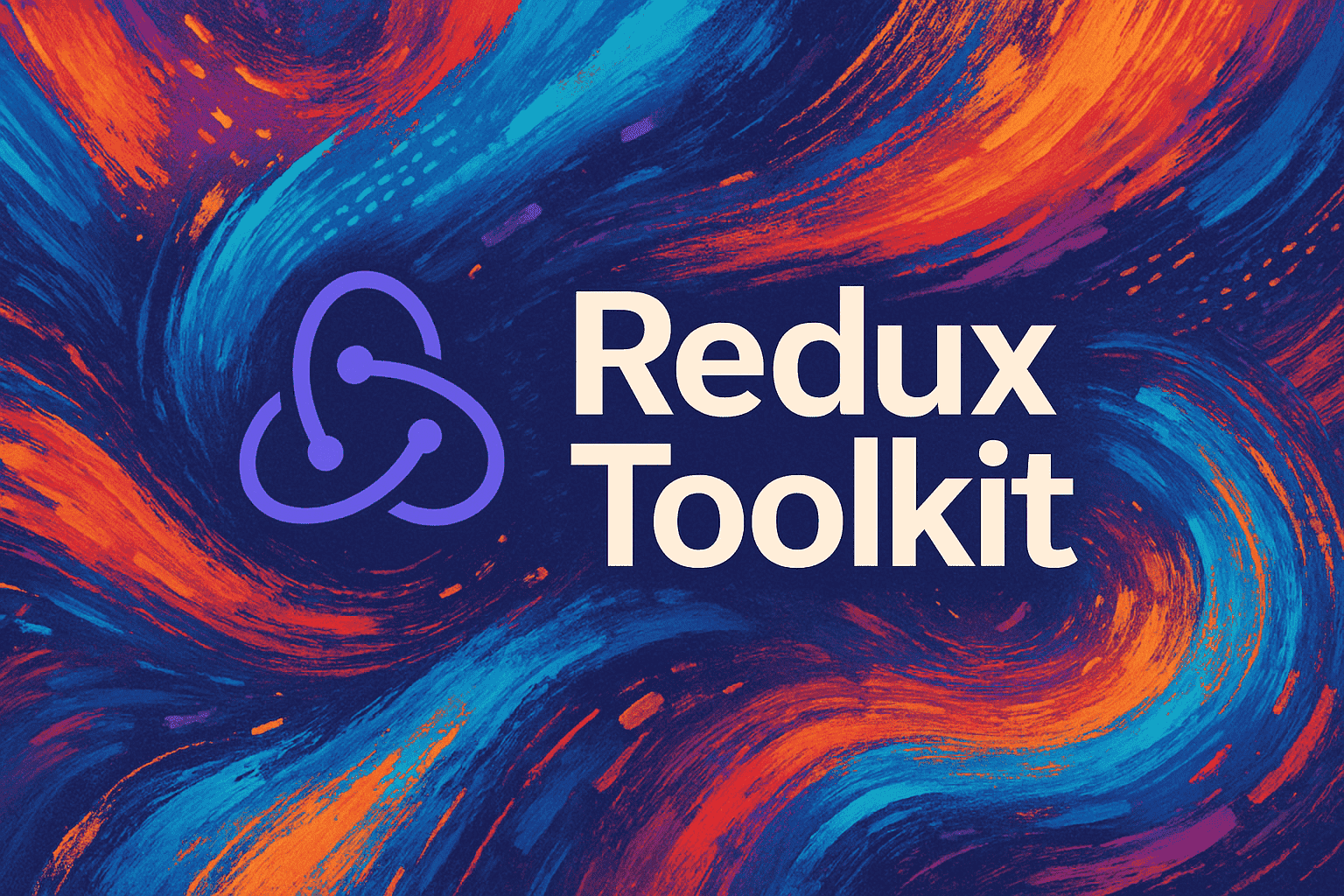
Introduction to Redux Toolkit in React
In the world of React development, managing state efficiently is crucial. Redux has been a popular choice for this task, but it can be complex and verbose. Enter Redux Toolkit, a library designed to simplify the process of using Redux in your applications. It offers a set of tools that make state management more straightforward and less error-prone.
