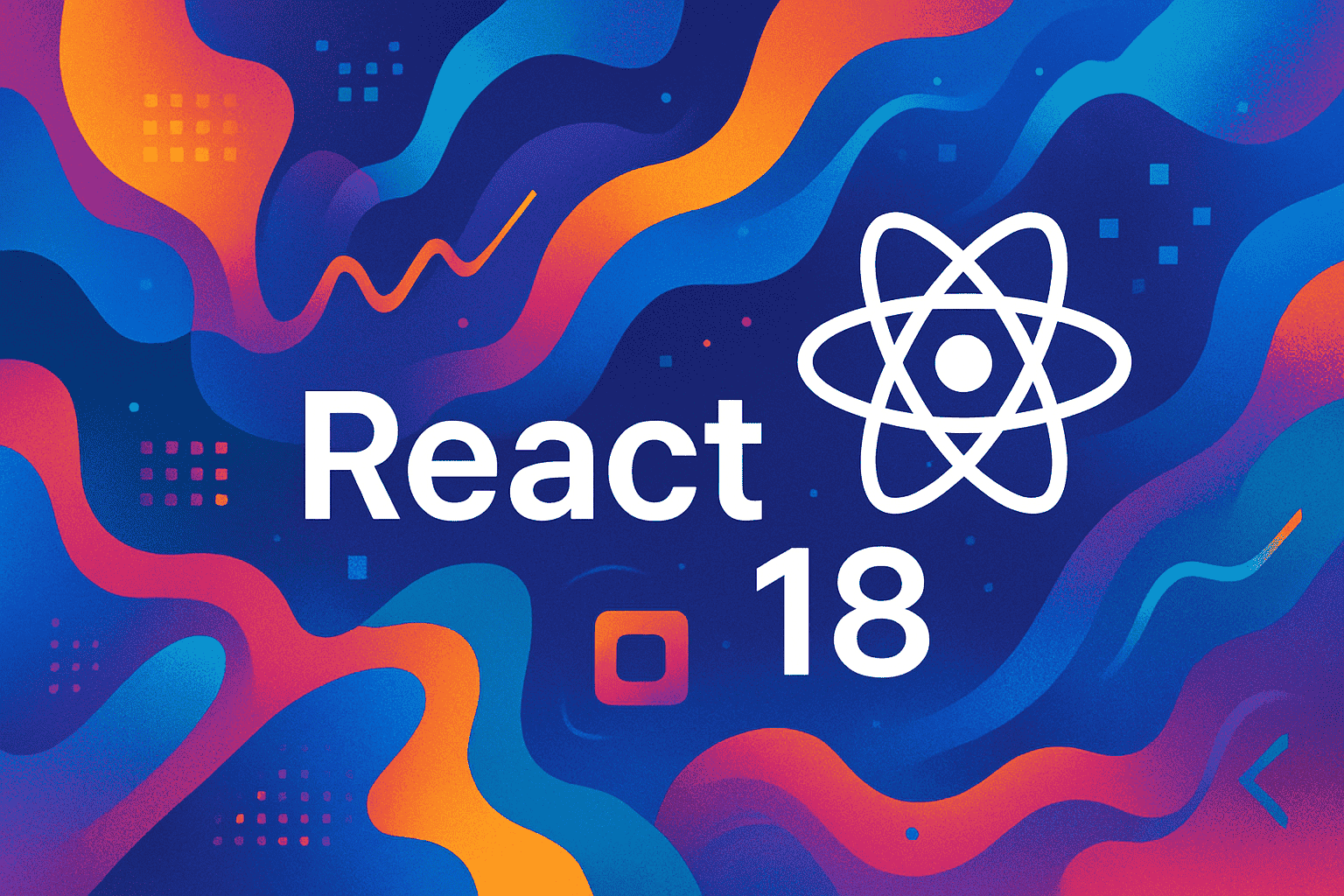
Introduction in React 18
React 18 introduces exciting features that enhance the capabilities of this popular JavaScript library. For developers, understanding these features is crucial to leverage their full potential. The spotlight shines on concurrent rendering, a game-changer for building smoother UIs.
Concurrent rendering allows React to prepare multiple versions of the UI simultaneously. This means your applications will be more responsive, avoiding the dreaded frozen screen. Developers can now prioritize tasks, improving performance and user experience. With React 18, it’s easier to build apps that feel fast and fluid.
