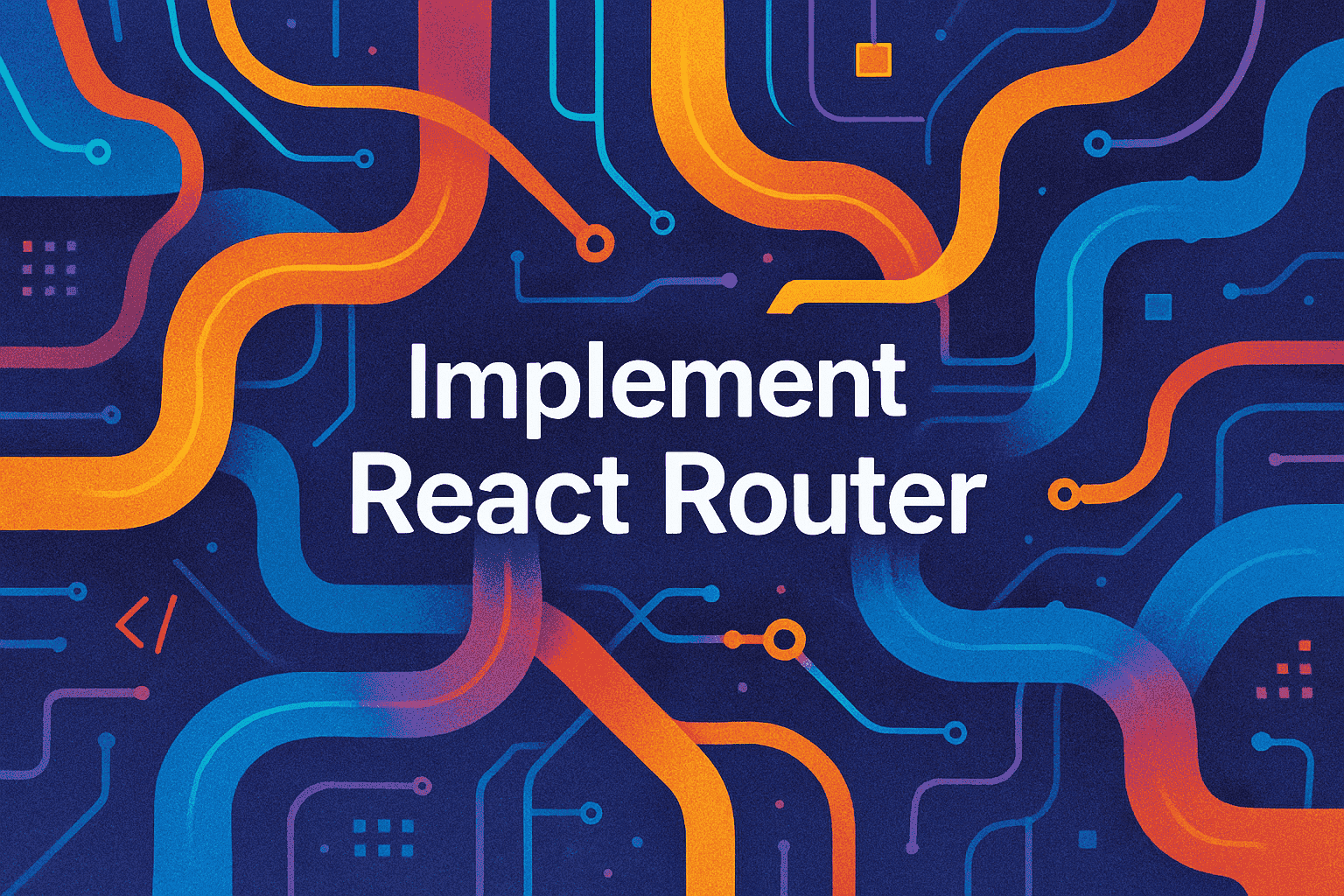
Fast Answers
Introduction in React Router
As developers, navigating the world of web development often involves creating seamless navigation experiences. React Router, a powerful library in the React ecosystem, is key to implementing dynamic routing in your applications.
React Router allows you to manage and navigate between different components and views in a React application. It enhances user experience by making navigation intuitive and straightforward. In dynamic routing, the route paths are created dynamically based on the data or user input.
To start with React Router, install it using npm or yarn. Once installed, you can import essential components like BrowserRouter, Route, and Switch. These components are the building blocks of your routing system.
