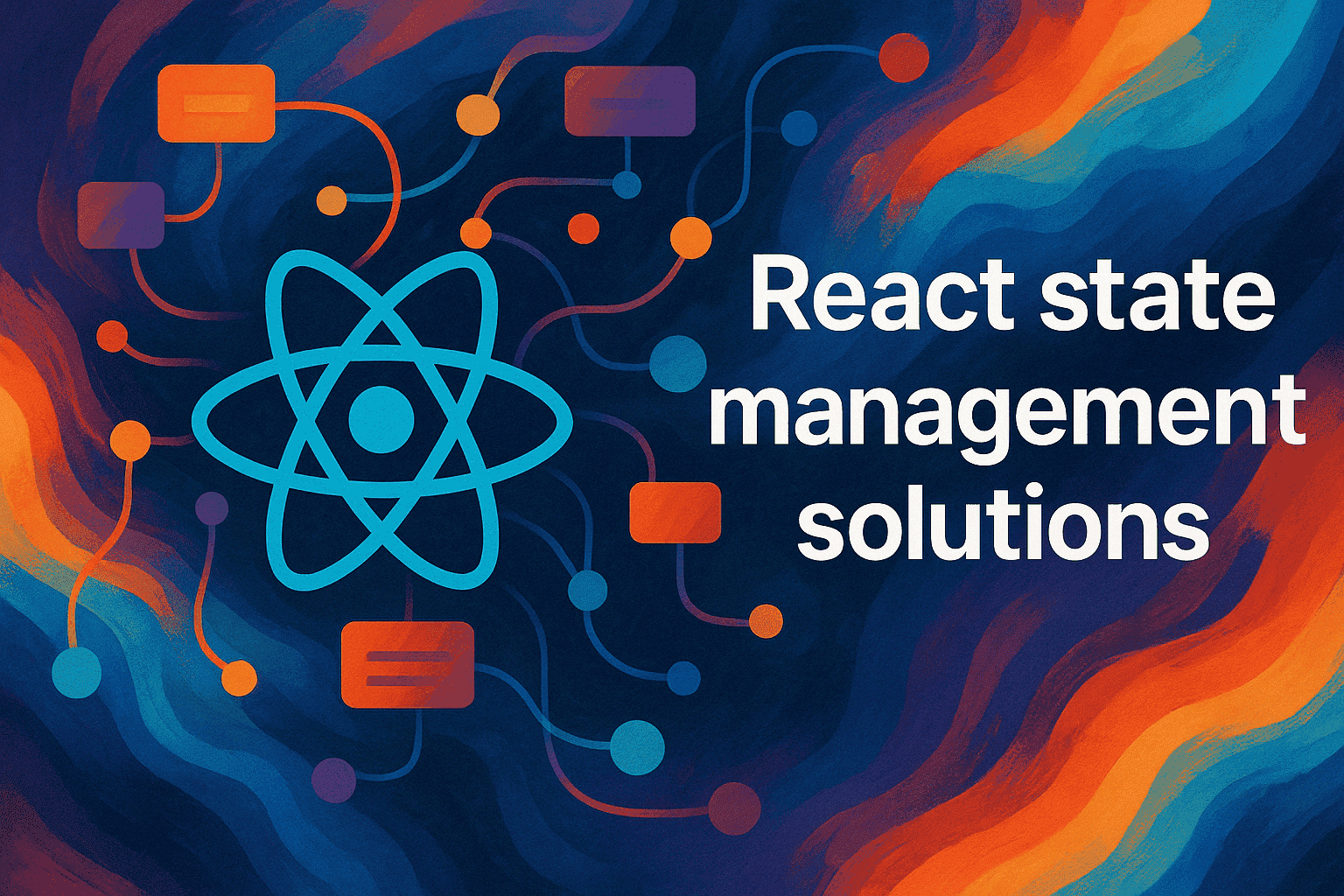
Understanding the Need for State Management
As React applications grow, managing state becomes crucial. State management helps you track and update the information your app relies on. It forms the backbone of your application’s data flow.
