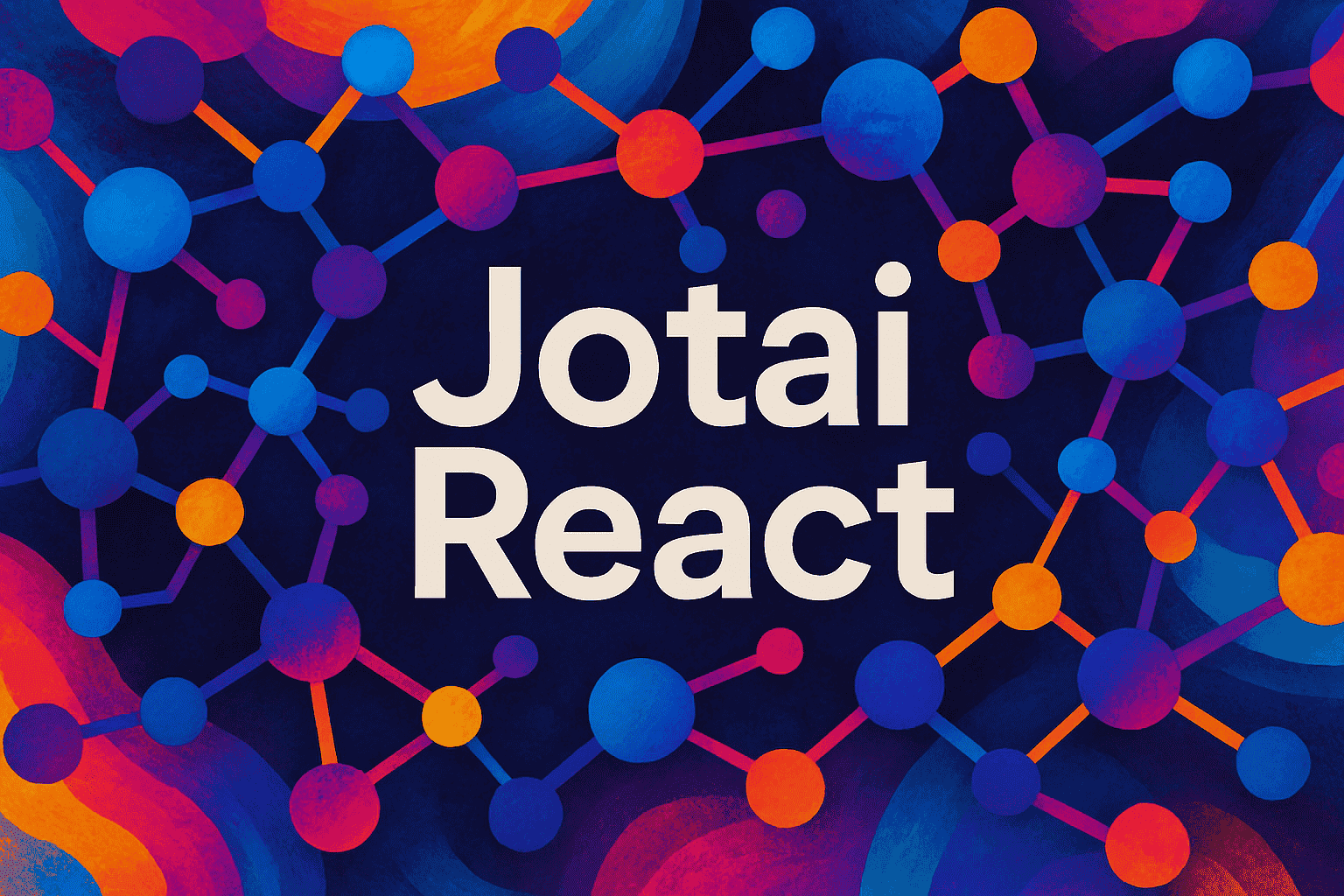
Introduction to Jotai for State Management in React
State management in React can be a daunting task, especially for complex applications. While Redux and Context API are popular choices, they can be overkill for smaller projects. Enter Jotai, a minimalistic state management library that provides a simpler and more flexible approach.
Jotai, which means “state” in Japanese, offers a refreshing take on managing state in React. It focuses on atoms, which represent a single piece of state. These atoms are easy to create and manage, making your state management more granular and efficient.
One of the standout features of Jotai is its atomic design. This allows developers to break down the state into smaller, more manageable pieces. You can then combine these atoms to create a cohesive state management solution. This approach encourages scalability and maintainability in your codebase.
Another advantage of Jotai is its integration with React’s concurrent mode. This means your application can handle more complex state changes without compromising performance. Jotai’s lean and flexible architecture makes it ideal for developers who want a lightweight solution without sacrificing power.
Why Choose Jotai?
- Minimalistic API: Jotai offers a simple and intuitive API that’s easy to learn and use.