Redux Toolkit eliminates boilerplate code and provides powerful features like createSlice and configureStore. These features streamline the setup of reducers, actions, and the store. With Redux Toolkit, developers can focus on building features rather than wrestling with configuration.
Why Use Redux Toolkit?
Redux Toolkit addresses common pain points by offering sensible defaults and simplifying setup. It encourages best practices while reducing the need for custom boilerplate code. For example, the createSlice function bundles actions and reducers together, making your codebase cleaner.
- Reduced boilerplate code
- Improved maintainability
- Integrated Redux DevTools support
- Built-in middleware for asynchronous logic
Additionally, Redux Toolkit seamlessly integrates with other popular libraries like React-Redux, making it an excellent choice for both new and existing projects.
Key Features
Feature | Description |
---|---|
createSlice | Combines reducers and actions in one place. |
configureStore | Sets up the store with sensible defaults and middleware. |
createAsyncThunk | Handles complex asynchronous logic easily. |
Redux Toolkit is a modern approach to state management in React applications. By simplifying Redux setup and reducing boilerplate, it allows developers to focus on what truly matters: building great user experiences.
Installing Redux Toolkit
As a developer ready to enhance your React application with Redux Toolkit, the first step is installation. This process is straightforward, allowing you to quickly integrate robust state management into your project. Let’s dive into the steps to get started with Redux Toolkit.
Step 1: Setup Your React Project
Before installing Redux Toolkit, ensure you have a React project ready. If not, create one using Create React App:
npx create-react-app my-app cd my-app
Step 2: Install Redux Toolkit
With your React project prepared, it’s time to install Redux Toolkit. Use npm or yarn to do this:
# Using npm npm install @reduxjs/toolkit react-redux # Using yarn yarn add @reduxjs/toolkit react-redux
Step 3: Verify Installation
Once the installation is complete, verify it by checking your project’s package.json
file. You should see @reduxjs/toolkit
and react-redux
listed under dependencies. This confirms Redux Toolkit is ready for use in your project.
Transition to Redux Toolkit
Redux Toolkit simplifies setting up Redux in your application. It reduces boilerplate code, making your development process more efficient. Follow these installation steps to integrate Redux Toolkit seamlessly.
Creating a Redux Slice
In the world of state management with Redux, slices are a game-changer. They help you organize your Redux code more efficiently. A slice represents a segment of your Redux state and contains all related reducers and actions.
Step 1: Define Your Slice
Creating a slice is straightforward with Redux Toolkit. Let’s create a simple counter slice:
import { createSlice } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: { value: 0 }, reducers: { increment: (state) => { state.value += 1; }, decrement: (state) => { state.value -= 1; } } }); export const { increment, decrement } = counterSlice.actions; export default counterSlice.reducer;
Step 2: Adding Slice to Store
Next, integrate your slice into the Redux store:
import { configureStore } from '@reduxjs/toolkit'; import counterReducer from './counterSlice'; const store = configureStore({ reducer: { counter: counterReducer } }); export default store;
Step 3: Using Your Slice in a Component
Finally, use the slice within a React component:
import React from 'react'; import { useSelector, useDispatch } from 'react-redux'; import { increment, decrement } from './counterSlice'; function Counter() { const count = useSelector((state) => state.counter.value); const dispatch = useDispatch(); return ({count}); } export default Counter;
Integrating Redux Store with React
Integrating Redux with React can seem daunting, but Redux Toolkit makes it much more approachable. Let’s dive into how you can seamlessly integrate Redux with your React application.
Creating the Redux Store
Let’s create a simple Redux store. Start by defining a slice with createSlice:
import { configureStore, createSlice } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: { value: 0 }, reducers: { increment: state => { state.value += 1 }, decrement: state => { state.value -= 1 } } }); const store = configureStore({ reducer: { counter: counterSlice.reducer } }); export const { increment, decrement } = counterSlice.actions; export default store;
In the example above, we defined a counter slice with two actions: increment and decrement.