Without effective state management, your app can become unpredictable. Imagine a user interacting with a form, but the data doesn’t persist across components. This inconsistency can frustrate both developers and users.
State management provides a centralized location to manage changes. This centralization allows for smoother data updates, improving user experience. It also helps in debugging, as changes in state are easier to track.
There are several tools available for managing state in React. Each solution offers unique features and benefits. Choosing the right one depends on your project requirements and team preferences.
Why State Management Matters
Efficient state management leads to better performance. By minimizing unnecessary re-renders, your app runs faster. It also ensures data consistency across components, reducing the risk of errors.
Moreover, state management solutions often include debugging tools. These tools empower developers to trace changes and identify issues quickly. This capability speeds up the development process and enhances code quality.
Common Challenges Without State Management
- Data inconsistency across components
- Difficulty in tracking state changes
- Increased complexity with growing applications
To address these challenges, adopting a state management solution is essential. Whether you choose Redux, Context API, or another tool, understanding their differences is key.
React state management is crucial for building interactive applications. It determines how data flows in your app, ensuring components update correctly when data changes.
Understanding the basics is essential for any developer diving into React. Let’s explore the fundamental concepts:
- State: It represents the dynamic parts of your component. State changes trigger re-renders, keeping your UI in sync with data.
- useState Hook: This React hook allows you to add state to functional components. It returns a state variable and a function to update it.
- Props: While state is local to a component, props allow you to pass data from parent to child components. They facilitate component communication.
- Context API: Use this API when you need to share data across many components without prop drilling. It provides a way to pass data through the component tree.
- Reducer: For complex state logic, consider using a reducer. It’s a function that specifies how state updates based on actions.
Transitioning from basic to advanced state management solutions often involves libraries. These libraries help manage global state, offering more structure and scalability.
Choosing the right approach depends on your application’s complexity and your team’s familiarity with these concepts. As you gain experience, you’ll find the best fit for your projects.
Redux
In the vast landscape of React state management, Redux stands tall as a quintessential solution. It has not only become a staple for developers but also earned the title of an “industry standard.” With its robust architecture and predictability, Redux offers a streamlined approach to managing complex state logic in React applications.
Redux follows a unidirectional data flow, which simplifies debugging and makes testing more straightforward. This makes it a favorite choice among developers working on large-scale applications. Its global state management allows you to access and modify state from any component, providing flexibility and scalability.
Why Choose Redux?
Here are some reasons why Redux might be the right choice for your project:
- Predictable State Management
- Centralized Global State
- Rich Ecosystem and Community Support
- Middleware for Side Effects
Pros and Cons
- Pros:
- Highly Predictable State Management
- Strong Community and Ecosystem
- Cons:
- Can be Overkill for Small Projects
- Steep Learning Curve for Beginners
When selecting a state management solution, consider the complexity and scale of your application. Redux shines in projects where predictability and a centralized state are paramount.
Redux Toolkit
Redux Toolkit has become a game-changer in the realm of state management. It offers a streamlined approach, especially for developers who are keen to avoid the boilerplate of traditional Redux configurations.
Developers love Redux Toolkit for its simplicity and efficiency. It removes the complexity associated with setting up Redux from scratch. By leveraging features like createSlice and createAsyncThunk, Redux Toolkit makes managing state and asynchronous logic much more straightforward.
- Pros:
- Reduces boilerplate code significantly
- Improves code readability and structure
- Offers built-in support for common Redux patterns
- Cons
- Might feel abstracted for developers preferring full control
- Learning curve if accustomed to traditional Redux
If you’re considering Redux Toolkit, it’s essential to weigh its advantages against your project needs. It’s particularly beneficial for large-scale applications where managing complex state logic can quickly become overwhelming.
Core Features of Redux Toolkit
- CreateSlice for automatic action creators and reducers
- ConfigureStore for simplified store setup
- CreateAsyncThunk for handling async operations
Incorporating Redux Toolkit into your React projects can enhance performance and maintainability. It’s a compelling choice for developers seeking a modern, efficient state management solution.