Another significant addition is automatic batching. Previously, updates inside event handlers were batched. Now, React 18 extends this to all updates, reducing unnecessary re-renders. This optimization helps maintain efficient performance, especially in complex applications.
React 18 also introduces the startTransition API. This feature lets developers mark updates as non-urgent, allowing the UI to remain interactive. By deferring non-critical updates, the user experience stays seamless, even during heavy computations.
Moreover, the new streaming server-side rendering (SSR) enhances the delivery of content. It speeds up the loading process, as HTML is sent in chunks. Users see content faster, improving the perception of performance. This is invaluable for SEO, as search engines favor faster-loading sites.
React 18’s enhancements are not just about speed and performance. They empower developers to create more robust and scalable applications. By embracing these changes, you can deliver enhanced user experiences, making your apps stand out. Dive into React 18’s documentation to explore these features in detail, and start integrating them into your projects today.
Concurrent Rendering in React 18
React 18 introduces Concurrent Rendering, a game-changer for developers aiming to create smooth, responsive UIs. This feature allows React to prepare multiple versions of the UI simultaneously, enhancing user experience.
Concurrent Rendering enables React to work on different tasks concurrently. This means your application remains interactive while rendering complex components in the background. It’s like having multiple hands working on a project, ensuring everything gets done efficiently.
Why Concurrent Rendering Matters
- Improves application responsiveness
- Reduces blocking times
- Enhances user experience during heavy computations
In traditional rendering, React had to finish one task before starting another. However, with Concurrent Rendering, React can interrupt a task, prioritize urgent updates, and then continue with the previous task without losing progress.
How to Utilize Concurrent Rendering
To leverage Concurrent Rendering, you can use ReactDOM.createRoot
instead of ReactDOM.render
. This enables concurrent features automatically.
const root = ReactDOM.createRoot(document.getElementById('root')); root.render();
Concurrent Rendering optimizes the way React updates the UI. It helps in scenarios where multiple updates occur simultaneously, like in collaborative applications or real-time dashboards.
Code Splitting with Suspense
React 18 enhances the Suspense
component, allowing you to fetch data in a non-blocking manner. This works hand-in-hand with Concurrent Rendering, making data fetching seamless.
Loading...}>
By using Concurrent Rendering effectively, you can build applications that feel faster, respond better, and provide a more engaging user experience.
Automatic Batching Enhancements in React 18
React 18 has introduced several enhancements, one of the most notable being automatic batching. This feature allows React to group multiple state updates into a single render, leading to improved performance and a smoother user experience.
Understanding Automatic Batching
Previously, React only batched updates within event handlers. With the introduction of React 18, automatic batching now extends to all updates, including those inside async
functions, setTimeout
, and other non-event callbacks.
Why It Matters
Automatic batching reduces unnecessary renders, which can significantly enhance performance. By batching updates together, React minimizes the work needed to update the DOM, leading to faster applications.
Example of Automatic Batching
Let’s look at a simple example to see how automatic batching works in practice:
import { useState } from 'react'; function MyComponent() { const [count, setCount] = useState(0); const [text, setText] = useState(''); const handleClick = async () => { setCount(count + 1); setText('Updated'); console.log('State updated'); }; return (); }Count: {count}
Text: {text}
In the example above, even though setCount
and setText
are called separately, they are batched into a single render update.
Leveraging Automatic Batching
To take full advantage of automatic batching, ensure that your asynchronous logic leverages the new React 18 features. This will help you maintain an optimal performance profile in your applications.
Transitions and Their Importance
Transitions in React 18 bring a significant shift in how developers handle UI updates. They allow for smoother, non-blocking updates, enhancing user experience. Understanding transitions is crucial to leverage the full potential of React 18.
Why Transitions Matter
In applications, user interactions often trigger multiple state updates. Traditionally, these updates could result in UI jankiness. However, with transitions, developers can prioritize critical updates, ensuring that the UI remains responsive.
Implementing Transitions
To use transitions, React 18 introduces the startTransition
API. This API helps in marking certain updates as non-urgent. Consequently, it allows React to focus on essential updates first.
Example of startTransition
import { useState, startTransition } from 'react'; function MyComponent() { const [state, setState] = useState(0); const handleClick = () => { startTransition(() => { setState(prevState => prevState + 1); }); }; return ; }
In the above example, the startTransition
function ensures that the state update does not block more critical UI updates. Therefore, users experience a seamless interface.