Dynamic routing adapts to user input or data changes in real-time. This makes your application more interactive and responsive. For instance, you can use parameters in your route paths, such as /user/:id, to render different user profiles dynamically.
Using route parameters and query strings, you can access dynamic data within your components. This enables you to render content based on the current route parameters, providing a personalized experience for users.
Implementing dynamic routing in React requires understanding the structure and flow of your application. Plan your routes carefully, and ensure that you utilize nested routes for complex structures. This keeps your code modular and maintainable.
React Router also supports route guards and redirects. These features help in managing access control and redirecting users based on their actions or roles. Utilizing these features can enhance the security and usability of your application.
Incorporate React Router’s dynamic routing into your projects to elevate user experience. It empowers you to build flexible and scalable applications, ensuring users have a smooth navigation journey.
Installing React Router
React Router is essential for creating single-page applications with dynamic routing. Installing it is straightforward and can be done in a few steps.
Step-by-Step Guide
-
First, ensure you have Node.js and npm installed on your machine. You can check this by running:
node -v
and
npm -v
-
Navigate to your project directory in the terminal.
-
Use npm to install React Router by executing:
npm install react-router-dom
This command will add React Router to your project’s dependencies.
Verify Installation
After installation, verify that React Router is correctly added by checking your package.json
file. You should see it listed under dependencies.
Example: Basic Setup
To get started with React Router, import BrowserRouter
into your main App.js
file:
import { BrowserRouter as Router } from 'react-router-dom'; function App() { return (); } Your app components go here
This setup wraps your application components, enabling dynamic routing.
Implementing Dynamic Routing with React Router
Dynamic routing in React enables developers to create applications that can adapt based on user interactions. React Router, a popular library, offers a robust way to handle dynamic routes.
Understanding Dynamic Routing
Dynamic routes are paths in your application that change based on variables. These variables often represent different data points, such as user IDs or product names.
Creating a Basic Route
To start, set up a basic route using BrowserRouter
and Route
components:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; function App() { return (); }
Implementing Dynamic Routing
Dynamic routes are created using route parameters. Here’s an example of a dynamic route:
In this example, :id
is a dynamic segment. It allows you to load a user profile based on the ID passed in the URL.
Accessing Route Parameters
To access these parameters within a component, use the useParams
hook:
import { useParams } from 'react-router-dom'; function UserProfile() { const { id } = useParams(); // Fetch and display user data based on the ID }
Benefits of Dynamic Routing
- Improves user experience by providing unique URLs for different data.
- Enables easier navigation and bookmarking.
- Facilitates SEO optimization by creating meaningful
Using Route Parameters in React Router
In the realm of modern web development, dynamic routing is essential. React Router provides an elegant way to handle this through route parameters. These parameters allow developers to capture and use values from the URL, making the web application more interactive and robust.
What are Route Parameters?
Route parameters are dynamic segments of a URL. They are placeholders in your path definitions that can be replaced with actual data. This is particularly useful when you want to pass data to a component rendered by a route.
Implementing Route Parameters in React Router
To use route parameters, define them in your route paths. Use a colon (:) followed by a parameter name. Let’s explore how to implement this in a React application.
Defining Routes with Parameters
Consider an example where you want to display user details based on a unique ID. Define your route with a parameter like this:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; import UserProfile from './UserProfile'; function App() { return (); }
Accessing Route Parameters
Within your `UserProfile` component, use the `useParams` hook from `react-router-dom` to access the parameter:
import { useParams } from 'react-router-dom'; function UserProfile() { const { id } = useParams(); return (User ID: {id}); }
In this example, when a user navigates to `/user/123`, the `UserProfile` component displays “User ID: 123”. This method is efficient and maintainable, enabling dynamic content rendering based on the URL.
Advantages of Route Parameters
- Allows dynamic content rendering.
- Facilitates building RESTful applications.
- Enhances user experience with personalized data.
Nested Routes and Their Importance
In the realm of dynamic routing with React Router, nested routes play a crucial role. They allow developers to define routes within other routes. This capability is essential for building complex, yet organized, application structures.
Imagine a scenario where you have a user dashboard. Within this dashboard, there might be different sections like profile settings, notifications, and account history. Using nested routes, each section can have its own route and component, nested under the main dashboard route.
Advantages of Nested Routes
- Organizational Clarity: Nested routes help you maintain a clear structure, making your application easy to navigate and understand.
- Code Reusability: Components can be reused across different parts of the application, reducing redundancy.
- Enhanced User Experience: Users can navigate to specific sections without reloading the entire page.
