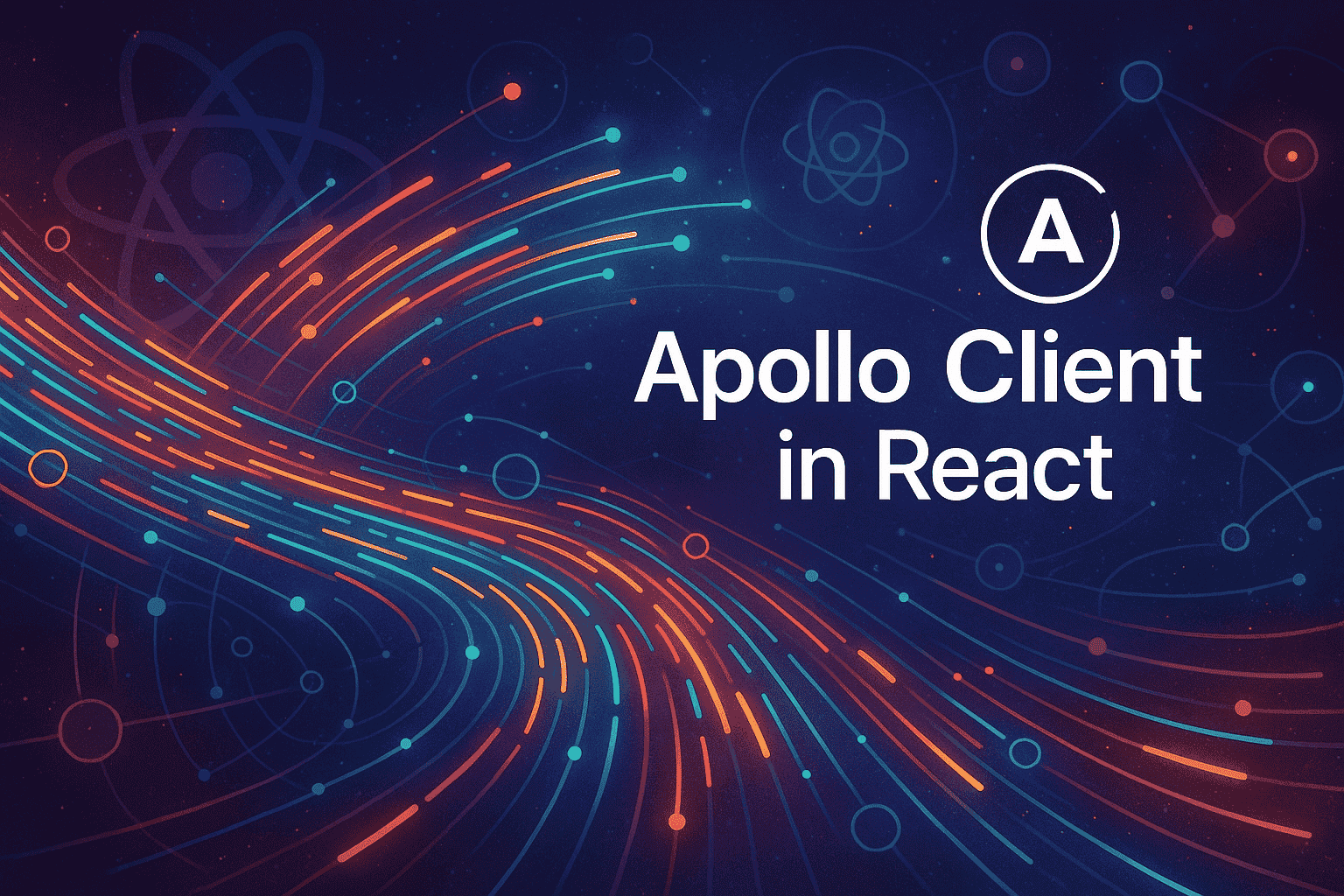
Introduction to Apollo Client
In the rapidly evolving world of web development, managing data fetching and state management efficiently is crucial. Apollo Client emerges as a powerful solution for developers working with GraphQL APIs. It not only simplifies data management but also enhances the overall performance of your React applications.