- Atomic State Management: Break state into independent atoms for better scalability.
- Concurrent Mode Support: Seamlessly integrates with React’s latest features for optimal performance.
- Composable: Atoms can be combined to create more complex state structures.
Jotai is a powerful tool for React developers looking for a simple yet effective state management solution. Its focus on atoms and concurrent mode support make it a standout choice for both small and large projects.
Why Choose Jotai Over Other State Management Libraries?
When it comes to state management in React, developers often find themselves at crossroads deciding which library best fits their needs. Jotai, a relatively modern and lightweight solution, has been gaining traction for several compelling reasons.
First and foremost, Jotai is known for its simplicity and minimalism. It allows developers to manage global state without the complexity that comes with other libraries. Jotai’s API is intuitive, making it easier for developers to adopt and integrate into their projects.
- Pros:
- Simple and intuitive API
- Minimalistic and lightweight
- Flexible and highly composable
- First-class support for asynchronous atoms
- Cons:
- Less mature than Redux
- Smaller community support
Another standout feature of Jotai is its flexibility. It is highly composable, allowing developers to create custom atoms that suit their specific needs. Additionally, Jotai supports asynchronous operations seamlessly, making it suitable for modern web applications that rely on data fetching.
However, like any tool, Jotai has its drawbacks. Being newer to the ecosystem, it lacks the maturity and extensive community support that more established libraries like Redux offer. Yet, for many developers, the trade-off is worth it for the ease and efficiency Jotai provides.
Installing Jotai in Your React Project
To get started with Jotai, you first need to install it in your React project. It’s a simple process, and you’ll have it up and running in no time. Below are the steps to install Jotai using npm or yarn, the choice depends on your package manager preference.
Step 1: Install Jotai
First, open your terminal and navigate to your React project’s root directory. Once there, execute one of the following commands:
Using npm:
npm install jotai
Using yarn:
yarn add jotai
Step 2: Verify Installation
After installation, it’s crucial to verify that Jotai is correctly added to your project. Check your package.json
file to ensure that Jotai is listed under dependencies. Here’s a quick example:
{ "dependencies": { "jotai": "^1.5.0", ... } }
Step 3: Import Jotai in Your Code
With Jotai successfully installed, you can now import it into your React components. This enables you to start managing your application’s state efficiently. For example:
import { atom, useAtom } from 'jotai'; const countAtom = atom(0); function Counter() { const [count, setCount] = useAtom(countAtom); return (); }Count: {count}
Now you’re all set to start using Jotai for state management in your React project. Enjoy the simplicity and flexibility it offers!
Understanding Atoms and Their Role in Jotai
As developers, managing state in React applications can quickly become complex. Jotai, a minimalist state management library for React, simplifies this process with the concept of “atoms”. But what exactly are atoms, and why are they fundamental to Jotai?
In Jotai, an atom represents a piece of state. Think of it as a single unit or a building block that holds data. Atoms are created using the atom
function and can hold any JavaScript value, whether it’s a simple string, number, or a more complex object.
Creating an Atom
Creating an atom is straightforward. You define an atom with an initial value, and Jotai handles the rest. Here’s a simple example:
const countAtom = atom(0);
In this example, countAtom
is an atom with an initial value of 0. You can think of it as a React state variable, but without the need for a component to exist.
Using Atoms in Components
To use an atom in a component, Jotai provides a custom hook called useAtom
. This hook allows you to read and write the atom’s value:
import { useAtom } from 'jotai'; function Counter() { const [count, setCount] = useAtom(countAtom); return (); }Current Count: {count}
In this code snippet, we use useAtom
to read the count
value and update it with setCount
. The component re-renders automatically when the atom’s state changes.
The Power of Atoms
Atoms provide a scalable way to manage state. They’re independent, reusable, and can be composed to build complex state logic. By isolating state into these modular units, Jotai promotes a clean and maintainable codebase.
Understanding atoms is crucial for leveraging the full potential of Jotai in your React applications. They not only simplify state management but also enhance code readability and scalability.