Benefits of Using Transitions
- Improved user experience with smoother UI interactions.
- Better performance by prioritizing crucial updates.
- Enhanced control over update priorities.
By embracing transitions, developers can create highly responsive applications. As a result, users enjoy a more fluid experience, even when complex state changes occur. With React 18, transitions become an essential tool in every developer’s toolkit.
Suspense for Data Fetching in React 18
React 18 introduces an exciting feature called Suspense for Data Fetching. This feature simplifies the process of loading data in your components, making your applications faster and more efficient.
Previously, handling asynchronous data involved intricate state management and error handling. With Suspense, you can now manage these aspects more gracefully. It allows your components to “wait” for the needed data before rendering.
How Does It Work?
Suspense works by wrapping your component tree with the Suspense
component. This lets React know to pause rendering until the data is ready.
import React, { Suspense } from 'react'; const DataComponent = React.lazy(() => import('./DataComponent')); function App() { return (Loading...}> ); }
Benefits of Using Suspense
- Improved User Experience: Users see loading indicators instead of blank screens.
- Cleaner Code: Simplifies data-fetching logic in your components.
- Optimized Rendering: React manages the loading state, reducing unnecessary re-renders.
Practical Example
Consider a scenario where you fetch user data from an API. With Suspense, you can streamline this process:
import React, { Suspense } from 'react'; import fetchUserData from './fetchUserData'; const UserComponent = React.lazy(() => import('./UserComponent')); function App() { const userData = fetchUserData(); return (Loading user data...}> ); }
In this example, the UserComponent
waits for the user data to load before rendering, enhancing the user experience.
New Hooks: StartTransition and UseDeferredValue
React 18 introduces two new hooks: startTransition
and useDeferredValue
. These hooks enhance your app’s UX by managing the rendering process more efficiently. They prioritize updates, making your app feel faster and more responsive.
StartTransition Hook
The startTransition
hook is perfect for scheduling non-urgent updates. It allows you to define transitions, giving priority to urgent updates like user input. This ensures a smooth UI experience.
Imagine a search bar that updates results on each keystroke. Using startTransition
, you can prioritize the typing experience over the rendering of search results.
import { startTransition, useState } from 'react'; function SearchComponent() { const [query, setQuery] = useState(''); const [results, setResults] = useState([]); const handleChange = (e) => { const value = e.target.value; setQuery(value); startTransition(() => { setResults(fetchResults(value)); }); }; return ( ); }
UseDeferredValue Hook
The useDeferredValue
hook helps in deferring a value’s update. It’s similar to startTransition
, but focuses on the value itself. This is handy for optimizing heavy computations or rendering tasks.
Consider a component displaying a large dataset. With useDeferredValue
, you can defer the rendering of this dataset, ensuring the UI remains responsive to user actions.
import { useDeferredValue, useState } from 'react'; function LargeDatasetComponent({ data }) { const deferredData = useDeferredValue(data); return ({deferredData.map(item => (); }{item.name}))}
The React 18 Upgrade Path
React 18 introduces exciting new features, but upgrading requires careful planning. Developers need to understand the changes to benefit fully from the improvements.
Key Considerations for Upgrading
Upgrading to React 18 involves several steps. Begin by ensuring that all dependencies are compatible. It’s crucial to address any warnings that may appear during the upgrade process.
Concurrent Features
React 18 introduces concurrent features, improving performance. These features enable components to render with non-blocking operations. For example, you can use the new startTransition
API:
import { startTransition } from 'react'; startTransition(() => { setState(newState); });
Automatic Batching
Another enhancement is automatic batching. React now batches state updates, reducing unnecessary renders. This feature works seamlessly with events, promises, and other asynchronous operations.
Testing and Validation
Before migrating a production app, test thoroughly in a staging environment. Utilize tools like Jest and React Testing Library to ensure components behave as expected.
Community and Resources
Leverage community resources to ease the transition. The React documentation provides extensive guidance. Additionally, forums and discussion groups offer invaluable insights and tips.
Upgrade Checklist
- Review all dependencies for compatibility.
- Test new concurrent features in a controlled environment.
- Utilize React’s documentation for guidance.
- Engage with the community for shared experiences.