Context API
When managing state in React applications, Context API offers a streamlined, native solution. Context API allows developers to share state across components without prop drilling. This approach is particularly useful in larger applications where passing props through multiple levels becomes cumbersome.
Context API is part of React’s core library. It enables developers to create global variables accessible throughout the app. Unlike other state management tools, Context API doesn’t require additional installations. It is built directly into React, making it a lightweight choice.
Using Context API involves creating a context object with React.createContext()
and wrapping your component tree with a context provider. Components can then consume the context using hooks like useContext
. This method simplifies access to shared state, reducing boilerplate code.
While Context API is great for smaller projects, it may not scale well in complex applications. Performance issues can arise if a large number of components consume the context. Frequent updates to the context can lead to unnecessary re-renders, impacting performance.
- Pros:
- Built-in React solution, no extra dependencies needed.
- Simplifies state management for smaller applications.
- Reduces prop drilling.
- Cons:
- May cause performance issues in large applications.
- Not suitable for managing deeply nested states.
Steps to Implement Context API
- Create a context using
React.createContext()
. - Wrap your component tree with a context provider.
- Access context using the
useContext
hook in components.
MobX
When it comes to state management in React, MobX stands out as a simpler alternative to other libraries. Its approach focuses on making state management intuitive and less cumbersome for developers, especially those who are just starting with complex applications.
MobX employs a reactive programming model, which automatically updates the UI when the state changes. This model reduces boilerplate code, making it easier to manage and understand. By using MobX, developers can focus more on building features rather than dealing with complex state logic.
Unlike Redux, which requires a more structured approach, MobX allows for greater flexibility. Developers can directly mutate the state, and MobX takes care of ensuring the UI stays in sync. This can be particularly beneficial in scenarios where rapid development and prototyping are needed.
- Pros:
- Less boilerplate code compared to Redux
- Automatically updates UI with state changes
- Flexible and easy to learn for beginners
- Cons:
- Can lead to less predictable state management
- Less suitable for large-scale applications
Key Features of MobX
- Reactive state management without explicit subscriptions
- Support for both functional and class components
- Seamless integration with React
- Small learning curve
In summary, MobX offers an approachable solution for state management, especially suitable for small to medium-sized projects. Its simplicity and automatic UI updates make it an attractive choice for developers seeking an efficient alternative to more complex libraries.
Recoil
Recoil is a state management library developed by Facebook to address the limitations of React’s built-in state management. It provides a set of tools for managing complex global state with ease, enabling developers to build highly responsive applications.
Recoil’s architecture is built around atoms and selectors. Atoms represent shared state across components, while selectors are pure functions that compute derived state. This design allows Recoil to efficiently manage state updates and optimize rendering performance.
One of the standout features of Recoil is its ability to provide asynchronous state management without the need for additional libraries. This is particularly useful for applications that rely on data fetching or other asynchronous operations.
Recoil is gaining popularity because of its flexibility and seamless integration with React’s hooks. Its minimalistic API and focus on performance make it a compelling choice for developers looking for a modern state management solution.
- Pros:
- Efficient state updates and rendering
- Seamless asynchronous state management
- Minimalistic API design
- Cons
- Still relatively new and evolving
- Limited community resources compared to Redux
Key Features
- Atoms and selectors for state representation
- Support for asynchronous state management
- Integration with React hooks
- Focus on performance and minimalism
Zustand
When it comes to state management in React, Zustand shines with its simplicity and flexibility. As developers, we often seek tools that are easy to integrate and offer minimal overhead. Zustand fits this description perfectly.
Unlike more complex libraries, Zustand provides a lightweight solution that doesn’t burden your application with unnecessary complexity. It leverages a small API, enabling you to focus on building features rather than managing state intricacies. Zustand’s simplicity doesn’t come at the cost of functionality. It is powerful enough to manage state efficiently, making it an attractive option for both small and large projects.
Furthermore, Zustand’s flexibility allows developers to use it in diverse scenarios. Whether you’re creating a simple app or a complex system, Zustand adapts to your needs. It supports both synchronous and asynchronous state updates, giving you control over your application’s behavior.