Jotai is a minimalist state management library for React. It is built around the concept of atoms. Atoms are units of state; they hold the state and can be shared across components. To create an atom in Jotai, you’ll first need to import the library. Below is a simple example: Here, Once you’ve created an atom, the next step is to use it within your React components. This is done using the Current Count: {count} In this example, the Jotai offers a simple and flexible way to manage state in React applications. Its use of atoms allows for fine-grained control over state management, making it an attractive choice for developers. In the world of React, managing state efficiently can make or break your application. Jotai is a powerful tool for local state management that offers a fresh approach compared to other libraries. Jotai’s primary strength lies in its simplicity and minimalistic API. It allows developers to focus on the essentials without getting bogged down in complex configurations. This makes it an excellent choice for projects of any size. First, you need to install Jotai in your project. This can be achieved using npm or yarn: After installation, you can start creating atoms. Atoms are the fundamental units of state in Jotai. They hold your state and can be used or updated directly in your components. Let’s create a simple atom to manage a counter: Now, you can use this atom in a functional component: Count: {count} With this simple setup, you have a fully functional local state for your component. Jotai’s approach is both intuitive and flexible, making it a favorite among developers who prefer minimalistic solutions. Jotai stands out because of its straightforwardness and the ability to create complex state systems without unnecessary complexity. If you’re looking for a state management solution that is easy to understand and implement, Jotai is worth considering. When working with React applications, managing state across different components can become a challenge. Jotai provides a flexible and straightforward solution to this problem. It allows you to create global state management without complicating your codebase. Jotai is designed to be simple and lightweight. Unlike other state management libraries, it uses atoms to handle state. Each atom represents a piece of state that can be shared across components. First, add Jotai to your React project. Use the following command: Atoms are the core of Jotai’s state management. Here’s a simple example of creating an atom: To use atoms in your components, Jotai provides a convenient hook called Count: {count} Jotai offers powerful state management capabilities for React developers. Let’s dive into some advanced techniques that can elevate your application. Derived atoms allow you to create atoms based on existing ones, enabling complex state logic. This technique helps in keeping your state modular and manageable. Jotai supports asynchronous operations by allowing atoms to return promises. This is particularly useful for fetching data or other asynchronous tasks. Atom effects let you subscribe to changes or perform side effects. This feature can be used for logging or triggering additional actions. Create custom hooks to encapsulate and reuse logic across components. This pattern promotes code reusability and cleaner components. Jotai offers a fresh perspective on state management in React applications. Its minimalistic approach caters to developers who prefer simplicity without sacrificing power. By embracing Jotai, you benefit from its atomic model that promotes fine-grained control over state updates. One of the standout advantages is its seamless integration with the React ecosystem. Jotai allows you to manage state with minimal boilerplate code, making your development process more efficient. This efficiency can lead to faster project completion times, which is always a plus for developers working under tight deadlines. Moreover, Jotai’s performance optimizations ensure that your applications remain responsive. The atomic state management ensures that only components that need to re-render do so, improving the overall user experience. This can be particularly beneficial in larger applications with complex state dependencies. Another significant benefit is its flexibility. Jotai can be used in conjunction with other state management libraries, offering you a hybrid approach tailored to your project’s specific needs. This adaptability makes it a versatile choice for developers looking to enhance their existing React applications. In summary, Jotai’s intuitive API, performance benefits, and flexibility make it an attractive option for developers. By incorporating Jotai into your React projects, you can achieve a more streamlined and performant application, ultimately delivering a better experience to your users.Creating Atoms: A Step-by-Step Guide
import { atom } from 'jotai';
const countAtom = atom(0);
countAtom
is an atom that holds a numeric state initialized to zero. This atom can be used in any component within your React application.Utilizing Atoms in Components
useAtom
hook, which is also provided by Jotai.
import { useAtom } from 'jotai';
function Counter() {
const [count, setCount] = useAtom(countAtom);
return (
Counter
component uses the countAtom
to display and update a count value. Each time the button is clicked, the count is incremented.Benefits of Using Jotai
Using Jotai for Local State Management
Setting Up Jotai
npm install jotai
Creating and Using Atoms
import { atom, useAtom } from 'jotai';
const counterAtom = atom(0);
import React from 'react';
function Counter() {
const [count, setCount] = useAtom(counterAtom);
return (
Why Choose Jotai?
Integrating Jotai with Global State Management
What Makes Jotai Unique?
Setting Up Jotai
npm install jotai
Creating Atoms
import { atom } from 'jotai';
const countAtom = atom(0);
Using Atoms in Components
useAtom
. This hook allows you to read and write the state of an atom.import { useAtom } from 'jotai';
import { countAtom } from './atoms';
function Counter() {
const [count, setCount] = useAtom(countAtom);
return (
Benefits of Using Jotai
Advanced Techniques in Jotai
1. Derived Atoms
const baseAtom = atom(1);
const derivedAtom = atom((get) => get(baseAtom) * 2);
2. Asynchronous Atoms
const asyncAtom = atom(async () => {
const response = await fetch('/api/data');
return response.json();
});
3. Atom Effects
const countAtom = atom(0);
const countEffect = atom(
(get) => get(countAtom),
(get, set, newCount) => {
console.log('Count changed:', newCount);
set(countAtom, newCount);
}
);
4. Custom Hooks with Jotai
import { useAtom } from 'jotai';
function useCustomCounter() {
const [count, setCount] = useAtom(countAtom);
const increment = () => setCount(count + 1);
return { count, increment };
}
Conclusion: The Benefits of Using Jotai in Your React Projects
Previous
Step-by-Step Guide to Using Redux Toolkit in React
Next