return axios.get('/api/user'); } function UserComponent() { const { data } = useQuery('userData', fetchUserData); return{data ? data.username : 'Loading...'}; }
3. Form Libraries
Form libraries like Formik or React Hook Form can be used with React Query for efficient data submission. Here’s an example using React Hook Form:
import { useForm } from 'react-hook-form'; import { useMutation } from 'react-query'; // Assume submitFormData is a function that sends form data to your backend function FormComponent() { const { register, handleSubmit } = useForm(); const mutation = useMutation(submitFormData); const onSubmit = (formData) => { mutation.mutate(formData); }; return (); }
Conclusion
Integrating React Query into your React application can be a game-changer. This powerful library simplifies state management for server data, providing a seamless developer experience. By leveraging, you can optimize network requests, enhance caching, and handle complex data-fetching scenarios with ease.
React Query offers automatic re-fetching of data, which ensures your application always displays the most up-to-date information. Furthermore, with features like pagination and infinite scrolling, you can efficiently manage large datasets without overloading the client.
The library’s built-in support for optimistic updates and error handling makes your app more robust. Users benefit from instantaneous feedback, enhancing the overall user experience. Additionally, its integration with dev tools enables easy debugging and performance monitoring.
To sum up, React Query is not just a tool but a paradigm shift in how we handle data fetching in React. It encourages best practices while reducing boilerplate code. For developers looking to build scalable and performant React applications, embracing is a step in the right direction.
Previous
How to use XState in React?
Next