Apollo Client is a comprehensive library that helps developers interact seamlessly with GraphQL endpoints. It provides a robust state management system, allowing you to fetch, cache, and update application data effortlessly. This means you can focus on crafting engaging user experiences without getting bogged down in data handling complexities.
One major advantage of Apollo Client is its ability to integrate smoothly with existing React applications. It offers a declarative approach to data fetching, making your code more readable and maintainable. Additionally, it supports real-time updates through subscriptions, ensuring your app remains responsive to changes.
- Easy Integration: Apollo Client works seamlessly with React, enhancing the development process.
- Efficient Caching: It automatically caches responses, reducing redundant network requests.
- Declarative Data Fetching: Simplifies the code and improves readability.
- Real-time Updates: Supports subscriptions for dynamic data updates.
By leveraging Apollo Client in your React projects, you gain a competitive edge in developing scalable and efficient applications. It empowers you to build high-performance apps with minimal effort, letting you focus on delivering value to your users.
Installing Apollo Client
To get started with Apollo Client in your React application, the first step is to install the necessary packages. This process is straightforward and can be done using either npm or yarn, depending on your preference. Let’s dive into the installation process.
Step 1: Install Apollo Client
Open your terminal and navigate to your project directory. Use the following command to install Apollo Client along with GraphQL:
npm install @apollo/client graphql // or yarn add @apollo/client graphql
This command will add Apollo Client and the GraphQL package to your project’s dependencies. Now you are ready to set up Apollo Client in your application.
Step 2: Set Up Apollo Client
After installation, the next step is to configure Apollo Client in your app. Start by creating an instance of ApolloClient and link it to your GraphQL endpoint.
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://your-graphql-endpoint.com/graphql', cache: new InMemoryCache() });
This code snippet demonstrates how to create a new ApolloClient instance. The uri option specifies your GraphQL server endpoint, and InMemoryCache is used to cache query results.
Step 3: Provide Apollo Client to Your App
Finally, wrap your React component tree with the ApolloProvider component. This makes the client available to your entire app.
import React from 'react'; import { ApolloProvider } from '@apollo/client'; import { App } from './App'; const Root = () => (); export default Root;
By placing your main App component within ApolloProvider, you enable the use of Apollo Client throughout your React application, allowing you to execute GraphQL operations seamlessly.
Configuring Apollo Client
Setting up Apollo Client in a React application can seem daunting, but it’s quite straightforward once you grasp the essentials. Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. Here’s how you can configure it effectively.
Setup Apollo Client
After installation, you need to create an instance of ApolloClient. This involves specifying the URI of your GraphQL server and setting up a cache for efficient data handling.
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://your-graphql-endpoint.com/graphql', cache: new InMemoryCache() });
Integrate with React
Next, wrap your React application in an ApolloProvider
. This step allows any component within the app to interact with the Apollo Client.
import { ApolloProvider } from '@apollo/client'; import App from './App'; function Root() { return (); } export default Root;
Fetching Data
With the Apollo Client configured, you can now fetch data using the useQuery
hook. This hook simplifies data retrieval from your GraphQL server.
import { useQuery, gql } from '@apollo/client'; const GET_DATA = gql` query GetData { data { id name } } `; function DataComponent() { const { loading, error, data } = useQuery(GET_DATA); if (loading) returnLoading...
; if (error) returnError: {error.message}
; return ({data.data.map(({ id, name }) => (); }{name}
))}
Creating a GraphQL Query
When you’re working with Apollo Client in a React project, one of the first things you’ll encounter is creating a GraphQL query. This is a pivotal step in fetching data from your server and integrating it with your application’s state. Let’s dive into how you can start crafting these queries effortlessly.
Understanding the Basics
GraphQL queries are structured in a way that allows you to specify exactly what data you need, making it efficient and concise. Unlike REST APIs, where you might over-fetch or under-fetch data, GraphQL ensures you get precisely what you ask for.