Connecting the Store to React
With the store ready, it’s time to connect it to your React components. Wrap your application with the Provider
component:
import React from 'react'; import ReactDOM from 'react-dom'; import { Provider } from 'react-redux'; import App from './App'; import store from './store'; ReactDOM.render(, document.getElementById('root') );
Now your application is connected to the Redux store. Any component can access the state or dispatch actions.
Accessing State and Dispatching Actions
To read from the Redux store, use the useSelector
hook. To dispatch actions, use the useDispatch
hook:
Dispatching Actions in Redux
Once you’ve set up Redux in your React project, dispatching actions becomes a powerful way to update the state. With Redux Toolkit, this process is streamlined, making it easier for developers to manage state changes effectively.
Why Dispatch Actions?
Dispatching actions is crucial because it allows your application to respond to user interactions and other events in a predictable manner. By dispatching actions, you trigger changes in the state, which in turn updates the UI.
How to Dispatch an Action
In Redux Toolkit, dispatching actions is straightforward. First, you’ll define an action in your slice, and then use the dispatch
method to execute it.
Example
Imagine you have a counter slice with an action called increment
. You can dispatch this action from a React component like so:
import { useDispatch } from 'react-redux'; import { increment } from './counterSlice'; function CounterComponent() { const dispatch = useDispatch(); const handleIncrement = () => { dispatch(increment()); }; return ( ); }
Understanding the Flow
When the button in the example is clicked, the handleIncrement
function dispatches the increment
action. This action is then processed by the reducer, updating the state accordingly. The UI automatically reflects this change due to React’s reactivity.
Tips for Effective Dispatching
- Plan actions: Ensure each action serves a specific purpose.
- Use middleware: Consider middleware like Thunk for handling asynchronous operations.
- Keep actions simple: Aim for actions that are easy to understand and maintain.
By mastering action dispatching with Redux Toolkit, you can create more responsive and maintainable applications. Remember, practice makes perfect, so keep experimenting with different patterns and techniques.
Using Selectors to Access State
In Redux, selectors are essential tools for accessing and deriving state from the store. They help retrieve specific slices of state, ensuring your components remain efficient and performant.
Why Use Selectors?
Selectors promote reusability and maintainability. They encapsulate logic for retrieving state, keeping components clean. This abstraction makes state management easier to understand and modify.
Creating a Selector
A basic selector is a function that takes the entire state as an argument and returns a part of it. Here’s a simple example:
const selectUser = (state) => state.user;
In this example, selectUser
extracts the user
slice from the state. When used, it ensures that only the necessary data is accessed.
Using Selectors with useSelector
The useSelector
hook in React-Redux allows components to access state through selectors. This method ensures your components are aware of relevant state changes.
import { useSelector } from 'react-redux'; const UserProfile = () => { const user = useSelector(selectUser); return{user.name}; };
By using useSelector
, the UserProfile
component subscribes to changes in the user
state. This ensures the UI updates when the state changes.
Memoizing Selectors for Performance
For complex state derivations, memoized selectors are beneficial. They prevent unnecessary recalculations, enhancing performance.
Libraries like Reselect can help create memoized selectors:
import { createSelector } from 'reselect'; const selectUser = (state) => state.user; const selectUserName = createSelector( [selectUser], (user) => user.name );
Testing Redux Logic
When working with Redux Toolkit in a React application, testing the logic of your Redux slices is crucial. It ensures your state management works as expected, making your application more reliable and maintainable.
To begin testing Redux logic, focus on action creators and reducers. These are the core of your state management and must behave predictably. By using libraries like Jest and React Testing Library, you can write tests that simulate real-world scenarios.