Implementing Nested Routes
To implement nested routes in React Router, you need to define routes within another route’s component. Here’s a simple example:
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom'; function App() { return (); } }> } /> } /> } />
In this example, the Dashboard
component contains nested routes for Profile
, Notifications
, and Account
. This hierarchy allows users to access any section directly.
Best Practices
- Keep your route paths meaningful and intuitive.
- Ensure components are modular and reusable.
- Test nested routes to ensure they work seamlessly across different devices.
Navigating programmatically in a React application is a fundamental skill for developers. React Router provides a powerful API to manage routing, allowing you to navigate without relying solely on user interaction.
Programmatic navigation enables developers to change the route in response to specific events, such as form submissions or successful data fetching. This approach enhances user experience by providing seamless navigation.
The useNavigate
hook is a key component in achieving programmatic navigation. It returns a function that you can call to navigate to different routes.
import { useNavigate } from 'react-router-dom'; function SubmitButton() { const navigate = useNavigate(); const handleSubmit = () => { // Perform some logic navigate('/success'); }; return ( ); }
- Improves user experience by automating transitions.
- Handles routing logic in response to application state changes.
- Allows conditional navigation based on user actions or data.
Best Practices
While using programmatic navigation, make sure to handle edge cases. Consider user permissions and application state before navigating to a new route.
Best Practices for Dynamic Routing
Dynamic routing in React allows you to create flexible and efficient applications. Implementing React Router for dynamic routing can be challenging, but following best practices can simplify the process. Here’s how you can make the most of React Router for dynamic routing.
Keep Routes Organized
Organizing your routes file is crucial. It helps in maintaining your code and makes it easier to navigate as the application grows.
Leverage Route Parameters
Utilize route parameters to pass dynamic data through the URL. This approach is essential for creating personalized user experiences.
{ path: '/user/:id', component: UserProfile }
Use Switch for Exclusive Routes
The Switch
component ensures that only one route is rendered at a time. This can prevent rendering conflicts and improve performance.
Implement Lazy Loading
Lazy loading routes can drastically enhance the user experience by reducing initial load time. Import routes as needed with React’s lazy
function.
const UserProfile = React.lazy(() => import('./UserProfile'));
Consider Nested Routes
Nesting routes within other routes can create a more intuitive and organized structure. It allows you to group related views together.
Handle Not Found Routes
Implement a catch-all route for handling 404 errors. This ensures users are informed when they navigate to a non-existent page.
Testing and Debugging
Regularly test your routes to ensure they work as expected. Use developer tools for debugging and to track route changes.
Troubleshooting Common Issues in React Router
Implementing React Router can be a game-changer for dynamic routing in your application. However, developers often face common issues during integration. Understanding these can save time and enhance your app’s performance.
1. Missing Route Parameters
Sometimes, route parameters may not pass correctly, causing unexpected behavior. Ensure your route path matches the component’s expected parameters.
{path: '/user/:id', component: UserProfile}
Check if the component receives the ‘id’ parameter as expected.
2. Incorrect Route Order
Order matters in React Router. Specific routes should come before more generic ones. Otherwise, the router might match the wrong route.
Place the home route at the bottom to avoid conflicts.
3. Non-Rendering Components
If a component isn’t rendering, check for typos in the path or component name. Verify that the component is imported correctly.
import About from './components/About';
Ensure the import path matches the file structure.
4. Browser Compatibility Issues
Some features may not work in all browsers. Ensure your app is tested across different browsers for consistent performance.
5. Performance Bottlenecks
Large applications can suffer from slow routing. Use React’s lazy loading and split code into chunks to enhance performance.
const Home = React.lazy(() => import('./components/Home'));
Utilizing lazy loading can significantly improve load times.
Conclusion
Implementing React Router for dynamic routing is a game-changer in web development. It allows developers to create seamless, single-page applications with ease. By understanding and utilizing React Router, you can enhance the user experience and optimize your web application for performance.
React Router’s dynamic routing capabilities make it possible to render components based on the URL, providing a more intuitive navigation system. This flexibility is essential for building scalable applications that can grow with user needs.
Moreover, React Router integrates smoothly with other libraries and tools, making it a versatile choice for developers. Its dynamic nature ensures that your application is not only efficient but also adaptable to future changes and updates.
In summary, by mastering React Router, you empower yourself to build robust, dynamic applications. Embrace this tool, and you’ll find your development process becoming more streamlined and efficient.
Previous
React 18: What’s New & How to Utilize It
Next