Writing Your First Query
To create a GraphQL query, you need to define the data requirements using the GraphQL syntax. Here’s a simple example of a query that fetches a list of books with their titles and authors:
const GET_BOOKS = gql` query { books { title author } } `;
Integrating with Apollo Client
Once you’ve defined your query, the next step is to integrate it with Apollo Client in your React component. Using the useQuery
hook provided by Apollo, you can easily fetch data and manage its state.
import { useQuery } from '@apollo/client'; const BooksList = () => { const { loading, error, data } = useQuery(GET_BOOKS); if (loading) returnLoading...
; if (error) returnError: {error.message}
; return ({data.books.map((book, index) => (); };{book.title} by {book.author}
))}
Best Practices
- Always define the exact fields you need in a query.
- Handle loading and error states to enhance user experience.
- Utilize Apollo Client’s caching to optimize performance.
By following these steps, you can leverage the power of GraphQL and Apollo Client to build efficient, scalable applications. Whether you’re fetching simple data or performing complex operations, GraphQL queries will be a cornerstone of your development process.
Integrating Apollo Client with React Components
Configure the Apollo Client at the root of your React application. This involves initializing the client and wrapping your app with the ApolloProvider
component.
import React from 'react'; import ReactDOM from 'react-dom'; import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client'; import App from './App'; const client = new ApolloClient({ uri: 'https://your-graphql-endpoint.com/graphql', cache: new InMemoryCache() }); ReactDOM.render(, document.getElementById('root') );
With your Apollo Client set up, you can now integrate it with your React components. Use the useQuery
hook to fetch data. This hook simplifies data retrieval by automatically managing loading and error states.
import React from 'react'; import { useQuery, gql } from '@apollo/client'; const GET_DATA = gql` query GetData { items { id name } } `; function DataComponent() { const { loading, error, data } = useQuery(GET_DATA); if (loading) returnLoading...
; if (error) returnError :(
; return ({data.items.map(({ id, name }) => (); }{name}
))}
This example demonstrates how easy it is to integrate Apollo Client with React. By leveraging the power of GraphQL, you can enhance data management in your applications.
Handling Data and Loading States
When developing with Apollo Client in React, efficiently managing data and loading states is crucial. This ensures a smooth user experience and optimizes app performance. Let’s dive into how you can handle these states dynamically.
Understanding Loading States
Loading states are essential for informing users about data-fetching activities. Apollo Client provides a straightforward way to manage these states using the useQuery
hook. This hook returns a loading
property, which you can leverage to display loading indicators.
const { loading, error, data } = useQuery(GET_DATA_QUERY); if (loading) returnLoading...
; if (error) returnError :(
;
Handling Data Once Loaded
Once the data is fetched, you need to render it in a way that’s both efficient and user-friendly. You can structure your data presentation using components that are aware of the data’s structure.
return ({data.items.map((item) => ();))}
Optimizing Performance
To enhance performance, consider implementing pagination or infinite scrolling. These techniques can prevent overwhelming the user with too much information at once and reduce initial load times.
- Use
fetchMore
for pagination - Implement lazy loading for images and assets
Using Apollo Client’s Cache Effectively
Leveraging Apollo Client’s cache can significantly enhance the performance of your React application. It reduces the need for redundant network requests, making your app faster and more responsive. Here, we’ll explore some strategies to effectively use Apollo Client’s cache.
Understanding the Cache
Apollo Client’s cache is a normalized, in-memory data store. It allows you to read and write data directly, bypassing the need for server interactions. This becomes particularly useful in scenarios where data doesn’t frequently change.
Strategies for Effective Caching
- Use Cache-First Policy: This policy retrieves data from the cache first. It only queries the server if the data is not present, reducing server load.
- Utilize Cache-Only Policy: This is ideal when you know the data won’t change. It ensures the operation is entirely dependent on the cache.
- Write Directly to Cache: Sometimes, you want to update the cache immediately after a mutation. Use the
writeQuery
orwriteFragment
methods for this purpose.
Code Example
// Writing directly to cache after a mutation const updateCache = (cache, { data: { addItem } }) => { cache.modify({ fields: { items(existingItems = []) { const newItemRef = cache.writeFragment({ data: addItem, fragment: gql` fragment NewItem on Item { id name } ` }); return [...existingItems, newItemRef]; } } }); };
Cache Eviction
Sometimes, you may need to evict data from the cache to free up memory or ensure fresh data is fetched. Apollo provides methods like cache.evict
and cache.gc
for this purpose.