- Pros:
- Lightweight and easy to integrate
- Minimal API, reducing learning curve
- Supports both synchronous and asynchronous updates
- Cons:
- May not provide advanced features out-of-the-box
For developers seeking a state management solution that is both efficient and straightforward, Zustand is worth considering. It empowers you to manage state with ease and focus on what truly matters: building exceptional applications.
Features of Zustand
- Minimalistic API for easy learning and quick setup
- Supports server-side rendering
- Works seamlessly with React’s concurrent mode
- Provides a simple way to manage global state
XState
When diving into the world of React state management, XState emerges as a compelling solution. It offers a unique approach by leveraging finite state machines and statecharts, providing developers with a robust model for managing complex states.
Why Consider XState?
XState brings clarity to state management by defining states explicitly and managing transitions between them. This makes it easier to visualize and debug state changes, which is particularly beneficial in large applications.
Key Features of XState
- Finite state machines and hierarchical statecharts
- Visualize state transitions using tools like XState Visualizer
- Integration with React through the xstate/react library
- Supports parallel states for complex scenarios
Pros and Cons
- Pros:
- Facilitates clear and predictable state transitions
- Enhances debugging with visual statechart representation
- Encourages a well-structured state management approach
- Cons
- Learning curve for developers unfamiliar with state machines
- Potentially overkill for simple applications
When to Use XState
If your application requires managing complex states and transitions, XState can be a game-changer. It is particularly useful in scenarios where state predictability and debugging are crucial.
Apollo Client
Apollo Client stands out as a powerful library for managing state in React applications. It excels particularly in environments where GraphQL is the primary data fetching mechanism. Developers appreciate its seamless integration with React, providing a robust solution for handling server state.
One of the best features of Apollo Client is its capability to handle both local and remote data seamlessly. This allows developers to leverage GraphQL to its fullest potential, making data management intuitive and efficient.
- Pros:
- Integrates well with GraphQL APIs.
- Offers built-in support for caching and state management.
- Provides excellent developer tools for debugging.
- Cons
- Might be overkill for small applications.
- Requires understanding of GraphQL.
Key Features
- Automatic caching of responses to minimize network requests.
- Client-side state management.
- Real-time updates with subscriptions.
Furthermore, Apollo Client supports optimistic UI updates, which enhances user experience by providing immediate feedback while waiting for server responses. Transitioning from conventional REST APIs to GraphQL with Apollo Client can significantly improve your application’s efficiency and responsiveness.
Jotai
In the bustling world of React state management, Jotai stands out with its minimalist design and scalability. As developers, we often seek tools that are both lightweight and powerful. Jotai answers this call by offering a primitive approach that simplifies state management without sacrificing functionality.
Jotai, which means “state” in Japanese, is built on the idea of atoms. These atoms represent the smallest units of state, allowing developers to craft finely-tuned applications. By focusing on these primitive elements, Jotai provides a robust foundation for scalable state management.
Moreover, Jotai’s API is straightforward, making it an excellent choice for developers at all skill levels. Whether you’re building a small widget or a complex application, Jotai’s simplicity and scalability make it an attractive option.
- Pros:
- Lightweight and minimalistic
- Scales well with application complexity
- Easy to learn and implement
- Cons:
- May require more setup for complex state logic
Key Features
- Atom-based state management
- Simple API with powerful capabilities
- Flexibility in handling complex state logic
Conclusion
In choosing the right React state management solution, consider your application’s complexity and specific requirements. Each tool offers unique advantages tailored to different scenarios. Whether it’s Redux for large-scale applications or Context API for simpler ones, your choice should align with the project’s goals.
Moreover, evaluate factors like scalability, ease of integration, and community support. A well-supported library can save time and streamline development. Additionally, think about your team’s familiarity with the tool and its learning curve. The right choice can significantly impact productivity and code maintainability.
Lastly, keep future growth in mind. Your application might evolve, and the state management solution should accommodate changes without major overhauls. By carefully weighing these considerations, you can choose the most suitable state management approach for your React application.
Previous
How to Use Jotai for State Management in React
Next