React 18 Impacts on Server-Side Rendering
React 18 brings significant improvements to server-side rendering (SSR), making it more efficient and flexible for developers. These enhancements are designed to improve performance and offer new capabilities that enhance the rendering process.
Streaming Server-Side Rendering
One of the most notable changes is the introduction of streaming server-side rendering. This allows components to be sent to the client as they are generated, rather than waiting for the entire page to be ready. This can significantly reduce the time to first byte (TTFB) and improve the perceived performance of your application.
const ReactDOMServer = require('react-dom/server'); const element = <App />; const stream = ReactDOMServer.renderToPipeableStream(element, { onCompleteShell() { // Send the initial HTML response to the client stream.pipe(response); }, });
Concurrent Features
React 18 introduces concurrent rendering features that allow for more flexible data fetching strategies. This is particularly useful for SSR as it enables developers to prioritize critical content and defer non-critical elements. The integration with Suspense and concurrent features can optimize the loading sequence.
Automatic Batching
Automatic batching in React 18 allows multiple state updates to be batched together into a single render, reducing unnecessary re-renders. This is particularly beneficial in SSR, where performance is critical and every millisecond counts.
Enhanced Hydration
React 18 improves the hydration process, making it more resilient and performant. The new selective hydration feature allows React to prioritize rendering parts of the application that are visible to the user, improving load times and user experience.
In conclusion, React 18 offers developers a suite of new tools and optimizations for server-side rendering. These improvements not only enhance performance but also provide more control over the rendering process, allowing for richer and more interactive applications.
React 18 and Its Impact on SEO
React 18 introduces several enhancements that can significantly impact SEO. One of the most notable features is concurrent rendering. This allows React to prepare multiple versions of the UI simultaneously. Consequently, it improves page load times and interactivity, which are critical for SEO.
Google’s algorithm favors faster websites. The new concurrent features in React 18 can help reduce Time to Interactive (TTI) and First Input Delay (FID). These metrics are crucial for Core Web Vitals, a key component of Google’s ranking factors.
- Concurrent Rendering
- Automatic Batching
- New Suspense Features
Furthermore, React 18’s automatic batching feature can optimize state updates. It minimizes unnecessary re-renders, helping to enhance performance. This is important because Google indexes content more effectively when it’s fast and efficient.
With React 18, developers can also leverage the new Suspense features. These allow for better server-side rendering (SSR) and hydration. Improved SSR means more HTML is available to crawlers right away, enhancing SEO.
Here’s a simple example of how to implement automatic batching in React 18:
// Automatic Batching Example import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const handleClick = () => { setCount(c => c + 1); setCount(c => c + 1); }; return ; }
In this example, React 18 batches the state updates, reducing unnecessary re-renders. This efficiency can lead to faster page loads, which is beneficial for SEO.
By understanding and utilizing these features, developers can improve both user experience and search engine rankings. React 18 makes it easier to build SEO-friendly applications, ensuring content reaches the right audience.
Best Practices for Utilizing React 18 Features
React 18 comes with a suite of new features aimed at improving performance and developer experience. To make the most of these updates, developers should focus on a few key practices.
Concurrent Rendering
Concurrent rendering is one of the most significant updates in React 18. It allows React to prepare multiple versions of the UI at the same time. This results in smoother transitions and enhanced user experience.
To enable concurrent rendering, use the createRoot
method instead of ReactDOM.render
. Here’s a quick example:
const root = ReactDOM.createRoot(document.getElementById('root')); root.render();
Automatic Batching
Another improvement in React 18 is automatic batching. This feature reduces unnecessary renders by batching multiple state updates together. This can significantly boost app performance.
Consider the following example, where multiple state updates are batched together:
function handleClick() { setCount(c => c + 1); setFlag(f => !f); }
Suspense for Data Fetching
Suspense is now more robust and can be used for managing asynchronous data fetching. This allows developers to declaratively handle loading states within components.
Here’s a simple illustration of using Suspense with a data-fetching component:
Loading...}>
Transition API
The new Transition API helps in distinguishing between urgent and non-urgent updates. This way, React prioritizes rendering tasks effectively.
To use the Transition API, wrap non-urgent updates within the startTransition
method:
startTransition(() => { setSearchQuery(input); });
By following these best practices, developers can fully leverage React 18’s capabilities, leading to more responsive and efficient applications.
Previous
Top React State Management Solutions: How to Choose
Next