Using Cache Effectively
By effectively managing the cache, you can create a seamless user experience. It allows your application to be both performant and efficient, handling data with precision. With these strategies, start optimizing your Apollo Client’s cache today!
Error Handling in Apollo Client
When working with Apollo Client in React, efficiently managing errors is crucial for providing a smooth user experience. Handling errors gracefully ensures that your application can recover from issues and provide useful feedback to users.
Understanding Apollo Client Error Handling
Apollo Client provides a robust mechanism for error handling. It distinguishes between network errors, which occur when there’s a problem with the server, and GraphQL errors, which are issues with the request or response.
Implementing Error Handling
You can handle errors using the onError
link, which can be added to your Apollo Client setup. This allows you to intercept errors globally and respond appropriately.
Example: Setting Up Error Handling
import { ApolloClient, InMemoryCache, ApolloLink, HttpLink } from '@apollo/client'; import { onError } from '@apollo/client/link/error'; const errorLink = onError(({ graphQLErrors, networkError }) => { if (graphQLErrors) { graphQLErrors.forEach(({ message, locations, path }) => console.log(`[GraphQL error]: Message: ${message}, Location: ${locations}, Path: ${path}`) ); } if (networkError) { console.log(`[Network error]: ${networkError}`); } }); const client = new ApolloClient({ link: ApolloLink.from([ errorLink, new HttpLink({ uri: 'https://example.com/graphql' }) ]), cache: new InMemoryCache() });
User-Friendly Feedback
Ensure your application provides user-friendly feedback. Display error messages that are clear and actionable. This helps users understand what went wrong and what they can do next.
Testing Error Scenarios
Regularly test different error scenarios. Simulate network failures and malformed queries to see how your application behaves. This proactive approach helps in identifying potential issues early.
By carefully implementing error handling, you can significantly improve the resilience and user experience of your application.
Advanced Features and Best Practices
When working with Apollo Client in React, leveraging advanced features can significantly enhance your application’s performance and maintainability. Understanding these features can set your project apart.
1. Utilize Fragments
Fragments allow you to reuse parts of queries and keep your code DRY (Don’t Repeat Yourself). This not only saves time but also ensures consistency across multiple queries.
const userFragment = gql` fragment UserDetails on User { id name email } `;
2. Implement Cache Management
Efficient cache management is crucial for enhancing the performance of your application. Apollo Client offers in-memory caching that can be customized to suit your needs.
const client = new ApolloClient({ cache: new InMemoryCache(), });
3. Use Error Handling
Error handling ensures that your application can gracefully manage server or network issues. Apollo Client provides tools to manage and display errors effectively.
const { loading, error, data } = useQuery(GET_DATA); if (error) returnError: {error.message}
;
4. Optimize Network Requests
Using features like polling and refetching can optimize how your application makes network requests, keeping data fresh without overwhelming the server.
const { startPolling, stopPolling } = useQuery(GET_DATA, { pollInterval: 5000, });
5. Adopt Best Practices
Adopting best practices such as modularizing your code, using TypeScript for type safety, and following naming conventions can make your application more robust and easier to maintain.
Conclusion
Embarking on a journey with Apollo Client and React can be both rewarding and enlightening. This powerful duo enables developers to efficiently manage state and data fetching in React applications, creating a seamless user experience.
By integrating Apollo Client, you unlock the potential to easily work with GraphQL, leverage caching for performance optimization, and simplify data management. This ensures that your application remains responsive and dynamic, even as it scales.
Moreover, Apollo Client’s intuitive API and comprehensive documentation make it accessible for both beginners and seasoned developers. This means you can quickly get up to speed and start building robust applications without being bogged down by steep learning curves.
As you delve deeper into this powerful combination, remember that the open-source community is a valuable resource. Engage with other developers, contribute to discussions, and draw on shared knowledge to enhance your projects.
Ultimately, mastering Apollo Client with React sets the stage for creating efficient, scalable, and modern web applications. The skills you gain will not only enhance your current projects but also prepare you for future challenges in the ever-evolving tech landscape.
Previous
How to use React Query?
Next