Testing Action Creators
Action creators are functions that return action objects. They are the first point of interaction with your Redux store. Testing them involves verifying that they produce the correct actions.
import { createAction } from '@reduxjs/toolkit'; const increment = createAction('counter/increment'); test('increment action creator returns the correct action', () => { expect(increment()).toEqual({ type: 'counter/increment' }); });
Testing Reducers
Reducers transform the current state into a new state based on the action received. Testing reducers involves checking if they return the expected state.
import { createSlice } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: 0, reducers: { increment: state => state + 1, }, }); const { reducer } = counterSlice; test('reducer increments state', () => { expect(reducer(0, { type: 'counter/increment' })).toBe(1); });
As you can see, testing Redux logic is straightforward with Redux Toolkit. By focusing on action creators and reducers, you ensure your application’s state management is robust and reliable.
Optimizing Performance with Redux Toolkit
When building complex applications with React, managing state efficiently is crucial. Redux Toolkit offers a streamlined approach to state management that enhances performance and developer experience.
Why Use Redux Toolkit?
Redux Toolkit simplifies the process of writing Redux logic, reducing boilerplate and ensuring better code quality. It provides a set of tools that make it easier to manage state optimally.
Memoizing Selectors
Memoization is key to optimizing performance. Redux Toolkit’s createSelector
from the reselect
library helps you create memoized selectors. This reduces unnecessary recalculations and re-renders.
import { createSelector } from 'reselect'; const selectItems = state => state.items; const selectVisibleItems = createSelector( [selectItems], items => items.filter(item => item.visible) );
Using createSlice
for Efficient Reducers
Redux Toolkit’s createSlice
function simplifies creating reducers and actions. It automatically generates action creators and action types, reducing boilerplate.
import { createSlice } from '@reduxjs/toolkit'; const itemsSlice = createSlice({ name: 'items', initialState: [], reducers: { addItem: (state, action) => { state.push(action.payload); }, removeItem: (state, action) => { return state.filter(item => item.id !== action.payload.id); } } }); export const { addItem, removeItem } = itemsSlice.actions; export default itemsSlice.reducer;
Best Practices for Using Redux Toolkit in React
Redux Toolkit simplifies working with Redux in React applications by removing boilerplate code. To make the most of it, consider these best practices designed for developers aiming for efficient and maintainable code.
1. Use CreateSlice
The createSlice
function is a core part of Redux Toolkit. It helps in creating reducers and actions in a single step. This reduces boilerplate and keeps your code organized.
const counterSlice = createSlice({ name: 'counter', initialState: 0, reducers: { increment: state => state + 1, decrement: state => state - 1 } });
2. Configure Store with Middleware
Always use configureStore
when setting up your Redux store. This function automatically adds useful middleware like Redux Thunk and DevTools extension support.
const store = configureStore({ reducer: { counter: counterSlice.reducer } });
3. Embrace Immer
Immer is built into Redux Toolkit, allowing you to write simpler update logic in reducers. With Immer, you can directly mutate the state, making your code cleaner.
const todosSlice = createSlice({ name: 'todos', initialState: [], reducers: { addTodo: (state, action) => { state.push(action.payload); } } });
4. Keep State Structure Flat
A flat state structure improves performance and simplifies state updates. Avoid deeply nested state trees, which can lead to complex and error-prone updates.
Conclusion
Redux Toolkit simplifies state management in React applications. It streamlines the process, reducing boilerplate code and improving maintainability.
One of the significant advantages is its powerful abstraction. Developers can focus more on application logic and less on setup intricacies.
Moreover, Redux Toolkit integrates seamlessly with modern JavaScript features. It supports TypeScript, making it a robust choice for type-safe applications.
Additionally, it offers tools like createSlice and configureStore, which handle common tasks efficiently. This leads to cleaner and more readable code.
In practice, Redux Toolkit has proven to speed up development time. Teams can iterate faster, focusing on new features rather than debugging complex state logic.
Ultimately, adopting Redux Toolkit can lead to a more productive development experience. Its comprehensive ecosystem supports developers in building scalable and robust applications.
In the ever-evolving landscape of web development, Redux Toolkit stands out. It embodies best practices and encourages a structured approach to state management. Embracing it can transform how developers manage application state, making projects more efficient and enjoyable.
Previous
How to Start with Apollo Client in React: Quick Guide
Next